Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial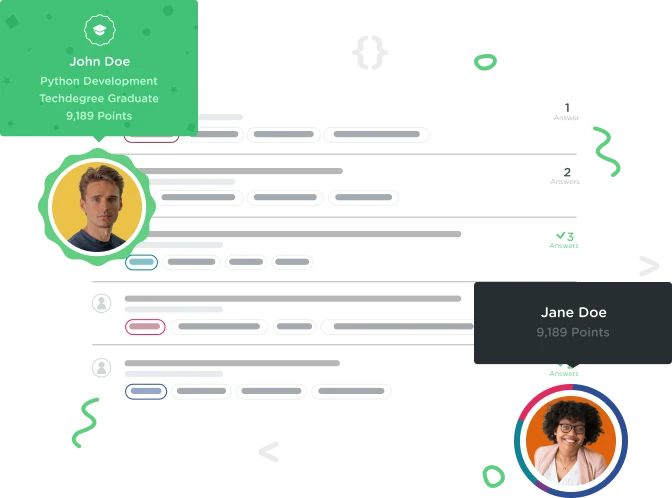
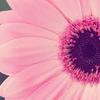
Carol Holmes
1,626 PointsHow do I declare a variable from letters to numbers?
I know that this is incorrect but I don't know how to define my parameters without creating random numbers first. If my thinking is entirely wrong, how should I precede?
var largerNumber = Math.floor( Math.random() * 7) + 1;
var smallerNumber = Math.floor( Math.random() * 6) + 1;
function max(largerNumber, smallerNumber){
return largerNumber;
}
2 Answers
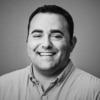
mikes02
Courses Plus Student 16,968 PointsHi Carol,
In this case there is actually no need for random numbers because you are just comparing which number has the greater value.
function max(number1, number2) {
if(number1 > number2)
{
return number1;
} else {
return number2;
}
}
alert(max(10, 2));
The challenge asks: "Create a new function named max() which accepts two numbers as arguments. The function should return the larger of the two numbers. You'll need to use a conditional statement to test the 2 numbers to see which is the larger of the two."
So we can create a function named max as shown above and pass in two parameters, in this case we call those number1 and number2. The second part asks us to use a conditional statement to test the two numbers to see which is larger, so we use an if/else to compare. For the IF we are saying if number1 is greater than number2, then return number1. However (ELSE) if number2 is greater than number1, return number 2. So that's how we compare.
The second part of the challenge asks us to call the function within an alert, so we can do alert(max(10,2)) where 10 and 2 are just randomly selected numbers to test our function and those could be replaced with any numbers you wanted.
Does that make sense?
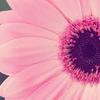
Carol Holmes
1,626 PointsThank you so much. I would not have asked about the semi-colons except that the code was accepted without them; the challenge regarded the code as correct even though they aren't there. I thought to ask whether or not this omission was optional. Thanks again mikes02; no need to reply but feel free to do so.
Carol Holmes
1,626 PointsCarol Holmes
1,626 PointsThank you! I modified your code to fit what I might have done had I been remotely close. Which leads to these questions: In the video, a function is written as --> function name (){}. In your code the second and last curly brace (after number2) is open not closed. A closer look I can see that there is a degree of nesting that I was supposed to imply, apparently. I didn't know that I could put braces inside other sets. It makes sense, and is a nice challenge. I wish I could practice similar problems until I mastered this concept; any suggestions? Also, I noticed that in the prior videos semi-colons follow the return statements - why not here?
mikes02
Courses Plus Student 16,968 Pointsmikes02
Courses Plus Student 16,968 PointsCarol,
The function has the curly braces as you mention:
The other curly braces you are seeing are related to the IF/ELSE:
So the IF/ELSE is being performed within the function. Also, you are correct, it is definitely good practice to end the line with semi-colons and they should be there, I have updated my code to include them.