Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial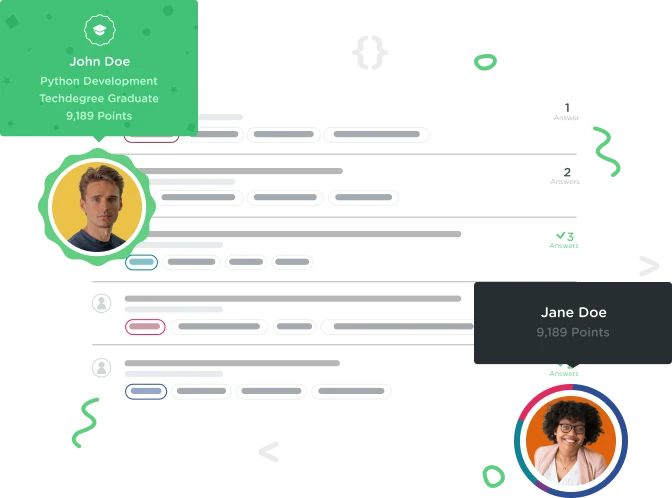

Antoine DuBois
3,130 PointsHaving a problem with this one
``public class Example {
public static void main(String[] args) {
System.out.println("We are going to create a GoKart");
GoKart String = new GoKart ("red");
System.out.printf("The GoKart is %n\", String.getColor());
}
}
public class Example {
public static void main(String[] args) {
System.out.println("We are going to create a GoKart");
GoKart String = new GoKart ("red");
System.out.printf("The GoKart is %n\", String.getColor());
}
}
1 Answer

Stone Preston
42,016 Pointsyou have a few things wrong with your code:
first you named your GoKart object String. that is a bad idea since String is a class in java already so whenever you reference your GoKart called String it probably thinks you mean the String class. name it something else like goKart:
GoKart goKart = new GoKart ("red");
then in your printf you used %n as the format specifier instead of %s. you need to use %s because getColor returns a string, and %s is the format specifier for strings. remember to reference the new name of the GoKart, goKart
GoKart goKart = new GoKart ("red");
System.out.printf("The GoKart is %s", goKart.getColor());
below is a table of common format specifiers obtained from this page
<table> <tbody> <tr> <td>%c</td> <td>character</td> </tr> <tr> <td>%d</td> <td>decimal (integer) number (base 10)</td> </tr> <tr> <td>%e</td> <td>exponential floating-point number</td> </tr> <tr> <td>%f</td> <td>floating-point number</td> </tr> <tr> <td>%i</td> <td>integer (base 10)</td> </tr> <tr> <td>%o</td> <td>octal number (base 8)</td> </tr> <tr> <td>%s</td> <td>a string of characters</td> </tr> <tr> <td>%u</td> <td>unsigned decimal (integer) number</td> </tr> <tr> <td>%x</td> <td>number in hexadecimal (base 16)</td> </tr> <tr> <td>%%</td> <td>print a percent sign</td> </tr> <tr> <td>\%</td> <td>print a percent sign</td> </tr> </tbody> </table>