Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial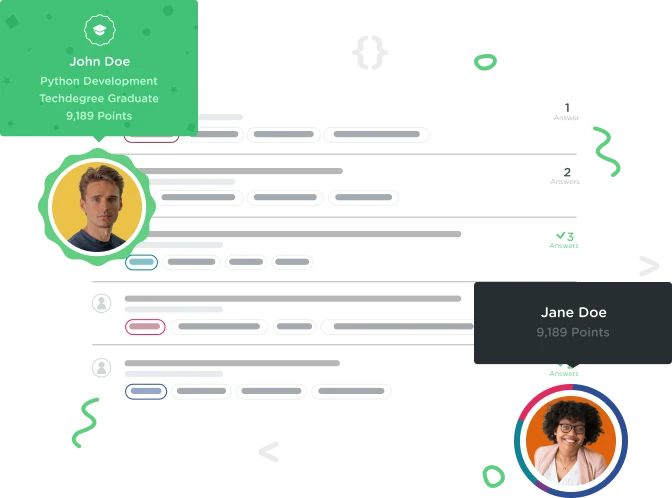
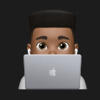
Caesar Bell
24,827 PointsHow can I have PHP spit out multiple lines of data to Email
Right now I have my form working fine, it sends out the information to an email, but for the questionnaire I am having some issues, with getting the answers to appear in the email that the user inputted. Right now it just gives me the first answer the user inputs but not the second, third or fourth answer the user inputs. The following HTML and PHP code can be found below
<!-- Modal -->
<div class="modal fade ques-modal-lg" id="questionModal" tabindex="1" role="dialog" aria-labelledby="questionnaireLabel">
<div class="modal-dialog modal-lg" role="document">
<div class="modal-content">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal" aria-label="Close"><span aria-hidden="true">×</span></button>
<h4 class="modal-title" id="questionnaireLabel">Questionnaire</h4>
</div>
<div class="modal-body">
<div id="form-message"></div>
<form id='ajax-questionaire' method='post' action='process.php'><!-- Start form -->
<!-- In browsers that support HTML5 form validation, this will prevent the form from being submitted until all the fields have been completed. -->
<div class="row">
<div class="form-inline" id="name-group">
<input type="text" class="form-control" id="fname" name="fname" placeholder="First name" required>
<input type="text" class="form-control" id="lname" name="lname" placeholder="Last name" required>
<input type="tel" class="form-control" id="telephone" name="telephone" placeholder="(888)-123-4567">
<input type="email" class="form-control" id="email" name="email" placeholder="hello@example.com" required>
</div>
</div>
<div id="questions-div"><!-- Start of the question div -->
<div class="row">
<div class="col-md-6">
<input type="text" class="form-control" name="ques1" id="ques1" placeholder="Questions 1" required>
</div>
</div>
<div class="row">
<div class="col-md-6">
<input type="text" class="form-control" id="ques2" placeholder="Questions 2" required>
</div>
</div>
<div class="row">
<div class="col-md-6">
<input type="text" class="form-control" id="ques3" placeholder="Questions 3" required>
</div>
</div>
<div class="row">
<div class="col-md-6">
<input type="text" class="form-control" id="ques4" placeholder="Questions 4" required>
</div>
</div>
</div><!-- End of question div -->
</form><!-- End of form -->
</div>
<div class="modal-footer">
<button type="submit" class="btn btn-default" id="submit">Send</button>
</div>
</div>
</div>
</div><!-- End of modal -->
<?php
// Only process POST reqeusts.
if ($_SERVER["REQUEST_METHOD"] == "POST") {
// Get the form fields and remove whitespace.
$firstName = strip_tags(trim($_POST["fname"]));
$LastName = strip_tags(trim($_POST["lname"]));
$name = $firstName . " " . $LastName;
$email = filter_var(trim($_POST["email"]), FILTER_SANITIZE_EMAIL);
$questionOne = trim($_POST["ques1"]);
$questionTwo = trim($_POST["ques2"]);
$questionThree = trim($_POST["ques3"]);
$questionFour = trim($_POST["ques4"]);
$messages = $questionOne . "\n" . $questionTwo . "\n" . $questionThree . "\n" . $questionFour;
// Check that data was sent to the mailer.
if ( empty($name) OR empty($messages) OR !filter_var($email, FILTER_VALIDATE_EMAIL)) {
// Set a 400 (bad request) response code and exit.
http_response_code(400);
echo "Oops! There was a problem with your submission. Please complete the form and try again.";
exit;
}
// Set the recipient email address.
// FIXME: Update this to your desired email address.
$recipient = "caesar.jd.bell@gmail.com";
// Set the email subject.
$subject = "New contact from $name";
// Build the email content.
$email_content = "Name: $name\n";
$email_content .= "Email: $email\n\n";
$email_content .= "Message: $messages";
// Build the email headers.
$email_headers = "From: $name <$email>";
// Send the email.
if (mail($recipient, $subject, $email_content, $email_headers)) {
// Set a 200 (okay) response code.
//http_response_code(200);
header('Location: successful.html');
} else {
// Set a 500 (internal server error) response code.
http_response_code(500);
echo "Oops! Something went wrong and we couldn't send your message.";
}
} else {
// Not a POST request, set a 403 (forbidden) response code.
http_response_code(403);
echo "There was a problem with your submission, please try again.";
}
?>
I was wondering if someone can provide guidence on what I am doing wrong and how I can fix this issues. If you want to see the site in its true form go to https://jnjfitness.com
3 Answers
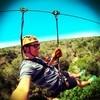
thomascawthorn
22,986 PointsLooking at your html, it would appear you've only given a 'name' attribute tot he first input. Only input from named inputs will be sent in the request (as part of the post data).
Try adding the name attribute like the first question:
<input type="text" name="quest2" class="form-control" id="ques2" placeholder="Questions 2" required>
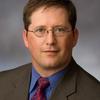
Ted Sumner
Courses Plus Student 17,967 PointsI agree with Tom Cawthorn. I am commenting to point out something that took me a long time to learn about. When you use " you can avoid long concatenation because PHP evaluates variables inside double quotes. For example, you could rewrite $names like this:
<?php
$names = "$firstName $LastName";
The other thing is that you normally will not capitalize the first letter in a variable. There is nothing wrong per se, but it is against normal convention. Classes are usually capitalized.

Sam Deacon
2,650 PointsVars render inside double quotes? Why didn't I know this already!?
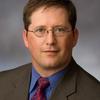
Ted Sumner
Courses Plus Student 17,967 Pointsit took me a long time to learn that. They render in double, but not in single.
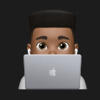
Caesar Bell
24,827 PointsThank you so much, I appreciate your feedback and I have made the necessary changes. Def cleaner coding.
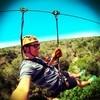
thomascawthorn
22,986 PointsYeah! It's called 'string interpolation'.
Using double quotes will tell php to replace placeholders with variable values, or . In other words, php knows that inside a string defined by double quotes, it's looking for keywords (placeholders) that begin with a dollar ($) sign. It will then replace the variable/placeholder with the value of the variable.
Read more in the double quotes section of the php docs.
I find it slightly better to always use curly braces around the placeholders (aka complex syntax) because you're removing any dollar related confusion. You don't have to just put string variables inside the curly braces, you can put in other expressions like:
<?php
echo "The sum is {$number1 + $number2}";
So for your example, I would do:
<?php
$names = "{$firstName} {$lastName}";
Ted Sumner I think you're right in saying convention (if by php-fig) says you must StudlyCap class names (and camelCase function names) - but I don't think there's anything official re variable names. Although referring to properties instead of variables, psr-1 says:
This guide intentionally avoids any recommendation regarding the use of $StudlyCaps, $camelCase, or $under_score property names.
PHP-fig is only one code standard though! So perhaps you're following another standard :) Generally speaking it's whatever you or your team find most readable (i.e. you set an internal standard that everyone follows), although I prefer camelCasing property and variable names too!
Hope this helps!
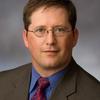
Ted Sumner
Courses Plus Student 17,967 PointsThat is interesting about the {} in a string. I have seen that done in one comment's code here in the forum but didn't know what it was for. If I understand it correctly, in this code the {} are unnecessary, but you would use them for consistency.
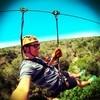
thomascawthorn
22,986 PointsThat's it - It's not 'wrong' to use them when you haven't got an expression, it's just unnecessary. Also, depending on your text editor/IDE, it usually highlights {$thisContents} differently - I find it makes my code a little more readable.
Caesar Bell
24,827 PointsCaesar Bell
24,827 PointsThank you so much for your help. It worked completely right, I can't believe I left out the name attributes for the other remaining questions.