Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial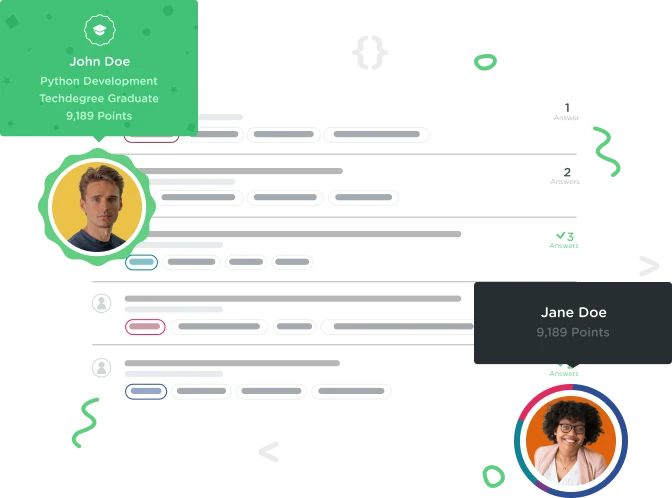

Unsubscribed User
6,269 PointsHi, problem with my moving square app
Hi, I'm trying to make an app that moves one square from one corner to another after clicking on it (in order: top left -> top right -> bottom right -> bottom left). Here is my HTML and CSS:
<!DOCTYPE html>
<html lang="en" dir="ltr">
<head>
<link rel="stylesheet" href="basic.css">
<meta charset="utf-8">
<title></title>
</head>
<body>
<div class="container">
<div class="block"></div>
</div>
<script src="basic.js"></script>
</body>
</html>
* {
margin: 0;
}
body {
display: flex;
align-items: center;
justify-content: center;
min-height: 100vh;
}
.container {
height: 500px;
width: 500px;
border: solid 1px;
position: relative;
}
.block {
height: 100px;
width: 100px;
position: absolute;
background: red;
transition-duration: 1s;
}
In JavaScript I add event listener for this square object. It moves it immediately from the top left corner to bottom right corner, not from top left to top right and then to bottom right. Why is it happening? Here is JavaScript code:
const block = document.querySelector(".block");
block.style.top = "0px";
block.style.left = "0px";
block.addEventListener('click', () => {
if ( block.style.top == "0px" && block.style.left == "0px" ) {
block.style.left = "400px";
}
if ( block.style.top == "0px" && block.style.left == "400px" ) {
block.style.top = "400px";
}
});
1 Answer

KRIS NIKOLAISEN
54,971 PointsYou have two if statements that are evaluated as true and the move is executed. The first one moves the block left to 400 at which point the second is true. To avoid this use else if
.
block.addEventListener('click', () => {
if ( block.style.top == "0px" && block.style.left == "0px" ) {
block.style.left = "400px";
}
else if ( block.style.top == "0px" && block.style.left == "400px" ) {
block.style.top = "400px";
}
});
Unsubscribed User
6,269 PointsUnsubscribed User
6,269 PointsThanks ;)