Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial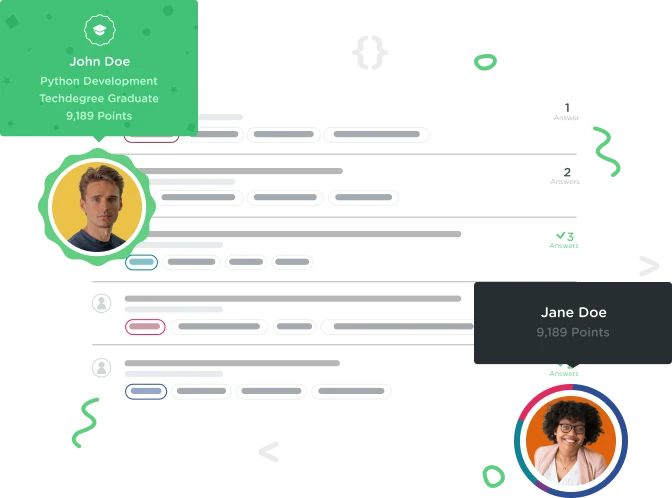

Babatunde Obidare
Courses Plus Student 10,514 PointsHow do I get this block of codes to print different messages depending on which condition is met?
using System;
namespace Treehouse.CodeChallenges {
class Program
{
static void Main()
{
try
{
Console.WriteLine("Enter the number of times to print \"Yay!\":");
int numTimes = Convert.ToInt32(Console.ReadLine());
for (int i = 1; i <= numTimes; i++)
{
if (numTimes.GetType() != typeof(int) || numTimes < 0)
{
continue;
}
else
{
Console.WriteLine("Yay!");
}
}
}
catch (FormatException)
{
Console.WriteLine("You must enter a whole number.");
}
}
}
}
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
try
{
Console.Write("Enter the number of times to print \"Yay!\": ");
int numTimes = Convert.ToInt32(Console.ReadLine());
for (int i = 1; i <= numTimes; i++)
{
if (numTimes.GetType() != typeof(int))
{
continue;
}
else if (numTimes < 0)
{
Console.WriteLine("You must enter a positive number.");
}
else
{
Console.WriteLine("Yay!");
}
}
}
catch (FormatException)
{
Console.WriteLine("You must enter a whole number.");
}
}
}
}
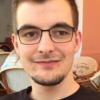
tobiaskrause
9,160 PointsNo problem :) Learning by doing/trying is still the best way to get coding experience. You can't make progress without mistakes... You even have to make mistakes to learn how to prevent them.
But C# is fun and later you can create Websites with ASP.NET (C#), mobile Apps and so much other stuff
Edit: And if you watch the roadmap of treehouse: https://teamtreehouse.com/roadmap you will see there are some nice upcoming courses for C# and ASP.NET (FINALLY)
2 Answers
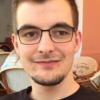
tobiaskrause
9,160 PointsAnd that would be the solution with a for loop. Int32.Parse is better in this case instead of Convert.ToInt32 since Int32.Parse specialy deals with strings while Convert.ToInt32() with any object TryParse() is maybe even better. Nice explenation: http://stackoverflow.com/questions/199470/whats-the-main-difference-between-int-parse-and-convert-toint32 Edit: Sry had bad formating
Edit: Just updated the code for the last Task
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.WriteLine("Enter the number of times to print \"Yay!\": ");
string read = Console.ReadLine();
try
{
int numTimes = Int32.Parse(read);
if (numTimes < 0)
{
Console.WriteLine("You must enter a positive number.");
}
else
{
for(int i = 1; i <= numTimes; i++) {
Console.WriteLine("Yay!");
}
}
}
catch(FormatException)
{
Console.Write("You must enter a whole number.");
}
}
}
}

Babatunde Obidare
Courses Plus Student 10,514 PointsThanks Tobias! The solution is perfect.
I guess as a beginner I'm going to write some codes that does not make sense initially but will learn.

Babatunde Obidare
Courses Plus Student 10,514 PointsThanks! I'm looking forward to the C# Objects course and other C#/ASP.Net courses.
tobiaskrause
9,160 Pointstobiaskrause
9,160 PointsThis part of your code makes no sense: You already tried to convert the Input to an int. There is no need to check if numTimes is a integer since, the program have an error if it is not possible to convert the input into an int. For example: When the user types "One" then Convert.ToInt32(Console.ReadLine()) will already lead to an Exception cause it is not possible to convert a String "One" to an integer
My solution to this challange was different I will post it soon