Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial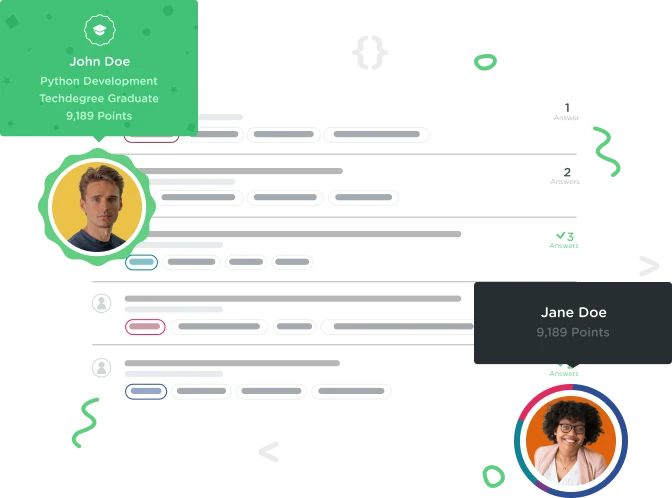

William J. Terrell
17,403 PointsHelp with Nested Content in C# and/or ASP.NET
I'm working on a page for our University's Academic Checklist. The goal is to provide students with a checklist that presents course offerings and can be printed off and filled in.
Part of the checklist involves electives. The way their structured in the database is, each Elective Group has a type, in a column called "GRP_REL_TYP".
If the GRP_REL_TYP for an Elective Group is "AND", the Elective Group is "Required" for the major, and we want to list out all of the courses, one on each row, so that the student can go through and mark them off as their taken.
If the GRP_REL_TYP for an Elective Group is "OR", than any courses listed for that Elective Group can be taken to meet the requirement. In this case, we want to display the courses in a block-list and provide the student with blank lines, so he or she can fill them in with the classes they choose.
Right now, I have it mostly working. The problem is that, for Elective Groups where GRP_REL_TYP is set to "AND", it displays the list of courses row-by-row, but also displays them in the block-list section. The block-list should only display for items where GRP_REL_TYP is set to "OR" and accompanied by blank lines.
Here is an example page, if it can be useful to visualize the result.
I've got the block list in a div with an ID of "divBlocklist3", and am trying to set Visible to "False" when the GRP_REL_TYP for an Elective Group is set to "AND". This way, it will just display the list of courses row-by-row.
When I try to run the code though, I get a Compilation Error, telling me that "divBlocklist3 does not exist in the current context".
I believe this is because, on the front-end page, divBlocklist3 is nested within the Repeater "RequiredElectives", and so is not seen by the back-end code.
I apologize for the length of this question, but it is quite complex.
The Front-End and Back-End code is below.
Any assistance would be appreciated.
Thanks!
Front-End Page:
<%@ Page Title="" Language="C#" MasterPageFile="/main.master" AutoEventWireup="true"
CodeFile="checklist_specific_test.aspx.cs" Inherits="checklist_specific" %>
<%@ MasterType VirtualPath="~/main.master" %>
<%@ Register TagPrefix="rightmenu" TagName="rightmenu" Src="right_menu.ascx" %>
<asp:Content ID="Content1" ContentPlaceHolderID="head" runat="Server">
</asp:Content>
<asp:Content ID="Content2" ContentPlaceHolderID="mainText1" runat="Server">
<div name="TopFields">
<table>
<tr><th><asp:Label ID="lbname" runat="server" Text=""></asp:Label></th>
<th><asp:Label ID="lbcatalog" runat="server" Text=""></asp:Label> Catalog</th>
</tr>
<tr><td colspan="2">ID#:</td></tr>
<tr><td colspan="2">Name:</td></tr>
<tr><td colspan="2">Advisor:</td></tr>
</table>
</div>
<p style="font-weight:bold;text-align: center;">
**Students are required to have <asp:Label ID="lbcrd" runat="server" Text="122"></asp:Label> distinct credits for graduation**
</p>
<!--Program Notes, if any-->
<div name="divProgramNotes" id="divProgramNotes" runat="server" Visible="false">
<hr/>
<p><asp:Label ID="lbProgramNote" runat="server" Text=""></asp:Label></p>
</div>
<!-- (To display only if major is B.A.) -->
<div name="BAFields" id="BAFields" runat="server" visible="false">
<hr/>
<table>
<tr><th>Bachelor of Arts degree programs require a minor and a year of a foreign language.</th></tr>
<tr><td>Minor:</td></tr>
<tr><td>Foreign Language:</td></tr>
</table>
</div>
<!-- (To display only if there are concentrations associated with this major) -->
<div name="concentrationFields" id="concentrationFields" runat="server" visible="false">
<hr/>
<table>
<tr><th>Concentration (if applicable)</th></tr>
<tr><td>Concentration:</td></tr>
</table>
</div>
<div runat="server" id="RequiredCourseList">
<hr/>
<p style="font-weight:bold;">Required Courses: <asp:Label ID="lbRequiredCourseTotalHrs" runat="server" Text=""></asp:Label> credits</p>
<table width="500">
<tr><th>
Course
</th>
<th>
Course Title
</th>
<th>
Credit
</th>
<th>
Semester Completed
</th>
<th>
Grade Earned
</th>
<th>
Substitutions
</th></tr>
<asp:Repeater ID="Repeater1" runat="server">
<ItemTemplate>
<tr>
<td>
<!--<a href="course_detail.aspx?CRS_CDE=<%# DataBinder.Eval(Container.DataItem, "CRS_CDE") %>">-->
<%# DataBinder.Eval(Container.DataItem, "CRS_CDE") %><!--</a>-->
</td>
<td>
<%# DataBinder.Eval(Container.DataItem, "CRS_TITLE")%>
</td>
<td align="center" width="75">
<%# DataBinder.Eval(Container.DataItem, "DFLT_CREDIT_HRS")%>
</td>
<td></td>
<td></td>
<td></td>
</tr>
</ItemTemplate>
</asp:Repeater>
</table>
</div>
<!-- //////////////////////////// Concentration Information //////////////////////////// -->
<div runat="server" id="divConCredits" visible="false">
<hr/>
<p style="font-weight:bold;">Concentration Requirements: <asp:Label ID="lbConcCredits" runat="server" Text=""></asp:Label> credits</p>
</div>
<div runat="server" id="divConcentrations" visible="false">
<table width="500">
<asp:Repeater ID="rptConcentrations" runat="server" OnItemDataBound="rptConcentrations_ItemDataBound">
<ItemTemplate>
<tr><th>
Course
</th>
<th>
Credit
</th>
<th>
Semester Completed
</th>
<th>
Grade Earned
</th>
<th>
Substitutions
</th></tr>
<tr style="font-weight:bold;">
<td><!--Title of Concentration-->
<!--<a href="concentration_details.aspx?concentration=<%# DataBinder.Eval(Container.DataItem, "concentration") %>">-->
<%# DataBinder.Eval(Container.DataItem, "REQ_TXT")%><!--</a>-->
</td>
<td align="center" width="75">
<%# DataBinder.Eval(Container.DataItem, "MIN_HRS")%>
</td>
<td></td>
<td></td>
<td></td>
</tr>
<asp:Repeater ID="rpt2" runat="server">
<ItemTemplate>
<tr>
<td><%# DataBinder.Eval(Container.DataItem, "REQ_TXT")%> <%# DataBinder.Eval(Container.DataItem, "CRS_CDE")%> <%# DataBinder.Eval(Container.DataItem, "crs_title")%></td>
<td style="text-align:center"><%# DataBinder.Eval(Container.DataItem, "DFLT_CREDIT_HRS")%></td>
<td></td>
<td></td>
<td></td>
</tr>
</ItemTemplate>
</asp:Repeater>
<tr runat="server" id="ConcentrationNotesRow" Visible="true">
<td colspan="5" id="ConcentrationNotes">
<strong>Notes:</strong><br/><%# DataBinder.Eval(Container.DataItem, "CONCENTRATION_NOTE")%>
</td>
</tr>
<!--nested table begins here-->
<tr><td colspan="5">
<div runat="server" class="accordion loop-12" id="divConcentrationElectives" Visible="true">
<div class="accordion-item">
<div class="accordion-heading"><h3 class="icon-arrow-swop">
Electives for <%# DataBinder.Eval(Container.DataItem, "REQ_TXT")%> <!--Concentration Electives-->
</h3></div><!-- End Accordion Heading -->
<div class="accordion-content" style="display: none;">
<table>
<tr>
<th>
Course
</th>
<th>
Credit
</th>
<th>
Semester Completed
</th>
<th>
Grade Earned
</th>
<th>
Substitutions
</th>
</tr>
<asp:Repeater ID="rpConcentrationElective" runat="server" OnItemDataBound="rpConcentrationElective_ItemDataBound">
<ItemTemplate>
<tr style="font-weight:bold;">
<td>
<!--<a href="elective_details.aspx?ADV_TREE_REQ_CDE=<%# DataBinder.Eval(Container.DataItem, "ADV_TREE_REQ_CDE") %>&Adv_Req_Code=<%# DataBinder.Eval(Container.DataItem, "ADV_REQ_CODE") %>&level=<%# DataBinder.Eval(Container.DataItem, "level") %>">-->
<%# DataBinder.Eval(Container.DataItem, "REQ_TXT")%><!--</a>-->
</td>
<td align="center" width="75">
<%# DataBinder.Eval(Container.DataItem, "MIN_HRS")%>
</td>
<td></td>
<td></td>
<td></td>
</tr>
<asp:Repeater ID="rpConcentrationElective_course" runat="server">
<ItemTemplate>
<tr>
<td>
<%# DataBinder.Eval(Container.DataItem, "CRS_CDE")%>
<%# DataBinder.Eval(Container.DataItem, "crs_title")%>
</td>
<td align="center" width="75">
<%# DataBinder.Eval(Container.DataItem, "dflt_credit_hrs")%>
</td>
<td></td>
<td></td>
<td></td>
</tr>
</ItemTemplate>
</asp:Repeater>
</ItemTemplate>
</asp:Repeater>
</table>
</div><!-- End Accordion Content -->
</div><!-- End Accordion Item -->
</div><!-- End Accordion Loop-12 -->
</td></tr>
<!--end nested table-->
</ItemTemplate>
</asp:Repeater>
</table>
</div>
<!-- //////////////////////////// Elective Information //////////////////////////// -->
<div runat="server" id="divElectCredits" visible="false">
<hr/>
<p style="font-weight:bold;">Required Electives: <asp:Label ID="lbElectCredits" runat="server" Text=""></asp:Label> credits</p>
</div>
<div runat="server" id="divRequiredElectives" visible="false">
<table width="500">
<tr><th>
Course
</th>
<th>
Hours
</th>
<th>
Semester Completed
</th>
<th>
Grade Earned
</th>
<th>
Substitutions
</th></tr>
<asp:Repeater ID="RequiredElectives" runat="server" OnItemDataBound="RequiredElectives_ItemDataBound">
<ItemTemplate>
<tr style="font-weight:bold;">
<td>
<!--<a href="elective_details.aspx?ADV_TREE_REQ_CDE=<%# DataBinder.Eval(Container.DataItem, "ADV_TREE_REQ_CDE").ToString().Trim() %>&Adv_Req_Code=<%# DataBinder.Eval(Container.DataItem, "ADV_REQ_CODE") %>&level=<%# DataBinder.Eval(Container.DataItem, "level") %>">-->
<%# DataBinder.Eval(Container.DataItem, "REQ_TXT")%><!--</a>-->
</td>
<td align="center" width="75">
<%# DataBinder.Eval(Container.DataItem, "MIN_HRS")%>
</td>
<td></td>
<td></td>
<td></td>
</tr>
<!--If "or", list out all the courses in a block-->
<div id="divBlocklist3" runat="server" Visible="true">
<tr><td colspan="5">
<strong>Courses that can be taken include the following:</strong>
<asp:Repeater ID="blocklist3" runat="server" Visible="true">
<ItemTemplate>
<%# DataBinder.Eval(Container.DataItem, "CRS_CDE")%> <%# DataBinder.Eval(Container.DataItem, "CRS_TITLE")%> - <%# DataBinder.Eval(Container.DataItem, "DFLT_CREDIT_HRS")%> Credits</ItemTemplate>
<SeparatorTemplate>, </SeparatorTemplate>
</asp:Repeater>
</td></tr>
</div>
<!-- End block list -->
<!--If "and", list all the courses out on their own rows-->
<asp:Repeater ID="rpt3" runat="server">
<ItemTemplate>
<tr>
<td><%# DataBinder.Eval(Container.DataItem, "REQ_TXT")%> <%# DataBinder.Eval(Container.DataItem, "CRS_CDE")%> <%# DataBinder.Eval(Container.DataItem, "crs_title")%></td>
<td style="text-align:center"><%# DataBinder.Eval(Container.DataItem, "DFLT_CREDIT_HRS")%></td>
<td></td>
<td></td>
<td></td>
</tr>
</ItemTemplate>
</asp:Repeater>
<!--End row list-->
</ItemTemplate>
</asp:Repeater>
</table>
</div>
<div name="signatures">
<hr/>
<table>
<tr><th colspan="2">Signatures:</th></tr>
<tr><td>Student:</td><td>Date:</td></tr>
<tr><td>Advisor:</td><td>Date:</td></tr>
<tr><td>Division Chair:</td><td>Date:</td></tr>
<tr><td colspan="2"><small>Substitutions to the coursework above requires the signature of the division chair.</small></td></tr>
</table>
</div>
</asp:Content>
<asp:Content ID="Content3" ContentPlaceHolderID="RightContent" runat="Server">
<rightmenu:rightmenu ID="rightmenu1" runat="server" />
</asp:Content>
Back-End Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Globalization;
using System.Configuration;
using System.Data;
using System.Data.SqlClient;
public partial class checklist_specific : System.Web.UI.Page
{ /* ----------------------------------------------------- Base/General Content ----------------------------------------------------- */
protected void Page_Load(object sender, EventArgs e) //Gets the main set of information for up top
{
Master.strPageTitle = "Checklist";
if (!Page.IsPostBack)
{
SqlConnection con = new SqlConnection(common.ConnectionString("EX_ProdConnectionString"));
string sql = "select ";
sql = sql + " ATT.ADV_TREE_REQ_CDE, MMD.MAJOR_CDE, ATT.ADV_TREE_YR_CDE, DEGR_CDE ";
sql = sql + " ,REQ_TXT = ";
sql = sql + " case ";
sql = sql + " when ATT.REQ_TXT like '%~%' ";
sql = sql + " then LEFT(ATT.REQ_TXT,CHARINDEX('~',ATT.REQ_TXT)-1) ";
sql = sql + " else ATT.REQ_TXT ";
sql = sql + " end ";
sql = sql + " ,SUBSTRING(ATT.REQ_TXT,CHARINDEX('~',ATT.REQ_TXT)+1,LEN(ATT.REQ_TXT)) AS PROGRAM_NOTE ";
sql = sql + " from ADV_TREE_TABLE ATT ";
sql = sql + " JOIN MAJOR_MINOR_DEF MMD ON SUBSTRING(ATT.ADV_TREE_REQ_CDE,2,LEN(ATT.ADV_TREE_REQ_CDE)) = MMD.MAJOR_CDE ";
sql = sql + " JOIN ADV_TREE_TABLE ATT2 ON SUBSTRING(ATT.ADV_TREE_REQ_CDE,2,LEN(ATT.ADV_TREE_REQ_CDE)) = SUBSTRING(ATT2.PAR_ADV_REQ_CDE,2,LEN(ATT2.PAR_ADV_REQ_CDE)) ";
sql = sql + " where ATT.ADV_TREE_YR_CDE = @Parameter1 ";
sql = sql + " AND ATT.ADV_TREE_REQ_CDE = @Parameter2 ";
sql = sql + " AND MMD.MAJOR_MINOR_DESC NOT LIKE '~%' ";
sql = sql + " AND ATT.ADV_REQ_TYPE = 'ROOT' ";
sql = sql + " and ATT2.ADV_TREE_REQ_CDE = 'MAJORS-U' ";
sql = sql + " order by ATT.ADV_TREE_REQ_CDE ";
sql = sql + " ";
SqlCommand cmd = new SqlCommand(sql, con);
cmd.Parameters.Add(new SqlParameter("@Parameter1", Request.QueryString["ADV_TREE_YR_CDE"]));
cmd.Parameters.Add(new SqlParameter("@Parameter2", Request.QueryString["ADV_TREE_REQ_CDE"]));
SqlDataReader Dr;
con.Open();
Dr = cmd.ExecuteReader();
try
{
while (Dr.Read())
{
lbname.Text = (string)DBUtils.GetNULLableString(Dr["REQ_TXT"]);
lbcatalog.Text = (string)DBUtils.GetNULLableString(Dr["ADV_TREE_YR_CDE"]);
lbProgramNote.Text = (string)DBUtils.GetNULLableString(Dr["PROGRAM_NOTE"]);
/*
if DEGR_CDE contains "ba" or "B.A.", BAFields.visible = "true"
*/
if ((DBUtils.GetNULLableString(Dr["DEGR_CDE"]).ToString().ToLower() == "ba") ||
(DBUtils.GetNULLableString(Dr["DEGR_CDE"]).ToString().ToLower() == "b.a."))
BAFields.Visible = true;
if (DBUtils.GetNULLableString(Dr["DEGR_CDE"]).ToString().ToLower() == "aa")
lbcrd.Text = "61";
if (DBUtils.GetNULLableString(Dr["DEGR_CDE"]).ToString().ToLower() == "as")
lbcrd.Text = "68";
if (DBUtils.GetNULLableString(Dr["PROGRAM_NOTE"]).ToString().ToLower() != "")
lbProgramNote.Text = (string)DBUtils.GetNULLableString(Dr["PROGRAM_NOTE"]);
divProgramNotes.Visible = true;
}
}
catch (Exception ex)
{
Literal litErrorMsg = (Literal)Master.FindControl("litErrorMsg");
litErrorMsg.Text = "<span style='color:Red'>Error: " + ex.Message + "</span>";
litErrorMsg.Visible = true;
}
finally
{
if (con.State == ConnectionState.Open)
con.Close();
}
RequiredCourseList_DataBind(); //Required Courses for the major or minor
RequiredCourseTotalHrs_DataBind();
RequiredElectives_DataBind();
ElectCredits_DataBind();
Concentrations_DataBind();
ConcCredits_DataBind();
}
}
protected void RequiredCourseList_DataBind() //Gets the list of required courses for the major
{
DataSet ds = new DataSet();
string sql;
sql = "select ";
sql = sql + " CM.CRS_CDE, crs_title = ";
sql = sql + " case ";
sql = sql + " when CM2.catalog_name_full = '' OR CM2.catalog_name_full IS NULL";
sql = sql + " then CM.crs_title";
sql = sql + " else CM2.catalog_name_full";
sql = sql + " end, CM.DFLT_CREDIT_HRS";
sql = sql + " from adv_tree_table ATT";
sql = sql + " join CATALOG_MASTER CM on ATT.ADV_REQ_CODE = CM.ADV_REQ_CDE";
sql = sql + " left outer join catalog_mstr_udf CM2 on CM.crs_cde = CM2.crs_cde";
sql = sql + " where ATT.ADV_TREE_YR_CDE = @Parameter1";
sql = sql + " and ATT.ADV_TREE_REQ_CDE = @Parameter2";
sql = sql + " and ATT.PAR_ADV_REQ_CDE = @Parameter2";
sql = sql + " and ATT.REQ_CLUSTER_CDE = 'c'";
sql = sql + " and CM.ACTIVE_INACTIVE_ST = 'a'";
sql = sql + " order by ATT.ADV_REQ_CODE";
ds = common.getDataSet("EX_ProdConnectionString", sql, new SqlParameter("@Parameter1", Request.QueryString["ADV_TREE_YR_CDE"])
, new SqlParameter("@Parameter2", Request.QueryString["ADV_TREE_REQ_CDE"])
);
Repeater1.DataSource = ds;
Repeater1.DataBind();
if (ds.Tables[0].Rows.Count == 0)
RequiredCourseList.Visible = false;
}
protected void RequiredCourseTotalHrs_DataBind() //Gets the total number of hours for the required courses (the collective range)
{
SqlConnection con = new SqlConnection(common.ConnectionString("EX_ProdConnectionString"));
string sql;
sql = "select ";
sql = sql + " sum(DFLT_CREDIT_HRS) AS TOTAL_HOURS";
sql = sql + " from adv_tree_table ATT";
sql = sql + " join CATALOG_MASTER CM on ATT.ADV_REQ_CODE = CM.ADV_REQ_CDE";
sql = sql + " where ATT.ADV_TREE_YR_CDE = @Parameter1";
sql = sql + " and ATT.ADV_TREE_REQ_CDE = @Parameter2";
sql = sql + " and ATT.PAR_ADV_REQ_CDE = @Parameter2";
sql = sql + " and ATT.REQ_CLUSTER_CDE = 'c'";
sql = sql + " and CM.ACTIVE_INACTIVE_ST = 'a'";
SqlCommand cmd = new SqlCommand(sql, con);
cmd.Parameters.Add(new SqlParameter("@Parameter1", Request.QueryString["ADV_TREE_YR_CDE"]));
cmd.Parameters.Add(new SqlParameter("@Parameter2", Request.QueryString["ADV_TREE_REQ_CDE"]));
SqlDataReader Dr;
con.Open();
Dr = cmd.ExecuteReader();
try
{
while (Dr.Read())
{
lbRequiredCourseTotalHrs.Text = (string)DBUtils.GetNULLableString((Dr["TOTAL_HOURS"]));
}
}
catch (Exception ex)
{
Literal litErrorMsg = (Literal)Master.FindControl("litErrorMsg");
litErrorMsg.Text = "<span style='color:Red'>Error: " + ex.Message + "</span>";
litErrorMsg.Visible = true;
}
finally
{
if (con.State == ConnectionState.Open)
con.Close();
}
}
protected void Concentrations_DataBind() //Gets the base concentrations available for the major
{
DataSet ds = new DataSet();
string sql;
sql = "select ";
sql = sql + " ATT2.ADV_TREE_REQ_CDE as concentration, ATT2.ADV_REQ_DESC, ATT2.MIN_HRS,";
sql = sql + " REQ_TXT = ";
sql = sql + " case ";
sql = sql + " when ATT2.REQ_TXT like '%~%' ";
sql = sql + " then LEFT(ATT2.REQ_TXT,CHARINDEX('~',ATT2.REQ_TXT)-1)";
sql = sql + " else ATT2.REQ_TXT";
sql = sql + " end";
sql = sql + " ,SUBSTRING(ATT2.REQ_TXT,CHARINDEX('~',ATT2.REQ_TXT)+1,LEN(ATT2.REQ_TXT)) AS CONCENTRATION_NOTE";
sql = sql + " from ADV_TREE_TABLE ATT ";
sql = sql + " JOIN CONCENTRATION_MAJOR CM ON CM.MAJOR_CDE = SUBSTRING(ATT.ADV_TREE_REQ_CDE,2,LEN(ATT.ADV_TREE_REQ_CDE))";
sql = sql + " JOIN ADV_TREE_TABLE ATT2 ON CM.CONC_CDE = SUBSTRING(ATT2.ADV_TREE_REQ_CDE,2,LEN(ATT2.ADV_TREE_REQ_CDE)) AND ATT2.ADV_REQ_TYPE = 'ROOT' ";
sql = sql + " where ATT.ADV_TREE_REQ_CDE = @Parameter1";
sql = sql + " and att.ADV_TREE_REQ_CDE not like '2%'"; //Won't show concentration for minors
sql = sql + " AND ATT.adv_tree_yr_cde = @Parameter2";
sql = sql + " AND ATT2.ADV_TREE_YR_CDE = @Parameter2";
sql = sql + " and ATT.ADV_REQ_TYPE = 'ROOT'";
sql = sql + " order by REQ_TXT";
ds = common.getDataSet("EX_ProdConnectionString", sql, new SqlParameter("@Parameter1", Request.QueryString["ADV_TREE_REQ_CDE"])
, new SqlParameter("@Parameter2", Request.QueryString["ADV_TREE_YR_CDE"]));
rptConcentrations.DataSource = ds;
rptConcentrations.DataBind();
if (ds.Tables[0].Rows.Count > 0)
{
divConcentrations.Visible = true;
concentrationFields.Visible = true;
}
}
protected void ConcCredits_DataBind() //Gets the total number of hours for the available concentrations (the collective range)
{
SqlConnection con = new SqlConnection(common.ConnectionString("EX_ProdConnectionString"));
string sql;
sql = "select ";
sql = sql + " CONCCREDITS.MIN_HRS,";
sql = sql + " CONCCREDITS.MAX_HRS,";
sql = sql + " case";
sql = sql + " when MAX_HRS != MIN_HRS then convert(varchar(10),MIN_HRS) + ' - ' + convert(varchar(10),MAX_HRS)";
sql = sql + " else convert(varchar(10),MIN_HRS) ";
sql = sql + " end as CONC_CREDITS";
sql = sql + " FROM";
sql = sql + " (select";
sql = sql + " min(ATT2.MIN_HRS) as MIN_HRS,";
sql = sql + " max(ATT2.MIN_HRS) as MAX_HRS ";
sql = sql + " from ADV_TREE_TABLE ATT ";
sql = sql + " JOIN CONCENTRATION_MAJOR CM ON CM.MAJOR_CDE = SUBSTRING(ATT.ADV_TREE_REQ_CDE,2,LEN(ATT.ADV_TREE_REQ_CDE))";
sql = sql + " JOIN ADV_TREE_TABLE ATT2 ON CM.CONC_CDE = SUBSTRING(ATT2.ADV_TREE_REQ_CDE,2,LEN(ATT2.ADV_TREE_REQ_CDE)) AND ATT2.ADV_REQ_TYPE = 'ROOT' ";
sql = sql + " where ATT.ADV_TREE_REQ_CDE = @Parameter1";
sql = sql + " and att.ADV_TREE_REQ_CDE not like '2%'"; //Won't show concentration for minors
sql = sql + " AND ATT.adv_tree_yr_cde = @Parameter2";
sql = sql + " AND ATT2.ADV_TREE_YR_CDE = @Parameter2";
sql = sql + " and ATT.ADV_REQ_TYPE = 'ROOT' ) CONCCREDITS";
SqlCommand cmd = new SqlCommand(sql, con);
cmd.Parameters.Add(new SqlParameter("@Parameter1", Request.QueryString["ADV_TREE_REQ_CDE"]));
cmd.Parameters.Add(new SqlParameter("@Parameter2", Request.QueryString["ADV_TREE_YR_CDE"]));
SqlDataReader Dr;
con.Open();
Dr = cmd.ExecuteReader();
try
{
while (Dr.Read())
{
lbConcCredits.Text = (string)DBUtils.GetNULLableString((Dr["CONC_CREDITS"]));
string conc = (string)DBUtils.GetNULLableString((Dr["CONC_CREDITS"]));
if (String.IsNullOrEmpty(conc))
divConCredits.Visible = false;
else
divConCredits.Visible = true;
}
}
catch (Exception ex)
{
Literal litErrorMsg = (Literal)Master.FindControl("litErrorMsg");
litErrorMsg.Text = "<span style='color:Red'>Error: " + ex.Message + "</span>";
litErrorMsg.Visible = true;
}
finally
{
if (con.State == ConnectionState.Open)
con.Close();
}
}
protected void RequiredElectives_DataBind() //Gets the base required elective groups available for the major
{
DataSet ds = new DataSet();
string sql;
sql = "select ";
sql = sql + " ADV_TREE_REQ_CDE , ADV_REQ_CODE, ADV_REQ_TYPE, ";
sql = sql + " MIN_HRS, ADV_REQ_DESC ";
sql = sql + " ,REQ_TXT = ";
sql = sql + " case ";
sql = sql + " when REQ_TXT like '%~%' ";
sql = sql + " then LEFT(REQ_TXT,CHARINDEX('~',REQ_TXT)-1) ";
sql = sql + " else REQ_TXT ";
sql = sql + " end, ";
sql = sql + " (ADV_PTY_2 + 1) as level, ";
sql = sql + " GRP_REL_TYP, BLANK_LINES = ";
sql = sql + " case";
sql = sql + " when MIN_HRS like '0.%'";
sql = sql + " then 1";
sql = sql + " else cast(ROUND(MIN_HRS/3,0) as int)";
sql = sql + " end";
sql = sql + " from ADV_TREE_TABLE ";
sql = sql + " where ADV_TREE_REQ_CDE = @Parameter1 ";
sql = sql + " and adv_tree_yr_cde = @Parameter2 ";
sql = sql + " and (PAR_ADV_REQ_CDE = @Parameter1 or PAR_ADV_REQ_CDE LIKE '%req%') ";
//sql = sql + " and ADV_PTY_2 = '1' "; //temporarily removed, attempt to display all sub-electives at once.
sql = sql + " and ADV_REQ_TYPE = 'GROUP' ";
sql = sql + " order by REQ_TXT ";
ds = common.getDataSet("EX_ProdConnectionString", sql, new SqlParameter("@Parameter1", Request.QueryString["ADV_TREE_REQ_CDE"])
, new SqlParameter("@Parameter2", Request.QueryString["ADV_TREE_YR_CDE"])
);
RequiredElectives.DataSource = ds;
RequiredElectives.DataBind();
if (ds.Tables[0].Rows.Count > 0)
divRequiredElectives.Visible = true;
}
protected void ElectCredits_DataBind() //Gets the total number of hours for the available electives (the collective range)
{
SqlConnection con = new SqlConnection(common.ConnectionString("EX_ProdConnectionString"));
string sql;
sql = " select";
sql = sql + " MIN_HRS,";
sql = sql + " MAX_HRS,";
sql = sql + " case";
sql = sql + " when MAX_HRS != MIN_HRS then convert(varchar(10),MIN_HRS) + ' - ' + convert(varchar(10),MAX_HRS)";
sql = sql + " else convert(varchar(10),MIN_HRS) ";
sql = sql + " end as ELECT_CREDITS";
sql = sql + " FROM";
sql = sql + " (select";
sql = sql + " min(MIN_HRS) as MIN_HRS,";
sql = sql + " max(MIN_HRS) as MAX_HRS ";
sql = sql + " from ADV_TREE_TABLE ATT";
sql = sql + " where ADV_TREE_REQ_CDE = @Parameter1";
sql = sql + " and adv_tree_yr_cde = @Parameter2";
sql = sql + " and (PAR_ADV_REQ_CDE = @Parameter1 or PAR_ADV_REQ_CDE LIKE '%req%')";
//sql = sql + " and ADV_PTY_2 = '1'"; //temporarily removed, attempt to display all sub-electives at once.
sql = sql + " and ADV_REQ_TYPE = 'GROUP'";
sql = sql + " ) ELECTCREDITS";
SqlCommand cmd = new SqlCommand(sql, con);
cmd.Parameters.Add(new SqlParameter("@Parameter1", Request.QueryString["ADV_TREE_REQ_CDE"]));
cmd.Parameters.Add(new SqlParameter("@Parameter2", Request.QueryString["ADV_TREE_YR_CDE"]));
SqlDataReader Dr;
con.Open();
Dr = cmd.ExecuteReader();
try
{
while (Dr.Read())
{
lbElectCredits.Text = (string)DBUtils.GetNULLableString((Dr["ELECT_CREDITS"]));
string conc = (string)DBUtils.GetNULLableString((Dr["ELECT_CREDITS"]));
if (String.IsNullOrEmpty(conc))
divElectCredits.Visible = false;
else
divElectCredits.Visible = true;
}
}
catch (Exception ex)
{
Literal litErrorMsg = (Literal)Master.FindControl("litErrorMsg");
litErrorMsg.Text = "<span style='color:Red'>Error: " + ex.Message + "</span>";
litErrorMsg.Visible = true;
}
finally
{
if (con.State == ConnectionState.Open)
con.Close();
}
}
/* ----------------------------------------------------- Dynamic/Generated Content ----------------------------------------------------- */
/* ----------------------------------------------------- Concentration Related ----------------------------------------------------- */
protected void rptConcentrations_ItemDataBound(object sender, RepeaterItemEventArgs e)
{
RepeaterItem item = e.Item;
if ((item.ItemType == ListItemType.Item) ||
(item.ItemType == ListItemType.AlternatingItem))
{
Repeater rpt2 = (Repeater)item.FindControl("rpt2");
DataRowView drv = (DataRowView)item.DataItem;
string code = ((DataRowView)e.Item.DataItem)["concentration"].ToString();
rpt2.DataSource = ConcentrationCourses(code);
rpt2.DataBind();
Repeater rpConcentrationElective = (Repeater)item.FindControl("rpConcentrationElective");
DataRowView drv2 = (DataRowView)item.DataItem;
string code2 = ((DataRowView)e.Item.DataItem)["concentration"].ToString();
rpConcentrationElective.DataSource = electiveDS(code2);
rpConcentrationElective.DataBind();
}
}
protected DataSet ConcentrationCourses( string code) //Displays the courses that can be taken for each concentration returned by [], only being used by rpt2.
{
DataSet ds = new DataSet();
string sql = " select";
sql = sql + " ATT.REQ_TXT, CM.CRS_CDE, crs_title = ";
sql = sql + " case ";
sql = sql + " when CM2.catalog_name_full = '' OR CM2.catalog_name_full IS NULL";
sql = sql + " then CM.crs_title";
sql = sql + " else CM2.catalog_name_full";
sql = sql + " end, CM.DFLT_CREDIT_HRS";
sql = sql + " from adv_tree_table ATT";
sql = sql + " join CATALOG_MASTER CM on ATT.ADV_REQ_CODE = CM.ADV_REQ_CDE";
sql = sql + " left outer join catalog_mstr_udf CM2 on CM.crs_cde = CM2.crs_cde";
sql = sql + " where ATT.ADV_TREE_YR_CDE = @Parameter2";
sql = sql + " and ATT.ADV_TREE_REQ_CDE = @Parameter1";
sql = sql + " and ATT.PAR_ADV_REQ_CDE = @Parameter1";
sql = sql + " and (ATT.REQ_CLUSTER_CDE = 'c' or ATT.REQ_CLUSTER_CDE = 'cc')";
sql = sql + " and CM.ACTIVE_INACTIVE_ST = 'a'";
sql = sql + " and CM.INSTIT_DIV_CDE != 'GA'";
sql = sql + " order by CM.CRS_CDE";
ds = common.getDataSet("EX_ProdConnectionString", sql, new SqlParameter("@parameter1", code)
, new SqlParameter("@parameter2", Request.QueryString["ADV_TREE_YR_CDE"])
);
return ds;
}
protected void rpConcentrationElective_ItemDataBound(object sender, RepeaterItemEventArgs e)
{
RepeaterItem item = e.Item;
if ((item.ItemType == ListItemType.Item) ||
(item.ItemType == ListItemType.AlternatingItem))
{
Repeater rpConcentrationElective_course = (Repeater)item.FindControl("rpConcentrationElective_course");
DataRowView drv = (DataRowView)item.DataItem;
string ADV_TREE_REQ_CDE = ((DataRowView)e.Item.DataItem)["ADV_TREE_REQ_CDE"].ToString();
string ADV_REQ_CODE = ((DataRowView)e.Item.DataItem)["ADV_REQ_CODE"].ToString();
string sql = "";
sql = "select ";
sql = sql + " ATT.PAR_ADV_REQ_CDE, CM.CRS_CDE, ATT.ADV_REQ_DESC, CM.DFLT_CREDIT_HRS, ";
sql = sql + " CM.ACTIVE_INACTIVE_ST, CRS_TITLE =";
sql = sql + " case ";
sql = sql + " when CM2.catalog_name_full = '' OR CM2.catalog_name_full IS NULL";
sql = sql + " then CM.crs_title";
sql = sql + " else CM2.catalog_name_full";
sql = sql + " end, ATT.ADV_PTY_2";
sql = sql + " from adv_tree_table ATT";
sql = sql + " join CATALOG_MASTER CM on ATT.ADV_REQ_CODE = CM.ADV_REQ_CDE";
sql = sql + " left outer join catalog_mstr_udf CM2 on CM.crs_cde = CM2.crs_cde";
sql = sql + " where adv_tree_yr_cde = @Parameter1";
sql = sql + " and adv_tree_req_cde = @Parameter2"; //This will be the first Parameter (ADV_TREE_REQ_CDE, C-ARTED, etc.)
sql = sql + " and par_adv_req_cde = @Parameter3"; //This will be the 2nd Parameter (can be E-REQ, E-REQ 2, etc.)
sql = sql + " and CM.ACTIVE_INACTIVE_ST = 'a' ";
sql = sql + " and ATT.ADV_REQ_DESC NOT LIKE 'Old Course'";
//sql = sql + " and ADV_PTY_2 = '1'"; //This line may or may not be needed...
sql = sql + " order by CM.CRS_CDE";
DataSet ds = common.getDataSet("EX_ProdConnectionString", sql, new SqlParameter("@Parameter1", Request.QueryString["ADV_TREE_YR_CDE"]), new SqlParameter("@Parameter2", ADV_TREE_REQ_CDE),
new SqlParameter("@Parameter3", ADV_REQ_CODE));
rpConcentrationElective_course.DataSource = ds;
rpConcentrationElective_course.DataBind();
}
}
/* ----------------------------------------------------- Concentration Related End ----------------------------------------------------- */
/* ----------------------------------------------------- Elective Related ----------------------------------------------------- */
protected void RequiredElectives_ItemDataBound(object sender, RepeaterItemEventArgs e)
{
RepeaterItem item = e.Item;
if ((item.ItemType == ListItemType.Item) ||
(item.ItemType == ListItemType.AlternatingItem))
{
Repeater rpt3 = (Repeater)item.FindControl("rpt3");
DataRowView drv = (DataRowView)item.DataItem;
string code = ((DataRowView)e.Item.DataItem)["ADV_REQ_CODE"].ToString();
string code2 = ((DataRowView)e.Item.DataItem)["GRP_REL_TYP"].ToString();
string code3 = ((DataRowView)e.Item.DataItem)["BLANK_LINES"].ToString();
rpt3.DataSource = ElectiveCourses(code, code2, code3);
rpt3.DataBind();
}
if ((item.ItemType == ListItemType.Item) ||
(item.ItemType == ListItemType.AlternatingItem))
{
Repeater blocklist3 = (Repeater)item.FindControl("blocklist3");
DataRowView drv = (DataRowView)item.DataItem;
string code = ((DataRowView)e.Item.DataItem)["ADV_REQ_CODE"].ToString();
string code2 = ((DataRowView)e.Item.DataItem)["GRP_REL_TYP"].ToString();
string code3 = ((DataRowView)e.Item.DataItem)["BLANK_LINES"].ToString();
blocklist3.DataSource = ElectiveCoursesBlocklist(code, code2, code3);
blocklist3.DataBind();
}
}
protected DataSet electiveDS(string code) //used by rpConcentrationElective to display the Elective groups for each Concentration
{
DataSet ds = new DataSet();
string sql;
sql = "select ";
sql = sql + " ADV_TREE_REQ_CDE , ADV_REQ_CODE, ADV_REQ_TYPE, ";
sql = sql + " MIN_HRS, ADV_REQ_DESC ";
sql = sql + " ,REQ_TXT = ";
sql = sql + " case ";
sql = sql + " when REQ_TXT like '%~%' ";
sql = sql + " then LEFT(REQ_TXT,CHARINDEX('~',REQ_TXT)-1) ";
sql = sql + " else REQ_TXT ";
sql = sql + " end, ";
sql = sql + " (ADV_PTY_2 + 1) as level ";
sql = sql + " from ADV_TREE_TABLE ";
sql = sql + " where ADV_TREE_REQ_CDE = @Parameter1 ";
sql = sql + " and adv_tree_yr_cde = @Parameter2 ";
sql = sql + " and (PAR_ADV_REQ_CDE = @Parameter1 or PAR_ADV_REQ_CDE LIKE '%req%') ";
//sql = sql + " and ADV_PTY_2 = '1' "; //temporarily removed, attempt to display all sub-electives at once.
sql = sql + " and ADV_REQ_TYPE = 'GROUP' ";
sql = sql + " order by REQ_TXT ";
return ds = common.getDataSet("EX_ProdConnectionString", sql, new SqlParameter("@parameter1", code)
, new SqlParameter("@parameter2", Request.QueryString["ADV_TREE_YR_CDE"])
);
}
protected DataSet ElectiveCourses(string ADV_REQ_CODE, string GRP_REL_TYP, string BLANK_LINES )
//Displays the list of courses that can be taken for each elective returned by [?], only being used by rpt3.
{
if( GRP_REL_TYP.ToString().ToLower() == "or" )
{
// Set the list of available courses in block format and display to user
// Use the same query as you would to display the courses, just present in block format
// Attach to new div and make visible
// This will be done using ElectiveCoursesBlocklist, which gets called
// right after ElectiveCourses above, in RequiredElectives_ItemDataBound
// Display blank lines for user to fill in manually
// This will be attached to rtp3
DataSet ds_blanklines = new DataSet();
string sql2 = " select";
sql2 = sql2 + " top " + BLANK_LINES ;
sql2 = sql2 + " ' ' as CRS_CDE, ' ' as CRS_TITLE, ' ' as DFLT_CREDIT_HRS, ' ' as REQ_TXT";
sql2 = sql2 + " from adv_tree_table ATT ";
ds_blanklines = common.getDataSet("EX_ProdConnectionString", sql2);
return ds_blanklines;
//Also display blocklist3
}
else // when GRP_REL_TYP = AND
{
DataSet ds = new DataSet();
//Run the original query and list out the courses on their own rows
string sql = " select";
sql = sql + " ATT.PAR_ADV_REQ_CDE, ATT.REQ_TXT, CM.CRS_CDE, ATT.ADV_REQ_DESC, ";
sql = sql + " CM.DFLT_CREDIT_HRS, CM.ACTIVE_INACTIVE_ST, CRS_TITLE =";
sql = sql + " case ";
sql = sql + " when CM2.catalog_name_full = '' OR CM2.catalog_name_full IS NULL";
sql = sql + " then CM.crs_title";
sql = sql + " else CM2.catalog_name_full";
sql = sql + " end, ATT.ADV_PTY_2";
sql = sql + " from adv_tree_table ATT";
sql = sql + " join CATALOG_MASTER CM on ATT.ADV_REQ_CODE = CM.ADV_REQ_CDE";
sql = sql + " left outer join catalog_mstr_udf CM2 on CM.crs_cde = CM2.crs_cde";
sql = sql + " where adv_tree_yr_cde = @parameter1";
sql = sql + " and adv_tree_req_cde = @parameter2"; //This will be the first Parameter (ADV_TREE_REQ_CDE, C-ARTED, etc.)
sql = sql + " and par_adv_req_cde = @parameter3"; //This will be the 2nd Parameter (can be E-REQ, E-REQ 2, etc.)
sql = sql + " and CM.ACTIVE_INACTIVE_ST = 'a' ";
sql = sql + " and ATT.ADV_REQ_DESC NOT LIKE 'Old Course'";
//sql = sql + " and ADV_PTY_2 = '1'"; //This line may or may not be needed...
sql = sql + " order by CM.CRS_CDE";
ds = common.getDataSet("EX_ProdConnectionString", sql,
new SqlParameter("@parameter1", Request.QueryString["ADV_TREE_YR_CDE"]),
new SqlParameter("@parameter2", Request.QueryString["ADV_TREE_REQ_CDE"]),
new SqlParameter("@parameter3", ADV_REQ_CODE)
);
return ds;
//if (ds.Tables[0].Rows.Count > 0)
//divBlocklist3.Visible = false;
}
}
protected DataSet ElectiveCoursesBlocklist(string ADV_REQ_CODE, string GRP_REL_TYP, string BLANK_LINES )
//Displays the list of courses that can be taken for each elective returned by [?], only being used by blocklist3.
{
DataSet ds = new DataSet();
//Run the original query and list out the courses
string sql = " select";
sql = sql + " ATT.PAR_ADV_REQ_CDE, ATT.REQ_TXT, CM.CRS_CDE, ATT.ADV_REQ_DESC, ";
sql = sql + " CM.DFLT_CREDIT_HRS, CM.ACTIVE_INACTIVE_ST, CRS_TITLE =";
sql = sql + " case ";
sql = sql + " when CM2.catalog_name_full = '' OR CM2.catalog_name_full IS NULL";
sql = sql + " then CM.crs_title";
sql = sql + " else CM2.catalog_name_full";
sql = sql + " end, ATT.ADV_PTY_2";
sql = sql + " from adv_tree_table ATT";
sql = sql + " join CATALOG_MASTER CM on ATT.ADV_REQ_CODE = CM.ADV_REQ_CDE";
sql = sql + " left outer join catalog_mstr_udf CM2 on CM.crs_cde = CM2.crs_cde";
sql = sql + " where adv_tree_yr_cde = @parameter1";
sql = sql + " and adv_tree_req_cde = @parameter2"; //This will be the first Parameter (ADV_TREE_REQ_CDE, C-ARTED, etc.)
sql = sql + " and par_adv_req_cde = @parameter3"; //This will be the 2nd Parameter (can be E-REQ, E-REQ 2, etc.)
sql = sql + " and CM.ACTIVE_INACTIVE_ST = 'a' ";
sql = sql + " and ATT.ADV_REQ_DESC NOT LIKE 'Old Course'";
//sql = sql + " and ADV_PTY_2 = '1'"; //This line may or may not be needed...
sql = sql + " order by CM.CRS_CDE";
ds = common.getDataSet("EX_ProdConnectionString", sql,
new SqlParameter("@parameter1", Request.QueryString["ADV_TREE_YR_CDE"]),
new SqlParameter("@parameter2", Request.QueryString["ADV_TREE_REQ_CDE"]),
new SqlParameter("@parameter3", ADV_REQ_CODE)
);
//blocklist3.DataSource = ds;//--Here
//blocklist3.DataBind();
//if (ds.Tables[0].Rows.Count > 0)
//divBlocklist3.Visible = false;
return ds;
}
/* ----------------------------------------------------- Elective Related End ----------------------------------------------------- */
}
1 Answer

James Churchill
Treehouse TeacherWilliam,
You're correct in your assumption that the divBlocklist3
control can't be referred directly in code because it's contained within a repeater item template. Here are a couple of workarounds that you might try:
1) Instead of trying to set the Visible
property via code, use binding.
<div id="divBlocklist3" runat="server" Visible='<%# Eval("???") %>'>
Using this approach would require you to add a calculated boolean column (I used ???
as a placeholder for the calculated column name) to the data table that you're binding. You could do that either by writing the necessary SQL or by manipulating the data table via code.
2) You can use the Repeater control's ItemDataBound
event to get a reference to the control in the item template. To prepare to do this, I'd change the <div>
element to a Panel control.
<asp:Panel id="pnlBlocklist3" runat="server" Visible="true">
And here's how to find the control in the item template:
protected void ItemDataBound(object sender, RepeaterItemEventArgs e)
{
if (e.Item.ItemType == ListItemType.Item || e.Item.ItemType == ListItemType.AlternatingItem)
{
var control = e.Item.FindControl("pnlBlocklist3") as Panel;
if (control != null)
{
// add your logic to determine the visibility of the Panel control
control.Visible = false;
}
}
}
I hope this helps!
Thanks ~James
William J. Terrell
17,403 PointsWilliam J. Terrell
17,403 PointsThanks, James!
Ultimately, we just decided to chop this bit and just list out all the courses. That's just as well, since I think this page is a bit of a technical monster as it is. :P
Thanks!