Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial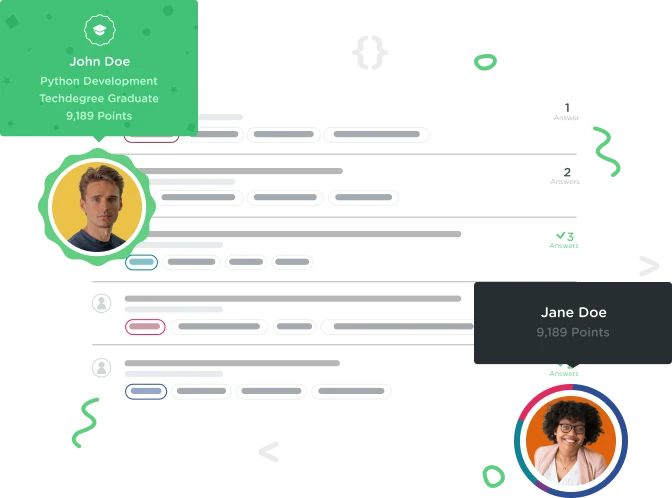
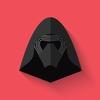
Stu Cowley
26,287 PointsHelp with PHP Array
Hey guys, I'm current building a site for my wife's beauty salon and I am building out the treatments page and I decided I would do the treatment pages like the Shirts4Mike shirts array. I want to have an array for each treatment (waxing, massage, etc). I have been able to build the waxing page but the way I am outputting it seems a bit weird as I can only manage to output all treatments, instead of outputting specific treatments for its correct page.
Here's the code I have done thus far.
(treatment-guide.php)*
<?php
function get_table_view_html($treatment_id, $treatment) {
$output = "";
$output = $output . "<tr>";
$output = $output . "<td>";
$output = $output . $treatment["name"];
$output = $output . "</td>";
$output = $output . '<td class="text-right">';
$output = $output . "$";
$output = $output . $treatment["price"];
$output = $output . "</td>";
$output = $output . "</tr>";
return $output;
}
$treatment = array();
$treatment[101] = array(
"name" => "Eyebrow",
"price" => 15
);
$treatment[102] = array(
"name" => "Lip",
"price" => 8
);
$treatment[103] = array(
"name" => "Lip Bleach",
"price" => 12
);
$treatment[104] = array(
"name" => "Chin",
"price" => 8
);
$treatment[105] = array(
"name" => "Eyebrow, Lip & Chin",
"price" => 30
);
$treatment[106] = array(
"name" => "Sides of Face",
"price" => 10
);
$treatment[107] = array(
"name" => "Underarms",
"price" => 12
);
$treatment[108] = array(
"name" => "Bikini Line",
"price" => 15
);
$treatment[109] = array(
"name" => "Half Arms",
"price" => 25
);
$treatment[110] = array(
"name" => "Full Arms",
"price" => 30
);
$treatment[111] = array(
"name" => "Half Legs",
"price" => 30
);
$treatment[112] = array(
"name" => "Half Legs & Back of Thighs",
"price" => 35
);
$treatment[113] = array(
"name" => "Full Legs",
"price" => 40
);
$treatment[114] = array(
"name" => "Full Legs & Bikini Line",
"price" => 55
);
$treatment[115] = array(
"name" => "Back (and Shoulders)",
"price" => 30
);
$treatment[116] = array(
"name" => "Chest (and Stomach)",
"price" =>30
);
$treatment[117] = array(
"name" => "Full Legs",
"price" => 50
);
$treatment[118] = array(
"name" => "Full Arms",
"price" => 40
);
?>
(waxing.php)
<?php
$pageTitle = "Treatments | Loveday Spa";
include('inc/header.php');
?>
<div class="jumbotron text-center">
<div class="container">
<h1>Treatments</h1>
</div>
</div>
<div class="container">
<div class="row">
<div class="col-sm-4">
<ul class="nav nav-pills nav-stacked">
<li class="active"><a href="#">Waxing</a></li>
<li><a href="lash-brow.php">Lash & Brow Treatments</a></li>
<li><a href="nails.php">Nail Treatments</a></li>
<li><a href="sunless-tanning.php">Sunless Tanning</a></li>
<li><a href="massage.php">Massage</a></li>
<li><a href="facial.php">Facial Treatments</a></li>
<li><a href="body.php">Body Treatments</a></li>
</ul>
</div>
<div class="col-sm-8">
<?php include('inc/treatments.php'); ?>
<table class="table treatments">
<?php foreach ($treatment as $treatment_id => $treatment) {
echo get_table_view_html($treatment_id, $treatment);
}
?>
<!-- ## Issue 001; Imports every $product in the array. -->
<!-- ## Issue 002; There needs to be a heading for "For Men". -->
</table>
</div>
</div>
</div>
<?php include('inc/footer.php'); ?>
So basically I want to do the same for each treatment, but I can't get my head around how I can do it correctly.
I know I'm not making any sense with this, but I'm sure there's someone out there that knows what I'm talking about.
Cheers!
Stu : )
2 Answers
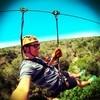
thomascawthorn
22,986 PointsHey Stu!
Am I right in thinking that the array of treatments covers many things from waxing + massage etc?
You will need to refactor your array, making it one level deeper. ps [] is the shorthand for array();
<?php
$treatmentTypes = [
'waxing' => [
'101' => [
'name' => 'Some Treatment',
'price' => 40.00,
]
'102' => [
'name' => 'Some Treatment',
'price' => 40.00,
]
'103' => [
'name' => 'Some Treatment',
'price' => 40.00,
]
]
'massage' => [
'201' => [
'name' => 'Some Treatment',
'price' => 40.00,
'202' => [
'name' => 'Some Treatment',
'price' => 40.00,
'203' => [
'name' => 'Some Treatment',
'price' => 40.00,
]
]
];
See how you're grouping all the treatments by category? You will need to refactor some of your loops if you ever wanted to display everything:
<?php
foreach($treatmentTypes as $treatmentType => $treatments) {
foreach($treatments as $treatment) {
// $treatment would contain name and price.
}
}
If you wanted to show the treatments for one treatmentType:
<?php
foreach($treatmentTypes['waxing'] as $treatment) {
// $treatment would contain name and price.
}
Hope this helps!
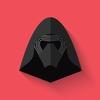
Stu Cowley
26,287 PointsHey Tom,
That makes sense, I'll have a go with it now!
Stu : )
thomascawthorn
22,986 Pointsthomascawthorn
22,986 PointsPS if you wanted to assign the values in a similar way, you just need to do this: