Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial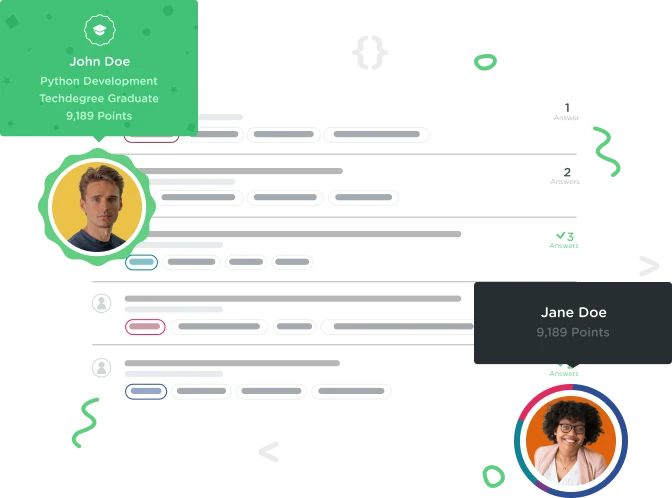

Tiago Ramos
2,380 PointsHow do I compare values[] array with the WeatherStationId?
Hi,
I've divided the question in several parts, as you see in the comments. I'm not sure what should I do in part 3. I want to verify what value inside the values[] array corresponds to WeatherStationId, so I'm thinking doing a for loop in values[]. Can you help me getting to the next phase?
Thank you guys :)
using System;
using System.IO;
namespace Treehouse.CodeChallenges
{
public class Program
{
public static void Main(string[] arg)
{
}
// 1. Create a static method named ParseWeatherForecast that takes a string[] parameter named values and returns a WeatherForecast.
public static WeatherForecast ParseWeatherForecast(string[] values)
{
// 2. Instantiate a WeatherForecast variable named weatherForecast
// and assign the appropriate value in the values array to the WeatherStationId property.
var weatherForecast = new WeatherForecast();
// 3. Use the sample data shown in WeatherForecast.cs to determine which value in the array is the WeatherStationId.
int values_lenght = values.lenght();
for(int i = 0; values_lenght > i; i++)
{
// verify if the current value is the WeatherStationId
weatherForecast.WeatherStationId;
}
// 4. Don't forget to return the weatherForecast in the new method!
return weatherForecast;
}
}
}
using System;
/*
Sample CSV Data
weather_station_id,time_of_day,condition,temperature,precipitation_chance,precipitation_amount
HGKL8Q,06/11/2016 0:00,Rain,53,0.3,0.03
HGKL8Q,06/11/2016 6:00,Cloudy,56,0.08,0.01
HGKL8Q,06/11/2016 12:00,PartlyCloudy,70,0,0
HGKL8Q,06/11/2016 18:00,Sunny,76,0,0
HGKL8Q,06/11/2016 19:00,Clear,74,0,0
*/
namespace Treehouse.CodeChallenges
{
public class WeatherForecast
{
public DateTime WeatherStationId { get; set; }
}
}
1 Answer
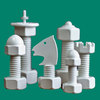
Steven Parker
241,970 PointsDid you try the "preview" button to see the compiler output? When I did, I saw "Type string[]
does not contain a definition for lenght
" So that issue is because the code has "values.lenght()
" instead of "values.Length
"
But you don't need a loop there at all. You only need to assign the WeatherStationId
property using the correct item in the "values" array. The instructions suggest looking at the comments in the other file to determine which one to use. Abd remember that index numbers start with zero.