Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial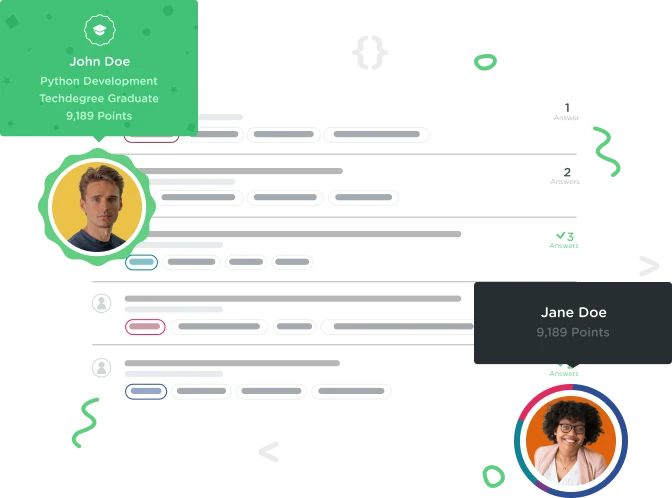
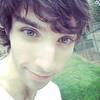
Alphonse Cuccurullo
2,513 PointsHey just confused at a few parts of this syntax.
# method that capitalizes a word
def capitalize(string)
puts "#{string[0].upcase}#{string[1..-1]}"
end
capitalize("ryan") # prints "Ryan"
capitalize("jane") # prints "Jane"
# block that capitalizes each string in the array
["ryan", "jane"].each {|string| puts "#{string[0].upcase}#{string[1..-1]}"} # prints "Ryan", then "Jane"
So the question i have here is what is with the second part of the of the puts method with
#{string(1.. - 1]}
" What is that doing exactly? Also is the each method using part of the capatilize method?
1 Answer
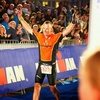
Steve Hunter
57,712 PointsHi Alphonse,
This is using string interpolation in two ways. First, it is taking the first letter of the string and making it a capital. This is then inserted into the output string. Ruby does this with #{var}
- that puts the contents of the variable var
into the string like "The variable contains #{var}
". I hope that's clear!
The next part of the output string is then being inserted in the same way. This is starting at position 1 of the array (the second letter) and then it goes to the end of the array, the -1 value. So, the output string is made up of two interpolated strings. The first is the capitalised first letter, then the rest of the array from the second letter to the end.
"#{string[0].upcase}#{string[1..-1]}"
#first interpolation:
"#{string[0].upcase}" # "R"
#second interpolation:
"#{string[1..-1]}" # "yan"
You could do this separately:
firstLetter = string[0].upcase
restOfWord = string[1..-1]
puts "#{firstLetter}#{restOfWord}"
The contents of #{}
are added to the string at the position entered. Make more sense?
Steve.