Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial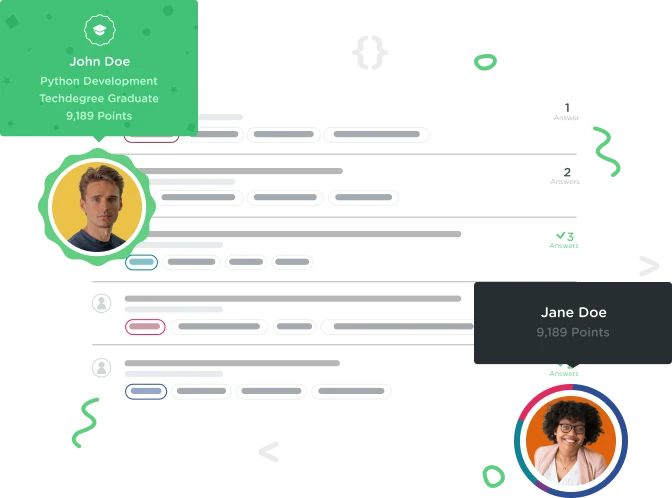

brendon hanson
6,191 PointsHelp Me With FUNCTIONS
SInce I started javascript I have absolutely no issues with anything except functions! For some reason i just simply don't understand them! could you please help me with this one and maybe give me an explanation that could help me understand it more...It's very frustrating!
function returnValue(apple) {
var echo = I dont know;
return apple;
}
returnValue('Apples are good');
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
1 Answer

Samuel Ferree
31,722 PointsA function is a special type of subroutine. Specifically, a function is a bit of code, that may accept input data (as parameters), and may return a single value.
Let's look at a super simple function
function add2(x) {
return x+2;
}
var six = add2(4);
var eight = add2(six);
console.log(six) // prints '6'
console.log(eight) //prints '8'
console.log(add2(10)) //prints '12'
So the data passed in, is a single parameter, a number, that we can use in the function as the variable x. The function adds 2 to x, and returns the result. We capture this result in other parts of our program and use it by assigning it to a variable, or as in the last example, passing it into another function (console.log is a function).
Here are some more examples of functions
//Takes two parameters
function min(a, b) {
if(a < b) {
return a;
} else {
return b;
}
}
//Doesn't return anything
function printToConsole(x) {
console.log(x);
}
Hope this helps.

brendon hanson
6,191 PointsThanks for the help, I still don't fully understand but that helped me enough to finish the challenge thanks! I'm just gonna keep trying to get the hang of it
Iagor Moraes
18,678 PointsIagor Moraes
18,678 PointsMy friend, you created a function called "returnValue" with a parameter "apple", inside that function you maked a mistake, you assigned a "echo" variable: "var echo = I dont know;" this is wrong, you are not receiving the apple parameter and putting to the "echo" variable, thats why your code broke, and you must put string in a double or single quote, your correct function above:
then the text "apples are good" will be printed. Hope you understands whats going on now.