Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial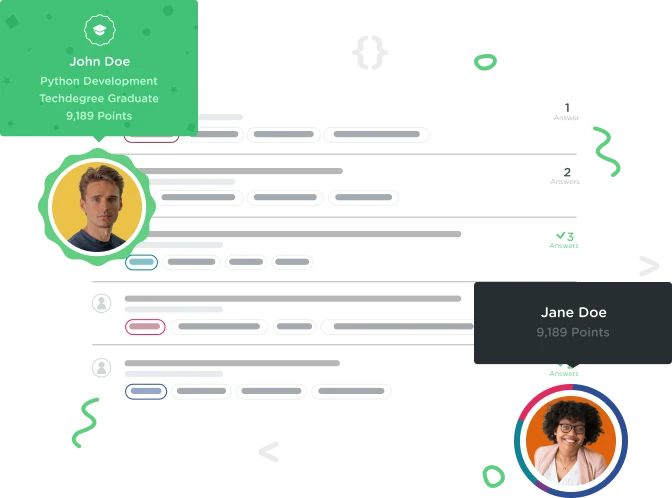
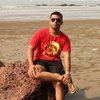
adriantennison
3,090 PointsHelp needed with the code
final quiz not able to complete
public class ForumPost {
private User mAuthor;
private String mTitle;
private String mDescription;
public ForumPost(User author, String title, String description){
mAuthor = author;
mTitle = title ;
mDescription = description ;
}
public void getDescription () {
System.out.printf("enter the description"+ mDescription );
}
public User getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
}
public class User {
private String mFirstName;
private String mLastName;
public User(String firstName, String lastName) {
mFirstName = firstName;
mLastName = lastName ;
}
public void getFirstName(String mFirstName)
{
System.out.printf(" first name %s"+mFirstName);
}
public void getLastName(String mLastName){
System.out.printf(" last name %s"+mLastName);
}
}
public class Forum {
private String mTopic;
public String getTopic() {
return mTopic;
}
Forum(String topic)
{
mTopic = topic;
}
public void addPost(ForumPost post) {
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
}
}
public class Example {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
Forum forum = new Forum("Java");
User author = new User("adrian","tennison");
ForumPost post = new ForumPost("adri","java", "funprogram");
forum.addPost(post);
}
}
2 Answers
Aaron Kaye
10,948 PointsSo there are a couple issues that I will address, challenge 2 asks you to create the member variables, assign the values to the member variables from the constructor, and add the appropriate getters, you did the first two correct but you have an issue with your getters.
Lets step through your code: (I will look at the first name but the same applies to the last name)
public void getFirstName(String mFirstName)
{
System.out.printf(" first name %s"+mFirstName);
}
First, we want to return a string so the method is not void. Remember this is the return type and you are saying we are not going to return anything. The value there should be switched to String. Also, we do not need to accept a parameter like you are asking for (String mFirstName). Getters are about getting information, you would need provide the first name for a setter (opposite of a getter, used to set a variable). So hopefully that part makes sense, we don't need a parameter because we do not know it, we are asking the object to give US the name. Lastly, you are not returning, you are only printing. We need a return statement because we may want to use the variable we get here wherever we called the method from. Please let me know if you need anymore clarification on this and I'd be happy to help. Your new methods should look like this:
public String getFirstName() {
return mFirstName;
}
public String getLastName() {
return mLastName;
}
For the last challenge: There is an issue with this line:
ForumPost post = new ForumPost("adri","java", "funprogram");`
Here is what the compiler says: ./Example.java:12: error: incompatible types: String cannot be converted to User ForumPost post = new ForumPost("adri","java", "funprogram");
So we have a hint, String cannot be converted to user. That is because you specified a string "adri" instead of a user Object. Remember our ForumPost constructor:
public ForumPost(User author, String title, String description){
mAuthor = author;
mTitle = title ;
mDescription = description ;
}
It wants a User, String, String. You are passing it a String, String, String. You need to pass it the user object you created so it looks like this:
ForumPost post = new ForumPost(author,"java", "funprogram");
where the author variable was the variable you defined the line before:
User author = new User("adrian","tennison");
Sorry for the long winded answer but hope that helps! Let me know if any other issues arise!

Mat Sanders
4,819 PointsI see a problem in Forum.java...
Forum(String topic)
{
mTopic = topic;
}
Should be:
Public void Forum(String topic)
{
mTopic = topic;
}
Aaron Kaye
10,948 PointsMethods are public by default and constructors do not include void. His code there was correct but I personally would have included the public/private:
public Forum(String topic) {
mTopic = topic;
}

Seth Kroger
56,416 PointsActually the default visibility is only within the class's package, not outside it. It's sometimes called "package local" which is a step down from fully public.
adriantennison
3,090 Pointsadriantennison
3,090 PointsThis helped me a lot Aaron Kaye .