Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial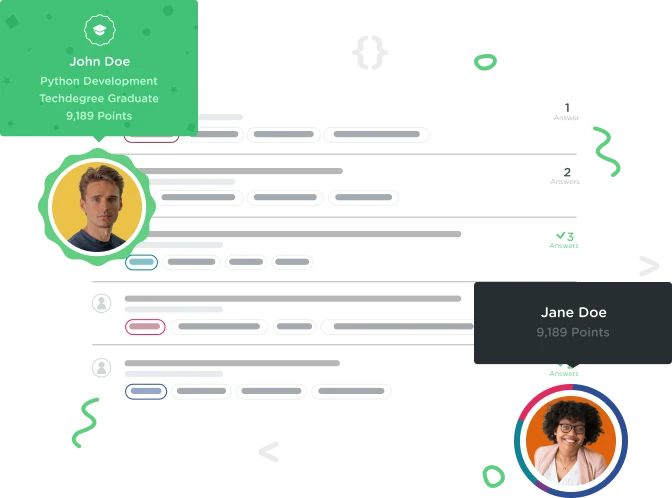

Brian Pitre
2,293 PointsHaving difficulty with escaping output on a code challenge.
I am on the last challenge in the "Build a Basic PHP Website" course, and I am having difficulty completing it. The error message received when using the code below is, "Bummer! I still see some malicious code in there. You want to escape the name variable before displaying it to the screen." I am not sure how else to escape the output even after reviewing the videos in the course and trying a search for output escape methods in PHP.
Here is the code so far: <?php if ($_POST == "POST") { $listing_name = trim(filter_input(INPUT_POST,"name",FILTER_SANITIZE_STRING)); $listing_link = trim(filter_input(INPUT_POST,"link",FILTER_SANITIZE_STRING)); $listing_description = trim(filter_input(INPUT_POST,"description",FILTER_SANITIZE_SPECIAL_CHARS));
if ($listing_name == "" || $listing_link == "") {
$error_message = "Fill in all fields. Thanks!";
}
require_once("controllers_listing.php");
exit;
}
?>
<html> <body> <?php if (isset($error_message)) { echo "<p>".$error_message . "</p>"; } else { echo "<h1>Edit Listing</h1>"; } ?>
<form method="post" action="views_listing_edit.php">
<table>
<tr>
<th>
<label for="name">Name</label>
</th>
<td>
<input id="name" name="name" value="<?php if (isset($listing_link)) {
echo htmlspecialchars($_POST["name"]); } ?>">
</td>
</tr>
<tr>
<th>
<label for="Link">Link</label>
</th>
<td>
<input id="link" name="link" value="<?php if (isset($listing_link)) {
echo htmlspecialchars($_POST["link"]); } ?>">
</td>
</tr>
<tr>
<th>
<label for="Description">Description</label>
</th>
<td>
<textarea id="description" name="description"><?php if (isset($listing_description)) {
echo htmlspecialchars($_POST["description"]); } ?></textarea>
</td>
</tr>
</table>
<input type="submit" value="Save">
</form>
</body> </html>
2 Answers
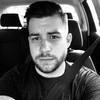
Szabolcs Szána
6,961 Pointsoh i see :) now i understand. you are think the problem too difficult :)
this is the solution for your problem:
<?php require_once("controllers_listing.php"); ?><html>
<body>
<h1>Edit Listing</h1>
<form method="post">
<table>
<tr>
<th>
<label for="name">Name</label>
</th>
<td>
<input id="name" name="name" value="<?php echo htmlspecialchars($listing_name); ?>">
</td>
</tr>
<tr>
<th>
<label for="Link">Link</label>
</th>
<td>
<input id="link" name="link" value="<?php echo htmlspecialchars($listing_link); ?>">
</td>
</tr>
<tr>
<th>
<label for="Description">Description</label>
</th>
<td>
<textarea id="description" name="description"><?php echo htmlspecialchars($listing_description); ?></textarea>
</td>
</tr>
</table>
<input type="submit" value="Save">
</form>
</body>
</html>
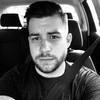
Szabolcs Szána
6,961 PointsHi,
in my opinion your discription is too difficult. please write the code challange's text or link it :) i don't understand more things your code, so it is not as simple and I can't help now :(

Brian Pitre
2,293 PointsThank you for the reply. Here is a link to the challenge: https://teamtreehouse.com/library/build-a-basic-php-website/enhancing-a-form/escaping-output-2
Szabolcs Szána
6,961 PointsSzabolcs Szána
6,961 Pointsand even a good tip into which I had fallen into the past :)
you haven't got $POST variable (used in if). so if u want to check the form use the isset() or maybe empty() functions. here is the documention page
Brian Pitre
2,293 PointsBrian Pitre
2,293 PointsThank you for the correct answer. However, this answer displays all of the styling information in the text area when previewed. How is this acceptable as being correct?