Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial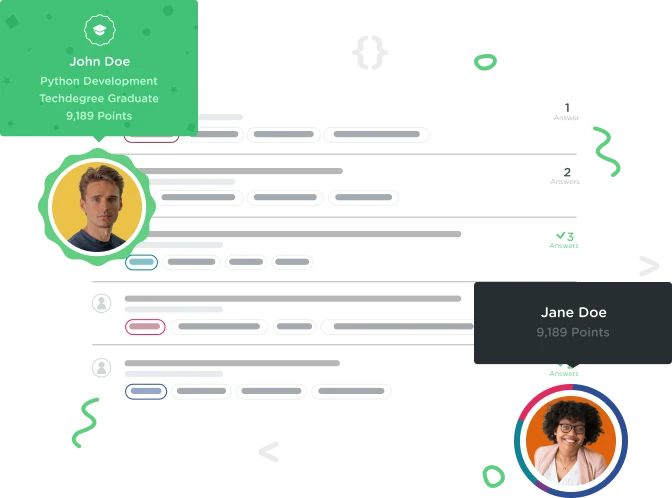
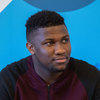
Mohammed Jammeh
37,463 PointsFunctions - Using numbers as parameters
Hi, I am struggling to solve a question under JavaScript basics - functions.
The question reads "Create a new function named max() which accepts two numbers as arguments. The function should return the larger of the two numbers. You'll need to use a conditional statement to test the 2 numbers to see which is the larger of the two".
The code below is my answer but it keeps saying there is parse error within the code which I believe is related to the numbers I'm using. I will really appreciate it if you could help. Thanks!
function max (10,15) {
if (15>10) {
return true;
} else {
return false;
}
}
4 Answers
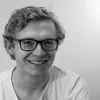
Luke Glazebrook
13,564 PointsHi Mohammed!
Your code is almost perfect! Instead of putting numbers straight into the function you have to put some variable names which can then be used to refer to the number you pass to the function.
function max(num1, num2) {
if(num1>num2) {
return true;
} else {
return false;
}
}
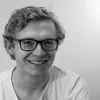
Luke Glazebrook
13,564 PointsI'm glad you got it working.
When you are declaring a function you can give it some arguments (variables) that it can use within the function. These can be named anything like num1, word, thisIsASentence and etc.
Whereas 1 or 50 is something that you would pass to the function so it can use it.
function max(num1, num,2) {
}
max(50, 25)
In the case above num1 is 50 and num2 is 25. I hope this helped clear up your confusion a bit.
-Luke
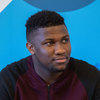
Mohammed Jammeh
37,463 PointsIt surely did. Thank you man; I really appreciate it.
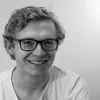
Luke Glazebrook
13,564 PointsNo problem! Have fun learning JavaScript!

Chanse Campbell
4,453 Points+1 Thanks for this guys, I was having major issues with this challenge for some reason but this really cleared it up for me :)
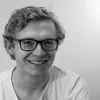
Luke Glazebrook
13,564 PointsGood job Chanse! Keep up the great work!

A FRENZ
2,207 PointsI think there asking to return a number here:
var num1 = 2; var num2 = 3;
function max(num1, num2) { if(num1>num2) { return num1; } else { return num2; } }
Mohammed Jammeh
37,463 PointsMohammed Jammeh
37,463 PointsThanks Luke.
With the help of your answer, I have solved it. I just remembered variables do not begin with numbers.
For some reason, the Boolean values were not working either. I had to put the parameters beside 'return' instead of true/false. For example, instead of:
I had to put:
By the way, thanks man :)
Luke Glazebrook
13,564 PointsLuke Glazebrook
13,564 PointsI am glad you managed to figure it out! Have fun learning JavaScript!
Hope you are having a good day Mohammed.
-Luke
Mohammed Jammeh
37,463 PointsMohammed Jammeh
37,463 PointsThanks Luke, you too.
I have managed to answer the first question of the challenge but I'm now stuck on the second one ha ha.
Luke Glazebrook
13,564 PointsLuke Glazebrook
13,564 PointsWould you like some assistance with that question too?
Mohammed Jammeh
37,463 PointsMohammed Jammeh
37,463 PointsYes please:
Question: Call the function and display the results in an alert dialog. You can pass the results of a function directly to the alert() method.
My solution:
.. but it's not passing through.
Luke Glazebrook
13,564 PointsLuke Glazebrook
13,564 PointsWhat you need to do in this case Mohammed is what you were doing for task 1. Try passing in some integer values and see what happens!
I hope this helps.
-Luke
Mohammed Jammeh
37,463 PointsMohammed Jammeh
37,463 PointsIt worked, thanks a lot man.
If you don't mind, what's the difference between 'num1' and the integer '1'?