Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial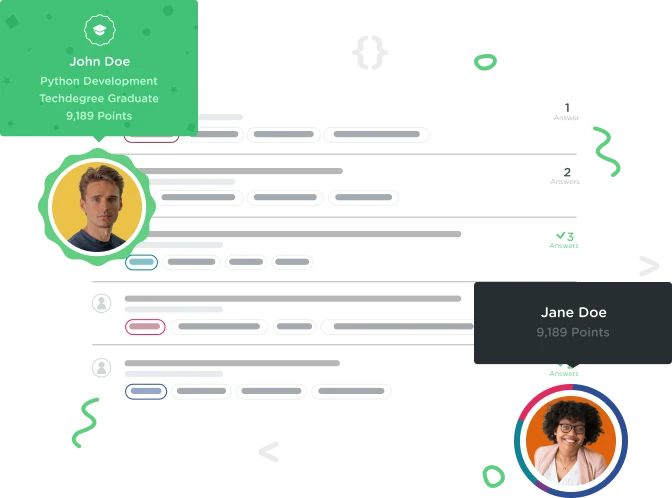

Dolly Kallab
925 Pointshow can i define a function?
how can i add numbers to max to be able to compare ?
function max(num1, num2) {
if (num1 > num2){
return num1 +" is the larger number";
} else{
return num2 + " is the larger number";
}
max(1,2);
}
8 Answers

Erik Nuber
20,629 Pointsfunction max(num1, num2) {
var max; if (num1 > num2){
max = (num1 + " is the larger number");
return(max);
} else{
max = (num2 + " is the larger number");
return(max);
}
}
max(1, 2);
Ive tried running it in the console and, it works fine, it spits out 2 is the larger number as expected. What is it you are trying to do? Because it does not actually return a number, it returns a string.
if you want just a number but also want the string you could do
var x = max(1, 2);
var y = parseInt(x);
In this case x would be a string stating "2 is the larger number" and y would just equal 2 which is a number.

Mike Nunyabeez
4,677 PointsIf you want to do more comparisons within the function, than you would want to write an else if statement....
if (x > 5) {
} else if (x > 50) {
} else {
}

Erik Nuber
20,629 PointsThere you go... sorry again.

Mike Nunyabeez
4,677 PointsIn response to Dolly. ... Here is an example of proper format.
function max(num1, num2) {
if (num1 > num2) {
console.log(num1 + " is the larger number");
} else {
console.log(num2 + " is the larger number");
}
}
max(1, 2);

Erik Nuber
20,629 PointsThe quizzes are very specific. You are asked to put the larger number into an alert...as so...
function max(num1, num2) {
if (num1 > num2) {
return num1;
} else {
return num2;
}
}
alert(max(1, 2));

Dolly Kallab
925 PointsThank you Erik that was the answer... and you thank you for the great feed back, i did learn a lot ..

Erik Nuber
20,629 PointsYou should take the max(1, 2) outside the function and you can list them then there.
function compareNums(num1, num2) {
var greaterNum;
if (num1 > num2){
greaterNum = (num1 + " is the larger number");
return(greaterNum);
} else{
greaterNum = (num2 + " is the larger number")
return(greaterNum);
}
}
var x = compareNums(1,2);
document.write(x);
console.log(compareNums(45, 12));
document.write(compareNums(24, 52));
etc.

Dolly Kallab
925 PointsThank you Eric for your quick answer, but it didn't work

Erik Nuber
20,629 Pointsmy fault, I didn't fix your code, I will in just a second. I was just answering your actual question and hadn't paid attention to the rest of it. I'm sorry...

Dolly Kallab
925 Pointsstill not working it says that function max doesn't return a number, I've updated my code to the following: function max(num1, num2) { var max; if (num1 > num2){ max = (num1 + " is the larger number"); return(max); } else{ max = (num2 + " is the larger number"); return(max);
}
}
max(1,2);

Mike Nunyabeez
4,677 PointsYou have a syntax error. Your final curly brace should be before you call the function and pass the arguments. If you want to try multiple sets, just call the function multiple times and pass a different set each time. The question is a little unclear to me, but this is what I think you are looking for.

Mike Nunyabeez
4,677 PointsIndentation is key to writing good scripts. Thats why I tell everyone to get OUT OF WORKSPACES and start writing code in Atom with LINTER!! :)

Dolly Kallab
925 Pointsthank you Mike, you are right :)

Dolly Kallab
925 Pointsand still not letting me pass the the quiz :(