Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial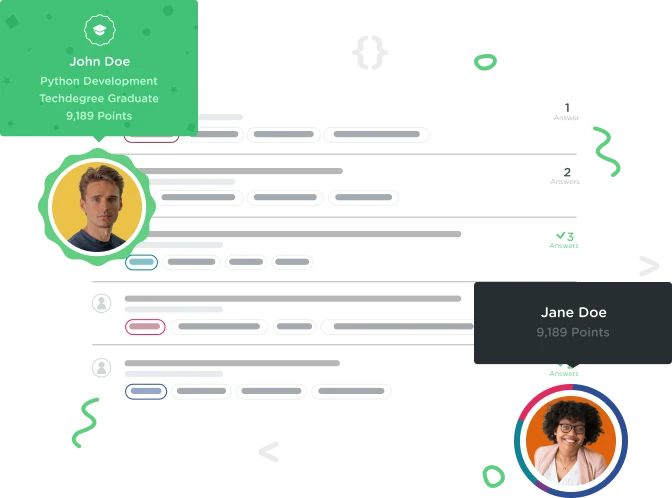

johnsdoe
Courses Plus Student 4,920 Points"Fun Fact has stopped" or "Fun Facts keeps stopping", While trying to RUN the app on Available Virtual Devices.
Can't seem to find a problem inside the code. Also can't RUN on Available Virtual Devices.
3 Answers
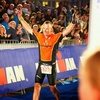
Steve Hunter
57,712 PointsHi Shamir,
Yes, the issue is highlighted well in your error. Your error is here:
Caused by: java.lang.NullPointerException: Attempt to invoke virtual method 'void android.widget.Button.setOnClickListener(android.view.View$OnClickListener)' on a null object reference
at com.buildultra.funfacts.FunFacts.onCreate(FunFacts.java:52)
This is a NullPointerException
which means one of your variables that you've tried to use has nothing assigned to it; it is pointing at null, or nothing.
The error is being thrown as you code execution reaches this line; but your error isn't at this line:
mShowFactButton.setOnClickListener(listener);
The problem is that nothing has been assigned into mShowFactButton
- it is pointing at nothing. That's beacuse you've not assigned anything to it. Further up your code, where you should have assigned something to it, you didn't - and this is easily fixed!
Here:
mFactTextView = (TextView) findViewById(R.id.factTextView);
mFactTextView = (Button) findViewById(R.id.showFactButton);
You have assigned your view and your button into mFactTextView
. You should have assigned the button into mShowFactButton
. So, change the lines above to this:
mFactTextView = (TextView) findViewById(R.id.factTextView);
mShowFactButton = (Button) findViewById(R.id.showFactButton);
That will clear the error you're currently getting but it may reveal more - so have a go and see if that fixes it. If not, shout back here,
Good luck!
Steve.
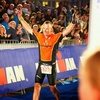
Steve Hunter
57,712 PointsHi Shamir,
Across the bottom of Android Studio, you should have the 'Logcat' area which displays messages relating to your app. In there, you're likely to find some red error messages that were generated at the point your app crashed. If you paste those messages in here, we may be able to help solve your problem.
We're likely to need to see your code too - paste that in first, it may be clear what the issue is from looking at your code. But pasting the error messages in too will really help.
Steve.

johnsdoe
Courses Plus Student 4,920 PointsHi, thanks for the reply. I guess the problem is with the .java file. Here are the codes:
package com.buildultra.funfacts;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import java.util.Random;
public class FunFacts extends AppCompatActivity {
//Declare our View variables
private TextView mFactTextView;
private Button mShowFactButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts);
// Assign the Views from the layout file to the corresponding variables
mFactTextView = (TextView) findViewById(R.id.factTextView);
mFactTextView = (Button) findViewById(R.id.showFactButton);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View v) {
String[] facts = {
"Ants stretch when they wake up in the morning.",
"Ostriches can run faster than horses.",
"Olympic gold medals are actually made mostly of silver.",
"You are born with 300 bones; by the time you are an adult you will have 206.",
"It takes about 8 minutes for light from the Sun to reach Earth.",
"Some bamboo plants can grow almost a meter in just one day.",
"The state of Florida is bigger than England.",
"Some penguins can leap 2-3 meters out of the water.",
"On average, it takes 66 days to form a new habit.",
"Mammoths still walked the earth when the Great Pyramid was being built." };
// The button was clicked, so update the fact TextView with new fact
String fact = "";
// Randomly select a fact
Random randomGenerator = new Random();
int randomNumber = randomGenerator.nextInt(facts.length);
fact = facts[randomNumber];
// Update the screen with our dynamic fact
mFactTextView.setText(fact);
}
};
mShowFactButton.setOnClickListener(listener);
}
}

johnsdoe
Courses Plus Student 4,920 PointsHere are the errors:
--------- beginning of crash
08-05 16:44:05.584 2658-2658/? E/AndroidRuntime: FATAL EXCEPTION: main
Process: com.buildultra.funfacts, PID: 2658
java.lang.RuntimeException: Unable to start activity ComponentInfo{com.buildultra.funfacts/com.buildultra.funfacts.FunFacts}: java.lang.NullPointerException: Attempt to invoke virtual method 'void android.widget.Button.setOnClickListener(android.view.View$OnClickListener)' on a null object reference
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2665)
at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2726)
at android.app.ActivityThread.-wrap12(ActivityThread.java)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1477)
at android.os.Handler.dispatchMessage(Handler.java:102)
at android.os.Looper.loop(Looper.java:154)
at android.app.ActivityThread.main(ActivityThread.java:6119)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:886)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:776)
Caused by: java.lang.NullPointerException: Attempt to invoke virtual method 'void android.widget.Button.setOnClickListener(android.view.View$OnClickListener)' on a null object reference
at com.buildultra.funfacts.FunFacts.onCreate(FunFacts.java:52)
at android.app.Activity.performCreate(Activity.java:6679)
at android.app.Instrumentation.callActivityOnCreate(Instrumentation.java:1118)
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2618)
at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2726)
at android.app.ActivityThread.-wrap12(ActivityThread.java)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1477)
at android.os.Handler.dispatchMessage(Handler.java:102)
at android.os.Looper.loop(Looper.java:154)
at android.app.ActivityThread.main(ActivityThread.java:6119)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:886)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:776)

Thomas Becket
19,820 PointsHi, I get a similiar error:
10-12 10:31:46.555 2781-2781/com.example.held.funfacts E/AndroidRuntime: FATAL EXCEPTION: main
Process: com.example.held.funfacts, PID: 2781
java.lang.RuntimeException: Unable to start activity ComponentInfo{com.example.held.funfacts/com.example.held.funfacts.FunFactsActivity}: java.lang.NullPointerException: Attempt to invoke virtual method 'void android.widget.Button.setOnClickListener(android.view.View$OnClickListener)' on a null object reference
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2665)
at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2726)
at android.app.ActivityThread.-wrap12(ActivityThread.java)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1477)
at android.os.Handler.dispatchMessage(Handler.java:102)
at android.os.Looper.loop(Looper.java:154)
at android.app.ActivityThread.main(ActivityThread.java:6119)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:886)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:776)
Caused by: java.lang.NullPointerException: Attempt to invoke virtual method 'void android.widget.Button.setOnClickListener(android.view.View$OnClickListener)' on a null object reference
at com.example.held.funfacts.FunFactsActivity.onCreate(FunFactsActivity.java:31)
at android.app.Activity.performCreate(Activity.java:6679)
at android.app.Instrumentation.callActivityOnCreate(Instrumentation.java:1118)
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2618)
at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2726)
at android.app.ActivityThread.-wrap12(ActivityThread.java)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1477)
at android.os.Handler.dispatchMessage(Handler.java:102)
at android.os.Looper.loop(Looper.java:154)
at android.app.ActivityThread.main(ActivityThread.java:6119)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:886)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:776)
And this is my code:
package com.example.held.funfacts;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
public class FunFactsActivity extends AppCompatActivity {
// Declare our View variables
private TextView factTextView;
private Button showFactButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts);
// Assign the Views from the layout file to the corresponding variables
factTextView = (TextView) findViewById(R.id.factTextView);
showFactButton = (Button) findViewById(R.id.showFactButton);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View v) {
// The Button was clicked, so update the fact TextView with a new fact
String fact = "Ostriches can run faster thean horses";
factTextView.setText(fact);
}
};
showFactButton.setOnClickListener(listener);
}
}
Can you please help me as well?
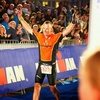
Steve Hunter
57,712 PointsGive me a minute ... I'll have a quick look.
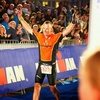
Steve Hunter
57,712 PointsIn your XML layout file, is your button definitely called showFactButton
?
The error is complaining that you're setting the listener on a null object which is throwing two exceptions. One makes it unable to start the activity. The other is a null pointer on the button.
Paste your xml in, or just check what you've called the widget in your layout file.
Steve.

Thomas Becket
19,820 PointsThank you! I accidentally called it ShowFactButton. I renamed it and now it works!
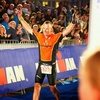
Steve Hunter
57,712 PointsCool. Glad it worked.
johnsdoe
Courses Plus Student 4,920 Pointsjohnsdoe
Courses Plus Student 4,920 PointsThank You, Steve!
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsNo problem!