Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial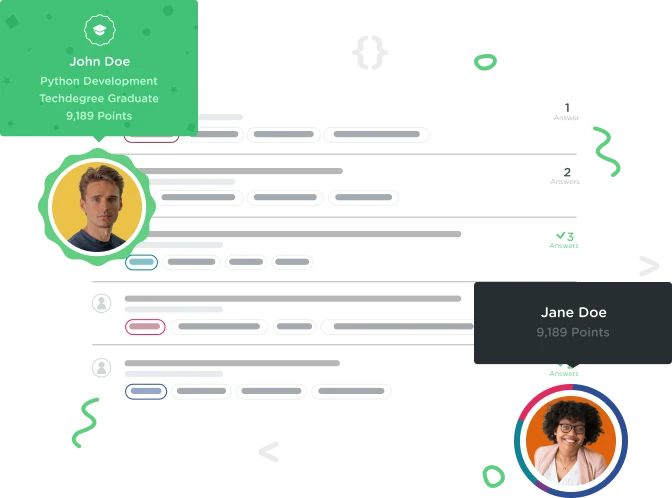
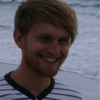
Daniel Schwemmlein
4,960 PointsHow can I add a new product instead o '1' product to the cart in this challenge
Hey there.
there is a new java script challenge and the question is to change the code from adding '1' new product to the shopping cart to adding a 'new' product to the cart. It seems quite easy but really need a hint. thanks in advance!
public class Example {
public static void main(String[] args) {
ShoppingCart cart = new ShoppingCart();
Product pez = new Product("Cherry PEZ refill (12 pieces)");
cart.addItem(pez, 5);
/* Since a quantity of 1 is such a common argument when adding a product to the cart,
* your fellow developers have asked you to make the following code work, as well as keeping
* the ability to add a product and a quantity.
*/
Product dispenser = new Product("Yoda PEZ dispenser");
/* Uncomment the line following this comment,
after adding a new method using method signatures,
to solve their request in ShoppingCart.java
*/
cart.addItem(dispenser);
}
}
public class ShoppingCart {
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity. Please imagine
lots and lots of code here. Don't repeat it.
*/
}
}
public class Product {
/* Other code omitted for clarity, but you could imagine
it would store price, options like size and color
*/
private String mName;
public Product(String name) {
mName = name;
}
public String getName() {
return mName;
}
}
3 Answers
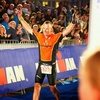
Steve Hunter
57,712 PointsHi Daniel,
There are two things to do in this challenge. The outcome is that you have the ability to use the existing functionality within the addItem
method to add an item
and a quantity
. For ease of use, however, there is requirement that says if the quantity
is omitted, then that is defaulted to 1; i.e. buying a single item.
So, if you want to buy two buckets, you call;
addItem(aBucket, 2);
But if you just want to buy one item, you can omit the integer:
addItem(aBucket);
This means you are calling the same method but passing in different numbers of parameters. All the functionality is contained in the existing implementation of addItem
- as the code says, there's loads omitted for clarity. We really don't want to duplicate any of that for reasons of DRY, maintenance etc.
We do need to stipulate a second method, called addItem
that will just add a single item
to the cart.
The new method signature looks like:
public void addItem(Product item)
That takes the single parameter. So now we just have to make it add one of item
to the cart. To do this we can call the initial method from within the new one:
public void addItem(Product item) {
addItem(item, 1);
}
That calls the function that takes two parameters and requests that item
is added to the cart 1 time.
Your code deletes the existing method - that's not what we want here. This is an additional requirement, not a replacement. But by using the existing code we can acheive the aims of the task.
In essence, we're shortening this:
cart.addItem(aBucket, 1);
... to this ...
cart.addItem(aBucket);
... but we're learning that we can have more than one method called the same thing but taking different parameters. And we're also adhering to DRY principles and reusing existing code wherever we can.
I do hope that makes sense - I have tested the code in the challenge and it does work.
I mentioned two steps. First, add the overridden method in SHoppingKart:
public class ShoppingCart {
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity. Please imagine
lots and lots of code here. Don't repeat it.
*/
}
public void addItem(Product item) {
addItem(item, 1);
}
}
Then uncomment the last line in Example
:
cart.addItem(dispenser);
That should get you through it.
Steve.
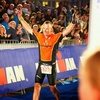
Steve Hunter
57,712 PointsHi Daniel,
I think you can use the exiting method within your new one. The challenge wants you to be able to just add one item to the cart only if no item number is passed into the method. The new method call that you are asked to uncomment only has an item
parameter, not a quantity
one too.
So, your new method only takes one parameter, Product item
. Inside that method, you then call the exsting addItem
method but defaulting the second, int quantity
parameter to 1
.
That's a hint, rather than an answer - I hope it helps you. If not - shout back!
Steve.
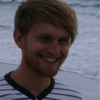
Daniel Schwemmlein
4,960 PointsHi Steve,
now I have this: I'm not sure anymore if that was how you set something to 1 by default. It can't be that easy. There is probably something else I have to put. Maybe a boolean? Please help me out here thanks
public class ShoppingCart {
public void addItem(Product item, int quantity =! 1) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
}
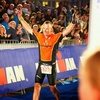
Steve Hunter
57,712 PointsHi Daniel,
You need to override the method which entails writing it out again but with different parameter requirements. Something like:
public class ShoppingCart {
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity. Please imagine
lots and lots of code here. Don't repeat it.
*/
}
public void addItem(Product item) {
addItem(item, 1);
}
}
So, the second method only takes one parameter but within it, the method calls the original one with a quantity of 1.
I hope that makes sense!
Steve.
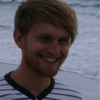
Daniel Schwemmlein
4,960 PointsHi Steve,
I tried what you suggested but it gave me a couple of compiler errors. The one thing that gave me the least amount of errors (still 1) was this:
public class ShoppingCart {
public void addItem(Product item, 1) { System.out.printf("Adding %d of %s to the cart.%n", 1, item.getName()); } }
Am I still doing something wrong? Sorry, this one really is not easy for me