Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial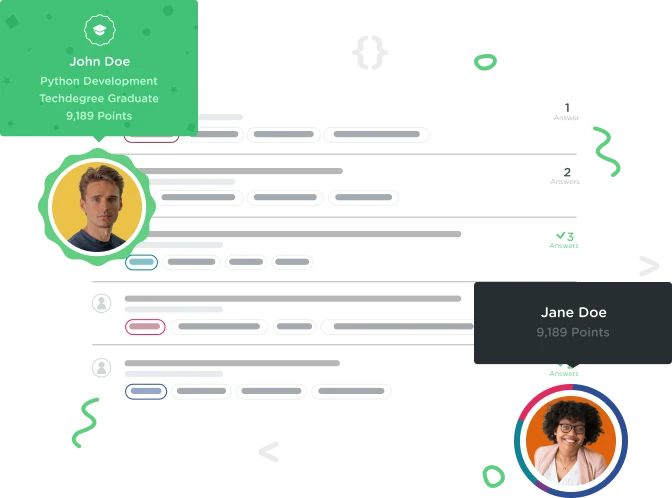

Aaron Banerjee
2,509 PointsHi I need help with Ruby
Why do you sometimes use && and then ||
Jason made us write this code in the last project for the 3rd badge in ruby, answer these questions
why does he write the code below name = get_name() greet(name) number = get_number()
also here
def get_name() print "Enter your name: " return gets.chomp end
he does not write name in the parentheses but the code interprets it in def greet(name) which I don't understand
def get_name() print "Enter your name: " return gets.chomp end
def greet(name) print "Hi #{name}!" if (name == "Jason") puts "That's a great name!" end end
def get_number(number) print "What number would you like to test to see if it is divisible by 3? " return gets.chomp.to_i end
def divisible_by_3?(number) return (number % 3 == 0) end
name = get_name() greet(name) number = get_number()
if divisible_by_3?(number) puts "Your number is divisible by 3!" end

Aaron Banerjee
2,509 Pointshey Burke, I really like your answer, but could you please explain the number = get_number(), I also want to give you the best answer, so please tell me how in the post
2 Answers

Burke Fitzpatrick
Python Web Development Techdegree Student 17,148 PointsHi Aaron,
Functions can take variables and return variables. So when a variable is in the parenthesis it means the function takes input. When the function uses the return keyword, it means it returns output. Functions can also take nothing and return nothing.
I'm sorry about the syntax. I'm not sure how to highlight the code on here.
So a basic function would be something like:
def hello() print "Hello" end
which doesn't take any input and it doesn't return anything, it just prints out "Hello". You would call it by typing hello()
A more advanced function would take a name as input:
def hello(name) print "Hello {name}" end
Which would be called by typing hello("bob")
The next thing you could do is make a function that doesn't print anything, but it does return a greeting for some other code to use.
def hello(name) return "Hello {name}" end
if the function has a return like that, you can assign the return value to a variable:
greeting = hello("bob") print greeting
This means greeting now has the value 'Hello bob" and that would be printed out. You can also shorten this up by writing print hello("bob") since the print statement will print out the value returned by the hello function.

Guillaume Maka
Courses Plus Student 10,224 Points1) Why do you sometimes use && and then ||
From Burke Fitzpatrick answer
&& and || are tests that return true or false.
The && is a logical AND. It means if variable1 is true AND variable2 is true, return true. Otherwise it will return false.
The || is a logical OR. It means if variable1 is true OR variable2 is true, return true. Otherwise (both are false) return false.
You can chain them together to make longer expressions. If (variable1 && variable2) || (variable3 && variable4) do something.
2) Jason made us write this code in the last project for the 3rd badge in ruby, answer these questions
def get_name()
print "Enter your name: " return gets.chomp
end
can be rewrite
def get_name()
print "Enter your name: "
name = gets.chomp
end
get_name() is a method to prompt the user to enter his name and return it. No need to pass a name variable to the method because in this case the method is responsible of getting something from the user and doesn't need to manipulate/compute any variable.
then
def get_number(number)
print "What number would you like to test to see if it is divisible by 3? " return gets.chomp.to_i
end
the "number" variable is not need because its never use like get_name() the method is responsible to get a number from the user.
# Ask the user for his name an assign the return value to the name variable
name = get_name()
# print the name
greet(name)
# Ask the user for a number an assign the return value to the number variable
number = get_number()
# Check if number is divisible by 3
# divisible_by_3?(number) return true or false
if divisible_by_3?(number)
puts "Your number is divisible by 3!"
end
Burke Fitzpatrick
Python Web Development Techdegree Student 17,148 PointsBurke Fitzpatrick
Python Web Development Techdegree Student 17,148 PointsI'll try to help...
&& and || are tests that return true or false.
The && is a logical AND. It means if variable1 is true AND variable2 is true, return true. Otherwise it will return false.
The || is a logical OR. It means if variable1 is true OR variable2 is true, return true. Otherwise (both are false) return false.
You can chain them together to make longer expressions. If (variable1 && variable2) || (variable3 && variable4) do something.
As for the function definitions, the first function doesn't take a value but it does return one. The code is getting a value from the user and returning it in one line, which can be a little confusing. it could be written as name = gets.chomp and then return name.
The second function takes a value called name and uses it. Functions can be defined with or without values, depending on what they do.
As for the rest, I'm not sure what your question is. Functions can be 'daisy chained' so that if one function returns a value it can be used as input into another function, but I'm not really sure what your question is.