Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial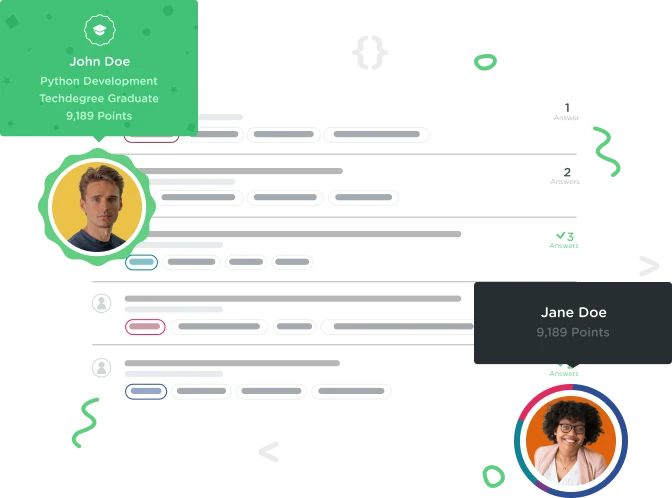

Max Smith
Courses Plus Student 3,973 PointsHow could I create pagination in php?
Say that I had a database containing 1000s of records and I wanted to return just 10 per page. What would be the best way to go about this?
In my head, the logic I would use would be to divide the array length by 10 and save it as a variable such as $pageresults
Somehow I would need to create a loop that looped through the results and displayed every 10 results ($pageresults)
I know I would need to use a GET variable but have hit a wall. Can anyone point me in the right direction.
I know the logic seems simple. Find the array length, divide by results by page and then loop through every 10 on each page.
But I can't seem to translate it into code. If anyone could help me, I would be forever grateful.
1 Answer
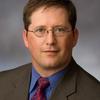
Ted Sumner
Courses Plus Student 17,967 PointsI suggest you take the building a simple web app courses in the PHP library. They build the site, including pagination as you want, refactor it in the second course, then add the data to a database in the third. Exactly what you want to ultimately do. This is the code on pagination from the course. These are from multiple files.
<?php
try {
$db = new PDO("mysql:host=localhost;dbname=shirts4mike","root","");
$db->setAttribute(PDO::ATTR_ERRMODE,PDO::ERRMODE_EXCEPTION);
$db->exec("SET NAMES 'utf8mb4'");
} catch (Exception $e) {
echo "Could not connect to the database.";
exit;
}
?>
<?php
/*
* Returns a specified subset of products, based on the values received,
* using the order of the elements in the array .
* @param int the position of the first product in the requested subset
* @param int the position of the last product in the requested subset
* @return array the list of products that correspond to the start and end positions
*/
function get_products_subset($positionStart, $positionEnd) {
$offset = $positionStart - 1;
$rows = $positionEnd - $positionStart + 1;
require(ROOT_PATH . "inc/database.php");
try {
$results = $db->prepare("
SELECT name, price, img, sku, paypal
FROM products
ORDER BY sku
LIMIT ?, ?"); //Two numbers are offset, then number returned.
$results->bindParam(1,$offset,PDO::PARAM_INT);
$results->bindParam(2,$rows,PDO::PARAM_INT);
$results->execute();
} catch (Exception $e) {
echo "Data could not be retrieved form the database. get_products_subset";
exit;
}
$subset = $results->fetchAll(PDO::FETCH_ASSOC);
return $subset;
}
?>
<?php
// retrieve current page number from query string; set to 1 if blank
if (empty($_GET["pg"])) {
$current_page = 1;
} else {
$current_page = $_GET["pg"];
}
// set strings like "frog" to 0; remove decimals
$current_page = intval($current_page);
$total_products = get_products_count();
$products_per_page = 8;
$total_pages = ceil($total_products / $products_per_page);
// redirect too-large page numbers to the last page
if ($current_page > $total_pages) {
header("Location: ./?pg=" . $total_pages);
}
// redirect too-small page numbers (or strings converted to 0) to the first page
if ($current_page < 1) {
header("Location: ./");
}
// determine the start and end shirt for the current page; for example, on
// page 3 with 8 shirts per page, $start and $end would be 17 and 24
$start = (($current_page - 1) * $products_per_page) + 1;
$end = $current_page * $products_per_page;
if ($end > $total_products) {
$end = $total_products;
}
$products = get_products_subset($start,$end);
?>
Not all of the comments appear to be updated with the addition of the database.