Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial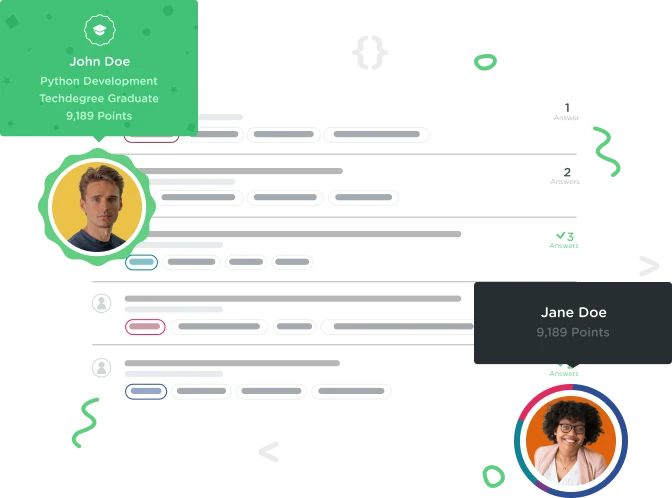

Mike B
10,424 PointsHelp with getTileCount in the scrabble exercise
I'm confused by the method type. I'm creating a getTileCount method to return an integer ("count"), but the error I'm getting prompts me that the method should accept a char instead of an int
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
public int getTileCount(int count)
{
count = 0;
{
for (char letter: mHand.toCharArray())
{
if (mHand.indexOf(letter) >= 0)
count += 1;
}
}
return count;
}
}
2 Answers
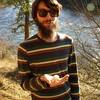
Nico Julian
23,657 PointsHi,
The getTileCount should return an int like you have it, but it should take a char as an argument. That way, when you call it and pass it a letter, it will return the count for specifically that letter. Using indexOf will only tell you if the letter is present in the player's hand, but not how many they actually have. When you use the for loop to iterate all the tiles the player has, you can check each letter to see if it matches the one you passed the method; if it matches, it'll increase the count by one.
public int getTileCount(char letter) {
int count = 0;
for (char tile: mHand.toCharArray()) {
if (tile == letter) {
count += 1;
}
}
return count;
}

julian itwaru
972 Pointsinstead of count += 1, you could just use count++

Mike B
10,424 PointsThanks....I'm always forgetting about that shortcut!
Mike B
10,424 PointsMike B
10,424 PointsThank you so much....I didn't realize you could pass an argument that wasn't the same type as the method. That's what I kept getting hung up on