Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial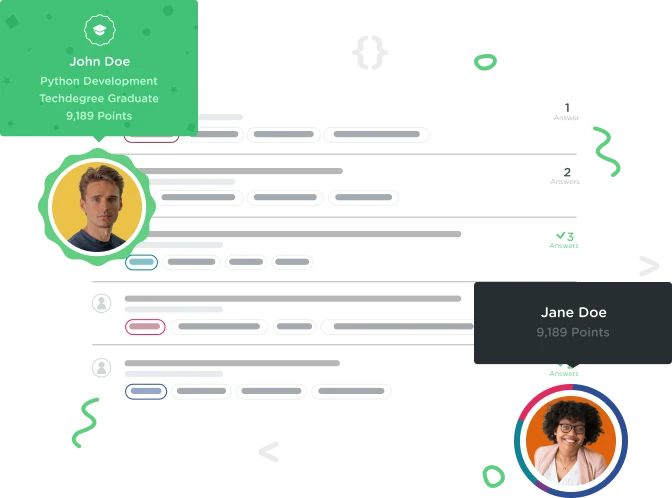
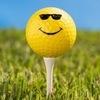
Jeff Vig
16,345 PointsHow do I notify the MainActivity when the song completes?
When the song ends, the button on the UI still says "Pause". I'm trying to get it to change back to "Play" when the song ends.
In the setOnCompletionListener, both the service and foreground are stopped. I thought this would be the place cause the button to change to "Play".
I thought I would send a message from the service, but the service does not know about activity.
What would be the best approach to accomplish this?
1 Answer

Ben Deitch
Treehouse TeacherHey Jeff!
First create a Messenger that we can use to communicate with the ActivityHandler:
public Messenger mActivityMessenger;
...
@Override
public void onCompletion(MediaPlayer mp) {
stopSelf();
stopForeground(true);
Message message = Message.obtain();
message.arg1 = 3;
try {
mActivityMessenger.send(message);
} catch (Exception e) {}
}
Then set the new 'mActivityMessenger' field by using the 'replyTo' property of the Message we pass to our PlayerHandler:
@Override
public void handleMessage(Message msg) {
if (msg.replyTo != null) {
mPlayerService.mActivityMessenger = msg.replyTo;
}
...
And finally add another 'else' branch to handle our new message argument:
else if (msg.arg1 == 3) {
mMainActivity.changePlayButtonText("Play");
}
Hope that helps!