Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial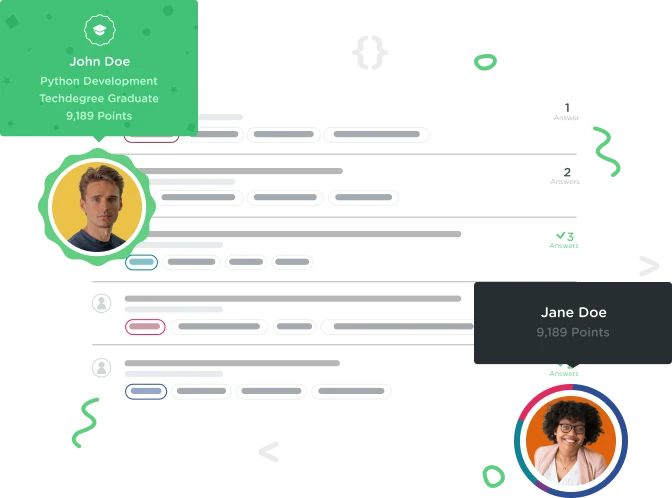

Jeana Koerber
2,397 PointsFunctions question
Hi, I am working on a function challenge and am stuck. I'm having a hard time wrapping my head around the instructions. Can anyone point me in the right direction?
Challenge is: After your newly created returnValue function, create a new variable named echo. Set the value of echo to be the results from calling the returnValue function. When you call the returnValue function, make sure to pass in any string you'd like for the parameter.
The function will accept the string you pass to it and return it back (using the return statement). Then your echo variable will be set with what is returned, the results.
And my answer is: (the test told me the first part is right, but I'm not understanding why it's right, having a hard time with these!
function returnValue(coffee) { return coffee; }
var echo = returnValue(tea); returnValue();
function returnValue(coffee) {
return coffee;
}
var echo = returnValue(drink);
returnValue();
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
3 Answers

Alexander La Bianca
15,960 PointsHi Jeana,
You are close. However, the question is asking to input a string as a parameter to the function. In your function definition 'function returnValue(coffee) { return coffee; }' you are saying that whatever is input into the function will simply be returned back to you. For you to better understand I changed the javascript code below a bit:
//This is your function definition. You are defining what the function should be doing so your program can use it anywhere
function returnValue(inputString) {
return inputString;
}
var echo = returnValue("drink"); //echo will now be the string "drink"
var echo2 = returnValue("Coffee"); //echo2 will now be the string "Coffee"
var echo3 = returnValue(25); //echo3 will now be the number 25 (see the function can return anything not only strings)
var echo4 = returnValue("Teamtreehouse is dope"); //echo4 will now be the string "Teamtreehouse is dope"
//returnValue(); // you do not need this line. this simply executes the function without a value given to it
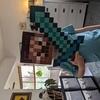
Gabbie Metheny
33,778 PointsYou're really close! So, right now you've got this:
function returnValue(coffee) {
return coffee;
}
var echo = returnValue(tea);
returnValue();
In returnValue
, you're creating a function that will simply return
whatever argument you pass into it. When a function returns a value, you can store that value in a variable. (In real life, you'd be creating a function that does something with an argument before returning a value, but this challenge just wants to make sure you're grasping the basics.)
When you actually call the function, which you're doing on the line where you created var echo
, you need to pass in a string as your argument. In creating the function, you're effectively saying coffee
is the variable name for whatever argument you will pass in when calling the function. Strings need to go in single or double quotes, otherwise they won't be recognized as strings, so make sure your tea
argument is in quotes! This line of code will store the results of returnValue('tea')
(so, 'tea'
,) in echo
.
One last thing, looks like you're calling the function an additional time at the end, with no argument. Just delete that line, you don't need it! Let me know if you need any further clarification!

Jeana Koerber
2,397 PointsThank you so much!