Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial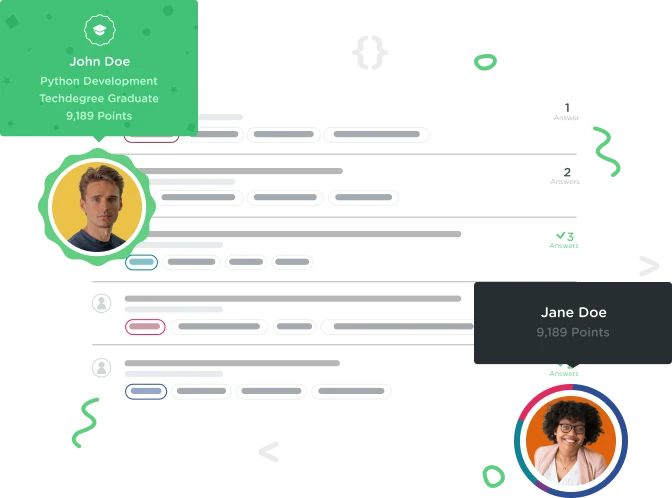
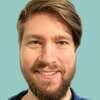
David Kayser
12,009 PointsHere's my try at adding a reset button and keeping score for the object oriented javascript challenge.
Gave a shot at adding a reset button and a score system.
First I added a score element and reset button to the index.html file. In Game.js I've added a method called resetGame():
/**
* Resets the game.
*/
resetGame(){
//Remove gameOver message
document.getElementById('game-over').style.display = 'none';
document.getElementById('game-over').textContent = '';
//Reset player order
this.players[0].active = true;
this.players[1].active = false;
//Remove all htmlTokens
Array.from(document.querySelectorAll('.token')).forEach((el) => el.parentNode.removeChild(el));
//Resets the play space
for (let column of this.board.spaces){
for (let space of column){
space.token = null;
}
}
//Reset played tokens
for (let player of this.players){
for (let token of player.tokens){
token.columnLocation = 0;
token.dropped = false;
}
}
//Start a new game
this.startGame()
}
and a method called updateHTMLScore()
/**
* Updates the score.
*/
updateHTMLScore(){
const scoreCard = document.getElementById(`player-${this.activePlayer.id}-score`);
scoreCard.textContent = `Player ${this.activePlayer.id}: ${this.activePlayer.score}`;
}
I've also added a click event listener in app.js:
/**
* Listen for click on `#play-again` and calls resetGame() on game object
*/
document.getElementById('play-again').addEventListener('click', function() {
game.resetGame();
});
Daoud Merchant
5,135 PointsDaoud Merchant
5,135 PointsI appreciate that you put a lot of effort in to the
resetGame()
method, but I think it would save you a lot of trouble to just instantiate a whole new game:Game
instantiatesBoard
andPlayer
,Board
instantiatesSpace
andPlayer
instantiatesToken
, so everything will be reinstantiated in a cascading fashion.Another teeny tip: if you ever write a function which takes no arguments and the only thing it does is call a different function which takes no arguments, you can just pass the second function directly. Your last line of code could be shortened to:
document.getElementById('play-again').addEventListener('click', game.resetGame);
Good luck!