Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial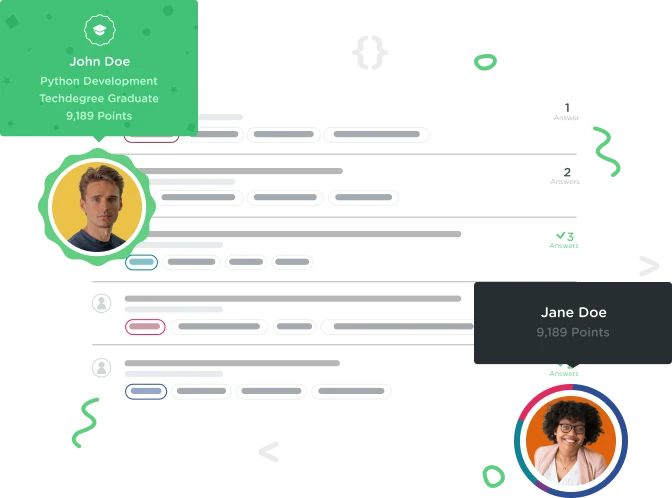

Mark Libario
9,003 PointsHelp with C# basic Averager Project
using System;
namespace Treehouse.FitnessFrog
{
class Program
{
static void Main()
{
Console.WriteLine ("Enter a number or type \"done\" to see the average.");
var totalCount = 0.0;
var averageNumber = 0.0;
var answer = Console.ReadLine ();
try
{
var number = double.Parse(answer);
while (true)
{
if (answer.ToLower().Equals("done"))
{
Console.WriteLine ("Average: " + averageNumber);
break;
}
else
{
number += 1;
continue;
}
}
}
catch (FormatException)
{
Console.WriteLine ("That is not a valid input.");
}
}
}
}
I dont know what Im doing wrong.
First off, I dont want the catch to override the Console.WriteLine when someone responds with "done". Everytime I put done, it just says That is not a valid input and it just ends.. if though it satisfies that if { condition.
2nd, I wanted that everytime someone put an number answer, it will be added to my variable averageNumber and then it will print the one in my Console.WriteLine saying "Totals : " + averageNumber
The only thing thats happening right now is when i put a number, it adds nonestop but only shows 0 over and over again.
and when i type done, it says "That is not a valid input", instead of saying the average.
What will I need to do so i can add every number in the input and then average all those numbers? ahhhhhhhhhhhhhhhh :(
1 Answer
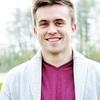
Simon Owerien
12,829 Pointstry
{
var number = double.Parse(answer);
If you type 'done' this throws an exception which is catched and you get the message "That is not a valid input.". That's the reason you don't get the average number if you write 'done'.
else
{
number += 1;
continue;
}
Here you increment the 'number'-variable instead of incrementing the total count. Also you have your while loop in the wrong position. You just wait once for the user input and after that you always evaluating this input.
I guess now you can figure out how you have to change your code so it works.