Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial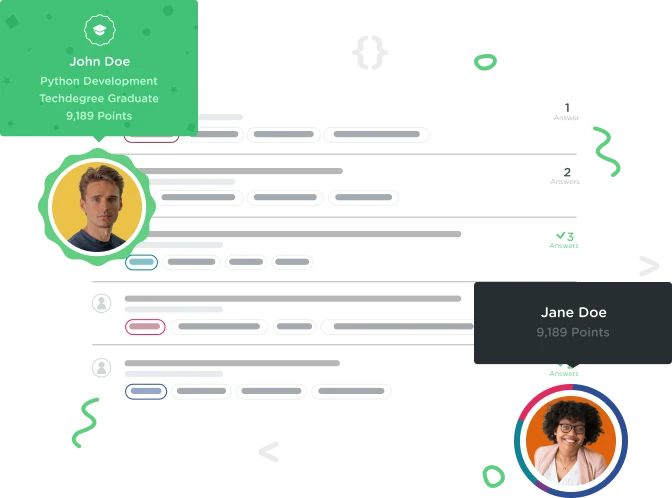
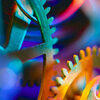
ja5on
10,338 PointsHelp with my JavaScript code please..
hello, after my inner.HTML i have alot of address.houseNumber address.Street address.City address.State
etc ... is there any way to make this shorter? simplier?
3 Answers

Blake Larson
13,014 PointsYou can use object destructuring and template strings using es6. It's cleaner to look at and you don't have to call the object.key over and over. It's also very common on front end frameworks and libraries for import statements. Template Strings are just backticks (``) and to use a variable you can just use ${variable} so you don't have to concat everything with spaces and plus signs.
let person = {
firstName: "John",
lastName: "Doe",
age: 50,
eyeColor: "blue",
};
let { firstName, lastName, age, eyeColor } = person; // <-- DESCTRUCTED PERSON OBJECT
document.getElementById(
"demo"
).innerHTML = `${firstName} is ${age} years old <br /> ${eyeColor} eye color`;
let address = {
houseNumber: "31770 ",
street: "Alvarado Blvd ",
city: "Union City",
state: "California(CA)",
country: "USA",
zipcode: "94587",
phone: "(510)489-5531",
};
let {
houseNumber,
street,
city,
state,
country,
zipcode,
phone,
} = address; // <-- DESCTRUCTED ADDRESS OBJECT
document.getElementById(
"demo2"
).innerHTML = `${houseNumber} ${street} <br />
${city}<br />
${state}<br />
${country}<br />
${zipcode}<br /><br />
("Phone:")
${phone}`;
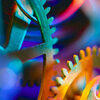
ja5on
10,338 Pointsoh yes i just noticed that ("phone:") upon running the code and i was wondering, question was my original code ok or was it not really best practise?

Blake Larson
13,014 PointsYeah, your original code was basically the exact same just the syntax makes it harder for the developer to read, but it runs the same. The only thing that I would get use to changing is let instead of var. It's the preferred way to declare variable.
If you want to read on that. Its not a massive difference but it's better to learn now and get used to: --> https://www.freecodecamp.org/news/var-let-and-const-whats-the-difference/
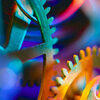
ja5on
10,338 PointsVery interesting Thanks, you've helped me a lot, i will definitely give (let) more consideration!
ja5on
10,338 Pointsja5on
10,338 Pointsi'm very new to this so i will study your code, thank you..
Blake Larson
13,014 PointsBlake Larson
13,014 PointsYup no problem, and you can take the quotation marks out of the ("phone:") part too. I didn't notice that I left that in so the quotes will be output in the HTML.