Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial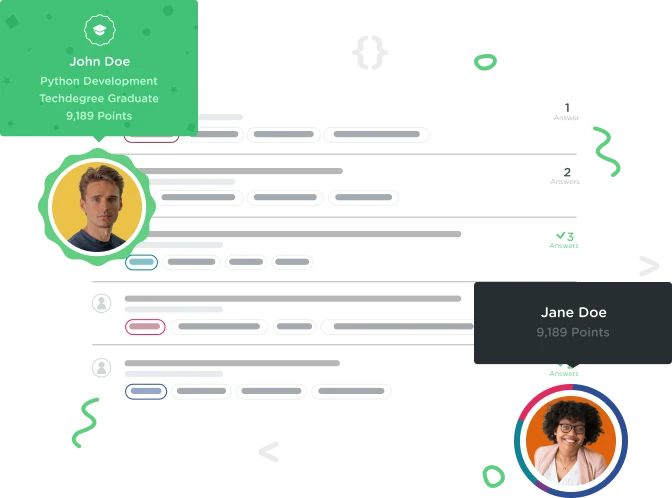
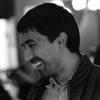
Martin Cornejo Saavedra
18,132 PointsHack-n-Slash (python) ported to Ruby !
Here is the code I ported to play this game from the python Object Oriented Programming course. Hope it is useful.
module Combat
def combat_initialize
@atk_limit = 8
@dodge_limit = 5
end
def dodge
roll = rand(1..@dodge_limit)
return true if roll > 4
return false
end
def attack
roll = rand(1..@atk_limit)
return true if roll > 4
return false
end
end
require "./Combat.rb"
COLORS = ["yellow", "green", "red", "blue"]
module Monster
include Combat
attr_accessor :hitpoints, :experience, :name
def monster_initialize
@min_hitpoints ||= 1
@max_hitpoints ||= 1
@min_exp ||= 1
@max_exp ||= 1
@weapon ||= "sword"
@sound ||= "roar"
@name = self.class
@hitpoints = rand(@min_hitpoints..@max_hitpoints)
@experience = rand(@min_exp..@max_exp)
@color = COLORS.sample
combat_initialize
end
def to_s
"#{@color.capitalize} #{self.class}, HP: #{@hitpoints}, XP: #{@experience}"
end
def battlecry
"#{@sound.upcase}!"
end
end
class Goblin
include Monster
def initialize
@max_hitpoints = 3
@max_exp = 3
@sound = "groar"
monster_initialize
end
end
class Troll
include Monster
def initialize
@min_hitpoints = 3
@max_hitpoints = 5
@min_exp = 2
@max_exp = 4
@sound = 'growl'
monster_initialize
end
end
class Dragon
include Monster
def initialize
@min_hitpoints = 5
@max_hitpoints = 10
@min_exp = 4
@max_exp = 10
@sound = 'rooooooar'
monster_initialize
end
end
1 Answer
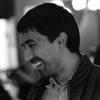
Martin Cornejo Saavedra
18,132 Pointsrequire "./Combat.rb"
class Character
include Combat
attr_accessor :hitpoints, :name, :weapon, :experience
def initialize
print "Name: "
@name = gets.chomp
@attack_limit = 6
@experience = 0
@base_hitpoints = 10
@hitpoints = @base_hitpoints
@weapon = get_weapon
combat_initialize
end
def get_weapon
puts "Weapon ([S]word, [A]xe, [B]ow:) "
weapon = gets.chomp.downcase
if 'sab'.include?(weapon)
case weapon
when 's'
"sword"
when 'a'
"axe"
when 'b'
"bow"
end
else
puts("Invalid weapon. Try again.")
get_weapon
end
end
def attack
roll = rand(1..@attack_limit)
case @weapon
when "axe"
roll += 1
when "sword"
roll += 2
end
roll > 4
end
def rest
if @hitpoints < @base_hitpoints
@hitpoints += 1
end
end
def leveled_up
@experience >= 5
end
def to_s
"#{@name}, #{@weapon.capitalize}, HP:#{@hitpoints}, XP:#{@experience}"
end
end
'''
main of the game
run this file game.rb and the game will start
'''
require "./Monster.rb"
require "./character.rb"
class Game
def setup
@player = Character.new
@monsters = [Dragon.new,
Troll.new,
Goblin.new
]
@monster = get_next_monster
end
def get_next_monster
@monsters.pop
end
def monster_turn
if @monster.attack
puts "#{@monster.name} is attacking!"
unless @player.dodge
puts "#{@player.name} loses hitpoints."
@player.hitpoints -= 1
else
puts "#{@player.name} dodged monster attack!"
end
else
puts "#{@monster.name} didn't attack."
end
end
def player_turn
print "[A]ttack, [R]est or [Q]uit:> "
move = gets.chomp.downcase
if "arq".include?(move) and move != ''
case move
when 'a'
if @player.attack
puts "#{@player.name} is attacking to a #{@monster.name} with a #{@player.weapon}!"
unless @monster.dodge
if @player.leveled_up
@monster.hitpoints -= 2
else
@monster.hitpoints -= 1
end
else
puts "#{@monster.name} dodged your attack!"
end
else
puts "Your attack failed."
end
when 'r'
@player.rest
puts "#{@player.name} gains hitpoints."
when 'q'
@player.hitpoints = 0
end
else
puts "Input not valid. Try again."
player_turn
end
end
def cleanup
if @monster.hitpoints <= 0
puts "#{@monster.name} is dead."
@player.experience += @monster.experience
if @player.leveled_up
puts "#{@player.name} leveled up!"
end
@monster = get_next_monster
end
end
def initialize
setup
while @player.hitpoints > 0 and @monster
puts '=' * 50
puts @player
puts @monster
puts '=' * 50
monster_turn
break if @player.hitpoints <= 0
puts '-' * 50
player_turn
cleanup
end
if @player.hitpoints and !@monster
puts "You win!"
else
puts "You loose.."
end
end
end
game = Game.new