Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial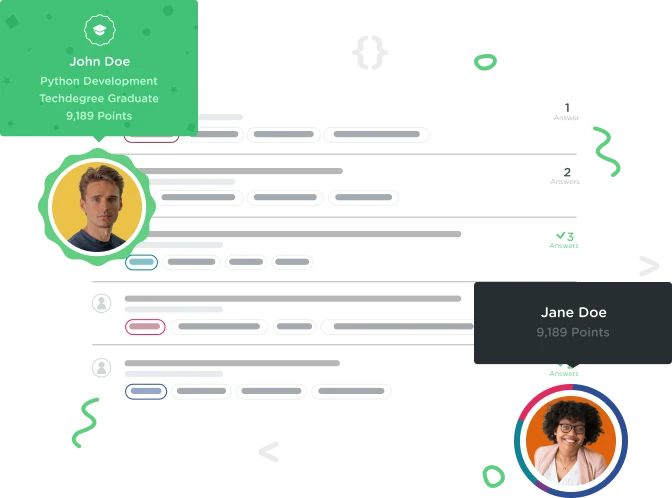

Unsubscribed User
5,777 PointsgetTileCount returning too high a count?
Hi all, thanks in advance for your help! I'm not sure I understand the feedback I'm getting when I submit. It's telling me that "The hand was "sreclhak" and "e" was checked. Expected 1 and got 8." I thought we wanted to know how many tiles the player had in their hand?
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
public int getTileCount(char tile) {
int tileCount = 0;
for (char aTile : mHand.toCharArray()) {
if (mHand.indexOf(tile) >= 0); {
tileCount += 1;
}
}
return tileCount;
}
}
1 Answer
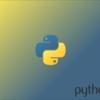
Kevin Faust
15,353 PointsHey Kathleen,
Your so close!!
Let's take a look at what you got here:
public int getTileCount(char tile) {
int tileCount = 0;
for (char aTile : mHand.toCharArray()) {
if (mHand.indexOf(tile) >= 0); {
tileCount += 1;
}
}
return tileCount;
}
you've got everything up to the for loop correct. Let's take a look at what's going on inside the for loop. You are basically looping through each char in the mHand and storing it as a char called aTile each time. However did you notice how you didn't make any reference to that aTile variable after that line of code? What you can do is simply check if the aTile is equal to the tile we pass in.
if (aTile == tile); {
tileCount += 1;
}
Its as simple as that. Every time we loop through that mHand array, we pull out a character from it (the aTile) and check if it equals to the one we pass in (tile).
We can't use the indexOf() method because that is only used with strings. However all we're doing is just checking if our char == the chars in the mHand array. Everything we do just involves chars!
one minor thing, you should take out the semi colon at the end of your if statement: if (mHand.indexOf(tile) >= 0)
I hope you understand what I'm trying to say and I hope you learned something!
As always, happy coding and dont forget to mark as best answer so others can see
Kevin
Unsubscribed User
5,777 PointsUnsubscribed User
5,777 PointsI really appreciate your help Kevin! Funny story, the first time I tried the code you gave me I must've tweaked something just slightly because I got a different feedback statement of something like, "The hand was "delbefk, "e" was checked. Expected 2 got 1." For the life of me I can't figure out what I did to get that error. The next time I tried submitting though, I passed the challenge. Thanks again!
Kevin Faust
15,353 PointsKevin Faust
15,353 PointsGood thing we got it all solved! Glad to help :)