Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial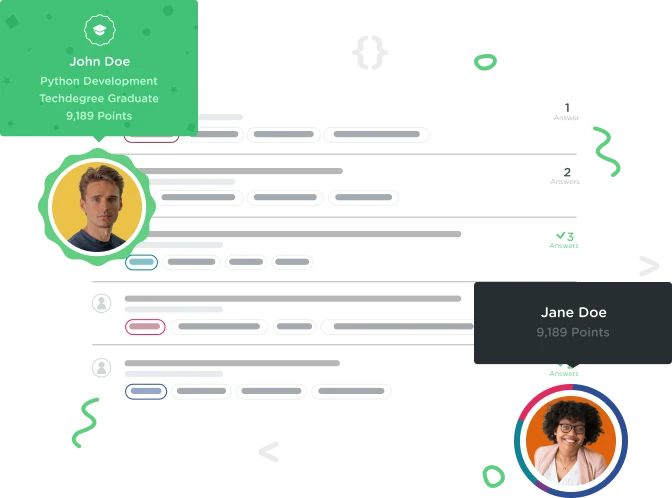
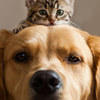
Devin Scheu
66,191 PointsHelp With Java
Question: Okay, so let's throw an IllegalArgumentException from the drive method if the requested number of laps cannot be completed on the current battery level. Make the exception message "Not enough battery remains".
My Code:
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
newCharge = mBarsCount - laps;
if (newCharge > MAX_BARS) {
System.out.printf("Not enough battery remains");
}
mBarsCount = newCharge;
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}
4 Answers

Stone Preston
42,016 Pointsalright so if the laps cant be completed on the current charge we need to throw an exception.
how do we tell if the laps can be completed on the current charge?
well if we substract the laps from the current number of bars and that dips below zero then we cant complete those laps. we will use that as our condition
then inside the if statement we need to throw a new exception. we can do that by using
throw new WhateverExceptionWeWant("some message");
we want to throw an IllegalArgumentException and pass in a special message
finally, we need to set the mBarsCount variable to the newCharge. if the exception is thrown the function will stop right there, if not, it will continue on, so we can put this at the very end of the function
public void drive(int laps) {
// Other driving code omitted for clarity purposes
//find out what the new charge will be if we complete the laps
int newCharge = mBarsCount - laps;
//if the laps take our charge below zero, its not possible to do that many
if (newCharge < 0) {
//throw the exception and pass it the message
throw new IllegalArgumentException("Not enough battery remains");
}
//this code wont run if the exception is thrown, but if its all good, then set the mBarsCount to the newCharge
mBarsCount = newCharge;
}
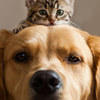
Devin Scheu
66,191 PointsHey Stone, I am stuck on number 2 of this challenge also.

ISAIAH S
1,409 PointsHi partha chakraborty, please see my answer on this post.
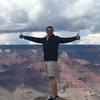
Andrew Wynyard
3,718 PointsHi Guys,
I was reading through this after making a silly mistake ("}" in the wrong place) and thought it has to be simpler. I had this:
public void drive(int laps) { // Other driving code omitted for clarity purposes if (mBarsCount < 1) { throw new IllegalArgumentException("Not enough battery remains"); } mBarsCount -= laps; }
and it seems a bit more straight forward than what the mod has up. Anyways, just thought I would share.
Devin Scheu
66,191 PointsDevin Scheu
66,191 PointsAh I see, thanks for pointing this out Stone!
partha chakraborty
10,562 Pointspartha chakraborty
10,562 PointsIn the below code, why can't I decrement first, and then throw the exception?
mBarsCount -= laps; if (mBarsCount<1) { throw new IllegalArgumentException("Not enough battery remains"); }