Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial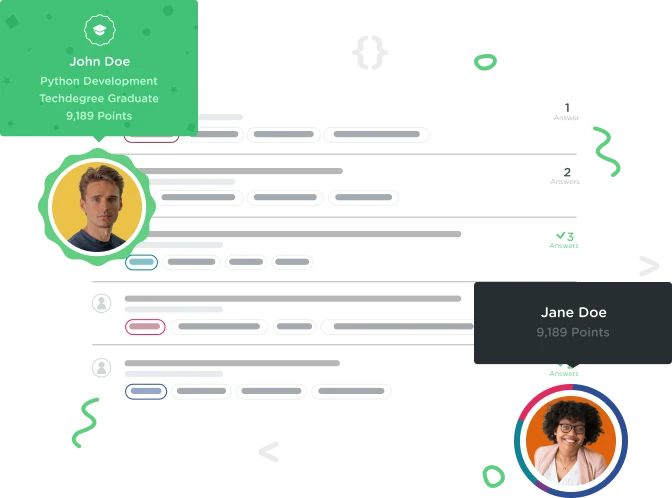
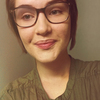
Pauliina K
1,973 PointsgetFirstName
Hey! I get this error: " Bummer! Please add a getter method to expose the first name. Name it getFirstName." I don't understand how to write it. I tried this, but that gets tons of errors, so I'm lost. How do I "expose" the first name?
public String getFirstName() {
return mFirstName;
This is my current code in User.java:
private mFirstName;
private mLastName;
public User(String firstName, String lastName) {
mFirstName = firstName;
mLastName = lastName;
1 Answer
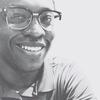
Ricardo Hill-Henry
38,442 PointsYou're missing the closing bracket for your getFirstName() method as well as setting the class type for your variables at the beginning of the class. You'll also need a getter method for the mLastName variable.
public class User {
private String mFirstName;
private String mLastName;
public User(String firstName, String lastName) {
// TODO: Set the private fields here
mFirstName = firstName;
mLastName = lastName;
}
public String getFirstName(){
return mFirstName;
}
public String getLastName(){
return mLastName;
}
}
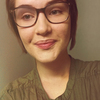
Pauliina K
1,973 PointsAh! I had just forgotten to write in the closing bracket here, because it was in the code. But, it was in the wrong place however, so thanks! And I did forget the setting type for my variables. Btw, how do you know if the setting type is supposed to be String? How do you know it's a string? Boolean and int's are easier to figure out.
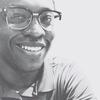
Ricardo Hill-Henry
38,442 PointsThe two variables would be instances of the String class because they're both given values from arguments (lastName & firstName) that are of type String when a user is instantiated. If not, you'd get an error. Also, if the two getter methods have been set to return a String, then the values held in mFirstName and mLastName would have to be Strings.
Gandhi Kotari
7,574 PointsGandhi Kotari
7,574 PointsYou forgot to declare data type to mFirstName and mLastName.