Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial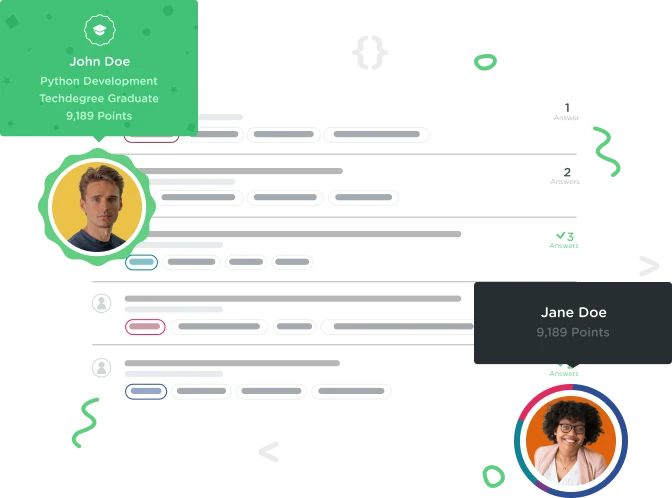

olamilekan oshodi
959 PointsHi guy am getting error on OOP.
//////////////////My first error
is when i tried to open this below code on browser is giving me error on line 31
<?php
// class
class Fish {
//property
public $common_name = common_name;
public $flavor = flavor;
public $record_weight = record_weight;
//construct method
function __construct($name, $flavor, $record){
//assign each of the properties on the Fish class with its corresponding parameter variable
$this->common_name = $name;
$this->flavor = $flavor;
$this->record_weight = $record;
}
// Create a method on Fish named getInfo that takes no parameters
public function getInfo(){
//returns a string that includes the common_name, flavor, and record_weight for the fish
// When called on $bass, getInfo might return "string below"
return "A $this->common_name is an $this->flavor flavored fish. The world record weight is $this->record_weight";
}
}
// create a Fish object name $bass
// Object is defined after the closen fish class tag
// A new object start with e.g $bass = new Fish (....);
$bass = new Fish ("Largemouth Bass", "Excellent", "22 pounds 5 ounces");
?>
///////////////////////////Second error
<?php
////// Object inheritant ////
public $name = 'default_name';
public $price = '0';
public $desc = 'default_description';
function __construct($name, $price, $desc){
$this->name = $name;
$this->price = $price;
$this->desc = $desc;
}
public function getInfo(){
return "Product Name: ". $this->name;
}
}
class Soda extends Product
{
public $flavor;
function __construct($name, $price, $desc, $flavor){
parent::__construct($name, $price, $desc);
$this->flavor = $flavor;
}
public function getInfo(){
return "Product Name: " . $this->name . " Flavor: " . $this->flavor;
}
$shirt = new Product("Space Juice T-shirt", 20, "Awesome Grey T-shirt");
$soda = new Soda("Space Juice Soda", "5", "Thirst Mutilator", "Grape");
echo $soda->getInfo();
?>
am getting this error below
Parse error: syntax error, unexpected '$soda' (T_VARIABLE), expecting function (T_FUNCTION) in /home/treehouse/workspace/index.php on line 57
please help
5 Answers
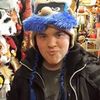
Codin - Codesmite
8,600 PointsThere is some mistakes in your syntax I have corrected them below :)
<?php
// class
class Fish {
//property
public $common_name;
public $flavor;
public $record_weight;
//construct method
function __construct($name, $flavor, $record){
//assign each of the properties on the Fish class with its corresponding parameter variable
$this->common_name = $name;
$this->flavor = $flavor;
$this->record_weight = $record;
}
// Create a method on Fish named getInfo that takes no parameters
public function getInfo(){
//returns a string that includes the common_name, flavor, and record_weight for the fish
// When called on $bass, getInfo might return "string below"
return "A $this->common_name is an $this->flavor flavored fish. The world record weight is $this->record_weight";
}
}
// create a Fish object name $bass
// Object is defined after the closen fish class tag
// A new object start with e.g $bass = new Fish (....);
$bass = new Fish("Largemouth Bass", "Excellent", "22 pounds 5 ounces");
echo $bass->getInfo();
?>
The first block of code was throwing an error from how you defined your public variables.
and
<?php
class Product {
public $name = 'default_name';
public $price = '0';
public $desc = 'default_description';
function __construct($name, $price, $desc) {
$this->name = $name;
$this->price = $price;
$this->desc = $desc;
}
public function getInfo() {
return "Product Name: ". $this->name;
}
}
class Soda extends Product {
public $flavor;
function __construct($name, $price, $desc, $flavor){
parent::__construct($name, $price, $desc);
$this->flavor = $flavor;
}
public function getInfo() {
return "Product Name: " . $this->name . " Flavor: " . $this->flavor;
}
}
$shirt = new Product("Space Juice T-shirt", 20, "Awesome Grey T-shirt");
$soda = new Soda("Space Juice Soda", "5", "Thirst Mutilator", "Grape");
echo $soda->getInfo();
?>
You were missing a couple of }.

olamilekan oshodi
959 Pointssorry guy's the first code did come out great.
<?php
// class
class Fish {
//property
public $common_name = common_name;
public $flavor = flavor;
public $record_weight = record_weight;
//construct method
function __construct($name, $flavor, $record){
$this->common_name = $name;
$this->flavor = $flavor;
$this->record_weight = $record;
}
public function getInfo(){
return "A $this->common_name is an $this->flavor flavored fish. The world record weight is $this->record_weight";
}
}
$bass = new Fish ("Largemouth Bass", "Excellent", "22 pounds 5 ounces");
echo $bass;
?>

olamilekan oshodi
959 PointsAshley Skilton thank you so much. little mistake can frustrate at time.
one more help can you take a look with the same code as above but here am testing the "static method and property".
<?php
////// Object inheritant ////
class Product {
public static $manufacturer = "lekan oshodi"; // static method and property
public $name = 'default_name';
public $price = '0';
public $desc = 'default_description';
function __construct($name, $price, $desc){
$this->name = $name;
$this->price = $price;
$this->desc = $desc;
}
public function getInfo(){
return "Product Name: ". $this->name;
}
// static method
public funtion getMaker(){
return self::$manufacturer;
}
// end static method
}
class Soda extends Product
{
public $flavor;
function __construct($name, $price, $desc, $flavor){
parent::__construct($name, $price, $desc);
$this->flavor = $flavor;
}
public function getInfo(){
return "Product Name: " . $this->name . " Flavor: " . $this->flavor;
}
}
$shirt = new Product("Space Juice T-shirt", 20, "Awesome Grey T-shirt");
$soda = new Soda("Space Juice Soda", "5", "Thirst Mutilator", "Grape");
//echo $soda->getInfo();
echo $shirt->getMaker();
?>
but am getting this error.
Parse error: syntax error, unexpected 'funtion' (T_STRING), expecting variable (T_VARIABLE) in /home/treehouse/workspace/index.php on line 29
also what is wrong with this code below.
<?php
class Person {
/**
* @var $firstName string
*/
public $firstName = 'John';
/**
* @var $lastName string
*/
public $lastName = 'Smith';
/**
* @var $age integer
*/
public $age = 1040;
/**
* @var $phoneNumber integer
*/
public $phoneNumber = 01234123456;
/**
* The Persons full name
*
* @return $fullName string
*/
public function fullName()
{
return $this->firstName .' '. $this->lastName;
}
}
echo fullName;
?>
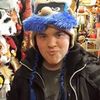
Codin - Codesmite
8,600 PointsWhile I take a look at your code check out this post on how to post code blocks to the forum (I've edited your posts for you but its useful to know :) ): https://teamtreehouse.com/community/posting-code-to-the-forum
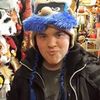
Codin - Codesmite
8,600 PointsLine 21 in the first code block has a typo on function (missing a c) "public funtion getMaker(){"
Second codeblock's variables and function need to be static like this:
<?php
class Person {
/**
* @var $firstName string
*/
public static $firstName = 'John';
/**
* @var $lastName string
*/
public static $lastName = 'Smith';
/**
* @var $age integer
*/
public $age = 1040;
/**
* @var $phoneNumber integer
*/
public $phoneNumber = 01234123456;
/**
* The Persons full name
*
* @return $fullName string
*/
public static function fullName()
{
return self::$firstName .' '. self::$lastName;
}
}
echo Person::fullName();
?>
Use $this to refer to the current object. Use self to refer to the current class. In other words, use $this->member for non-static members, use self::$member for static members.

olamilekan oshodi
959 Pointsthanks for that, now i know. to test it. do you manage to check my code? here it is again.
<?php
class Person {
/**
* @var $firstName string
*/
public $firstName = 'John';
/**
* @var $lastName string
*/
public $lastName = 'Smith';
/**
* @var $age integer
*/
public $age = 1040;
/**
* @var $phoneNumber integer
*/
public $phoneNumber = 01234123456;
/**
* The Persons full name
*
* @return $fullName string
*/
public function fullName()
{
return $this->firstName .' '. $this->lastName;
}
}
echo fullName;
?>

olamilekan oshodi
959 Pointsthanks mate. really appreciate it.
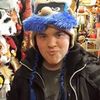
Codin - Codesmite
8,600 PointsNo problem, glad I could help :)