Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial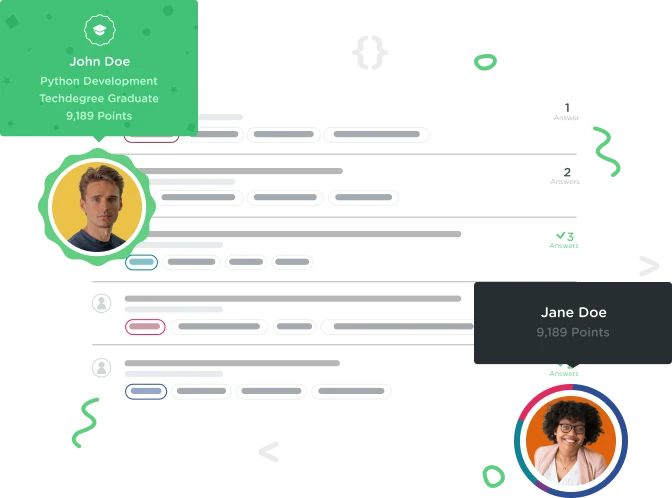
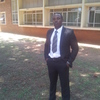
Brett Dube
2,653 PointsHARNESSING THE POWER OF OBJECTS
"Are you sure you threw the illegalargumentexception....." This is the bummer i am getting, can you help me understand what i am doing wrong.
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive() {
drive(1);
}
public void Drive (int laps) {
int newCharge = mBarsCount-laps;
if (newCharge < 0) {
throw new IllegalArgumentException ("Not enough battery remains");
}
mBarsCount= newCharge;
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
mBarsCount -= laps;
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}
2 Answers
William Li
Courses Plus Student 26,868 PointsHi, Brett Dube , I believe we've discussed about this very challenge several days ago, right?
I see that you code looks a bit different this time.
public void Drive (int laps) {
int newCharge = mBarsCount-laps;
if (newCharge < 0) {
throw new IllegalArgumentException ("Not enough battery remains");
}
mBarsCount= newCharge;
}
You've added this new method to the class template provided by the Challenge, you should NOT do that, because that's not what the challenge is looking for. The only spot you should make change to is here.
public void drive(int laps) {
// Other driving code omitted for clarity purposes
mBarsCount -= laps;
}
the ONLY task here is to add code to drive
method body as told by the Challenge, and leave other parts of the code template alone.
so let's throw an IllegalArgumentException from the drive method if the requested number of laps cannot be completed on the current battery level.
You only need to add 2 lines of code to pass this challenge.
public void drive(int laps) {
// Other driving code omitted for clarity purposes
if (isBatteryEmpty()) {
throw new IllegalArgumentException("Not enough battery remains");
}
mBarsCount -= laps;
}
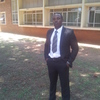
Brett Dube
2,653 PointsThank you so much man, i truly appreciate. sorry for the trouble.
William Li
Courses Plus Student 26,868 Pointsno trouble at all, happy to help out, if you have more question, feel free to post it on the forum.