Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial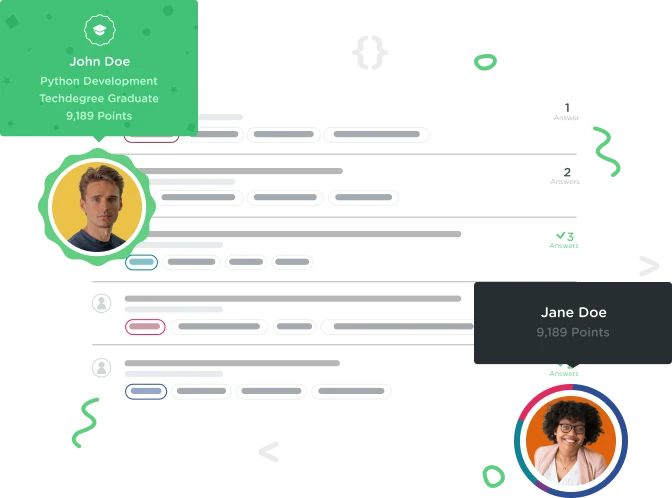
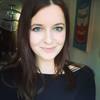
Marina Alenskaja
9,320 PointsgetTileCount - need help understanding the logic
Hi
I have just completed the challenge "for each loop", with a correct answer. But I can't seem to wrap my head around the logic here.. Can someone please explain it in a simple way? :-)
This is my code:
public int getTileCount(char tile) {
int count = 0;
for (char letter: mHand.toCharArray()) {
if(letter == tile) {
count += 1;
}
}
return count
}
What I don't understand are the to local variables "tile" (the argument) and "letter". Does this mean that when I call the function I would write for example "a" as an argument and it would then see how many a's I have on hand using the letter variable?
Thanks in advance.
4 Answers
Rebecca Rich
Courses Plus Student 8,592 PointsDon't be sorry; it's good that you are asking questions until you understand this.
Remember that the argument for the method is just one character, as opposed to a String. So for example, you could have an input of the character 'b' and this method (if mHand was "bob") would return 2. This way you know that you have two tiles of the character 'b'.
Based on a quick glance of the challenge, the goal is just to know how many tiles of a specific character you have in your Scrabble hand. This could be useful for some other method that you might write for trying to form a word for your next play. Say I wanted to try to play the word "banana" in a Scrabble game off of a 'b' tile already on the board ... I would need to make sure that I had 2 'n' characters and 3 'a' characters in my hand. If I had all of those, I would know that I could play that word. That's one use case I could think of... there are probably many more.
You could also have a method for returning the size of your hand. We could call that getHandSize()
and it could return mHand.length(). I think for the majority of the Scrabble game (until tiles from the draw pile start to run out), you will have 7 tiles in your hand. However, you could use a method like this to figure out how many more tiles you need to draw at the end of a play or to try to narrow down a dictionary of words that you might be able to play, etc.
Let me know if you have any additional questions.

Nuri Bayram
6,493 Pointsyou can only put in a character as argument in this method
so lets say tile = "b" (you put in as argument like this: getTileCount(b);
- mHand = "bob" (letter wil now take ["b", "o", "b"])
- first loop in for loop: letter = "b"
- is letter b same as tile? yes! count=1
- second loop: now letter = "o"
- is letter b same as tile? no! count=1
- third loop(because array has 3 letters) letter = "b"
- is letter b same as tile? yes! count=2 8 not for loop ends, because there are no more letters in the mHand array
- return 2 (count value)
the key to programming is only understand in what order the variables are manipulated.
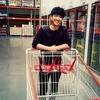
Jae Kim
3,044 PointsJust to make sure I understood this:
So if the tile = "o" and I put it as the argument like so: getTileCount(o);
- mHand = "bob" In total the count value should be 1 correct?
Rebecca Rich
Courses Plus Student 8,592 PointsHi Marina,
The getTileCount
method is designed to find the number of time the character tile
exists in the mHand object's characters. I am assuming that mHand is a String defined somewhere in the class containing this method.
Let's take a look at how this is working:
The toCharArray
method converts the mHand object (or String) into an array of characters. For example, let's say that mHand is a String: "bob". mHand.toCharArray() would return an array of the characters in mHand, e.g. ['b', 'o', 'b'].
The for loop notation for (char letter: mHand.toCharArray())
is the equivalent of walking through all of the characters in that array, each time placing that character in a variable called letter. This is equivalent to the following for loop if mHand is a String:
for (int i = 0; i < mHand.length(); i ++) {
char letter = mHand.charAt(i);
...
// now we can use the variable "letter"
...
}
So each time we run through the for loop we are comparing the current character we are on in the array of characters from mHand to the character (or "tile") we are trying to count the occurrences of. If the two characters match we know the "tile" has been seen. , so we increase the count.
When we have gone through all of the characters in mHand, we can return the count.
Hope this helps!
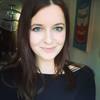
Marina Alenskaja
9,320 PointsSo basically if I put in "bob" as an argument, and the mHand had stored "bob", it would return 3? But then I don't understand what the purpose is - why would I need to use this method? I mean, if I want to know how many tiles I have, I can't keep writing out the entire alphabet just to see how many tiles I have? Or did I misunderstand something? I'm sorry - I'm a bit slow when it comes to logic, and I want to make sure I understand this..
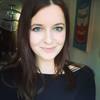
Marina Alenskaja
9,320 PointsThank you both of you, that was really helpful!! :-)
Nuri Bayram
6,493 PointsNuri Bayram
6,493 PointsHi Marina,
The code takes any kind of character you want to put in like "a" and uses that to compare with the letter. The actual letter variable gets filled by the mHand.toCharArray method. (mhand is probably an object that is defined somewhere) So whatever is in mHand object will be put to the letter variable.
if the letter variable is the same as the variable "a" that you put in, then it will return 1 back to you with the getTilefunction. So you could use this 1 somewhere else.
for example int x = getTileCount(a);