Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial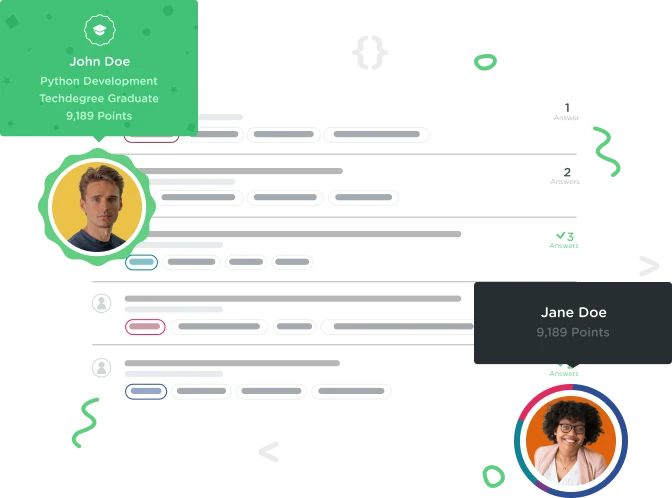

Hannah Elliott
11,001 PointsFunctions have proven to be a real challenge for me.
Can someone walk me through this code challenge? thanks!
2 Answers
John Coolidge
12,614 PointsHi Hannah,
To begin, you can declare the function, like so:
var max = function() {
};
Now, the function will take two arguments. Arguments go inside the parenthesis and are separated by a comma. You can have as many arguments as you want, but this code challenge asks for two. You can name them what you want; I'm going to name them number and otherNumber.
var max = function(number, otherNumber) {
};
Now you need to use a conditional statement that will run that compares the numbers together to see which one is larger. An if/else statement will do.
var max = function(number, otherNumber) {
if (number > otherNumber) {
return number;
} else {
return otherNumber;
}
};
When the function is called, it will look like this:
max(1, 2);
The function will evaluate which number is larger (in this case, 1 or 2) and return the larger.
For the second portion of the code challenge, you need to call the function and use an alert to display which number is greater. You can do that by putting the function inside of the alert parenthesis like so:
alert(max(1, 2));
And that should solve the code challenge. If there is still anything you don't understand, please don't hesitate to ask me more questions.
John

Jacob Mishkin
23,118 PointsJohn Coolidge I think did a very detailed breakdown of the challenge, but in order for you to understand functions better I have to ask, what do you think you should do? It's one thing to get an answer but to know is to go through the process.