Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial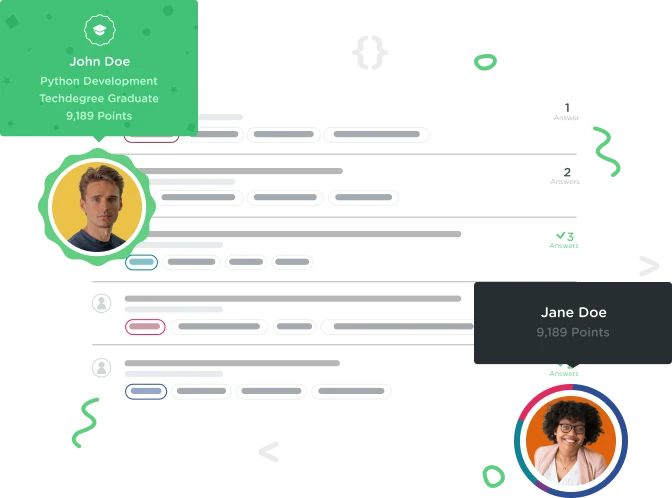
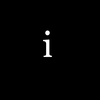
Furquan Ahmad
5,148 PointsHow do i output a certain bit of my array
The sql statement works, it produces this array :
Array ( [0] => Array ( [id] => 1 [name] => Matt G [subject1] => Maths [grade1] => A [subject2] => Further Maths [grade2] => A [subject3] => Computing [grade3] => A [subject4] => Physics [grade4] => B [final_maths] => A [attendance] => 99.1 [gender] => Male ) [1] => Array ( [id] => 11 [name] => Kieran Wallbanks [subject1] => Maths [grade1] => A [subject2] => Further Maths [grade2] => A [subject3] => Computing [grade3] => A [subject4] => Physics [grade4] => C [final_maths] => A [attendance] => 97 [gender] => Male ) )
I want to output just the final_maths grade. So it says your predicted grade will be final_maths ? I'm not sure on how to do this as i keep on getting errors.
<?php
error_reporting(E_ALL); ini_set('display_errors', 1);
require("includes/config.php");
//if not logged in redirect to login page
if(!$user->is_logged_in()){ header('Location: login.php'); }
//define page title
$title = "Predict a Student's Grade";
?>
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1">
<meta name="description" content="">
<meta name="author" content="">
<title>Past Student</title>
<!-- Bootstrap Core CSS -->
<link href="css/bootstrap.min.css" rel="stylesheet">
<!-- Custom Fonts -->
<link href="font-awesome/css/font-awesome.min.css" rel="stylesheet" type="text/css">
<!-- HTML5 Shim and Respond.js IE8 support of HTML5 elements and media queries -->
<!-- WARNING: Respond.js doesn't work if you view the page via file:// -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/libs/html5shiv/3.7.0/html5shiv.js"></script>
<script src="https://oss.maxcdn.com/libs/respond.js/1.4.2/respond.min.js"></script>
<![endif]-->
</head>
<body>
<?php
if (isset($_POST['name'])) {
$name = $_POST['name'];
$subject1 = $_POST['subject1'];
$grade1 = $_POST['grade1'];
$subject2 = $_POST['subject2'];
$grade2 = $_POST['grade2'];
$subject3 = $_POST['subject3'];
$grade3 = $_POST['grade3'];
$subject4 = $_POST['subject4'];
$grade4 = $_POST['grade4'];
$attendance = $_POST['attendance'];
$gender = $_POST['gender'];
$query = $db->prepare("SELECT * FROM paststudent WHERE subject1 = :subject1 AND grade1 = :grade1");
$query->bindValue(':subject1', $subject1, PDO::PARAM_STR);
$query->bindValue(':grade1', $grade1, PDO::PARAM_STR);
$query->execute();
$result = $query->fetchAll(PDO::FETCH_ASSOC);
/**$query = $db->prepare( " SELECT * FROM paststudent "
. " WHERE subject1 = :subject1 AND grade1 = :grade1");
$query->execute(array(':subject1' => $subject1, ':grade1' => $grade1)) ;
$rows = $query->fetchAll(PDO::FETCH_ASSOC); **/
/*$stmt = $db->prepare("SELECT * FROM paststudent WHERE subject1 = :subject1 AND grade1 = :grade1 AND subject2 = :subject2 AND grade2 =:grade2 AND subject3 = :subject3 AND grade3 =:grade3 ");
$stmt->execute(array(':subject1' => $subject1, ':grade1' => $grade1));
$rows = $stmt->fetchAll(PDO::FETCH_ASSOC); */
}
?>
<?php print_r($result); ?>
<h1> <?php echo "Your predicted grade is". $result?> </h1>
<h3> <a href="memberpage.php">Home</h3>
</body>
</html>
2 Answers
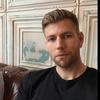
Paul Yabsley
46,713 PointsWhat errors are you getting? Looks like your query is returning 2 results.
Array (
[0] => Array (
[id] => 1
[name] => Matt G
[subject1] => Maths
[grade1] => A
[subject2] => Further Maths
[grade2] => A
[subject3] => Computing
[grade3] => A
[subject4] => Physics
[grade4] => B
[final_maths] => A
[attendance] => 99.1
[gender] => Male
)
[1] => Array (
[id] => 11
[name] => Kieran Wallbanks
[subject1] => Maths
[grade1] => A
[subject2] => Further Maths
[grade2] => A
[subject3] => Computing
[grade3] => A
[subject4] => Physics
[grade4] => C
[final_maths] => A
[attendance] => 97
[gender] => Male
)
)
Are you expecting just one?
If you wanted to display one of the values from the above array you could use $result[0]['final_maths'] for the first or $result[1]['final_maths'] but that doesn't seem like its what you want if you don't know which row to use.
Perhaps you want to change your query so you're only ever getting a single result back and then use fetch() rather than fetchAll to get just one array. Then you can just output $result['final_maths'].
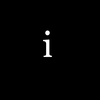
Furquan Ahmad
5,148 PointsIt's outputting 2 arrays as 2 people from the database took maths "subject1" and got an "a" grade1.
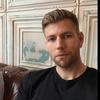
Paul Yabsley
46,713 PointsOK, so which one do you want to display the grade for?
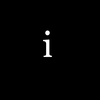
Furquan Ahmad
5,148 PointsJust the first one, as i'm hoping to add more WHERE statements, for example subject1 = :subject1 AND grade1 = :grade1 AND subject2 =:subject2 AND grade2 =:grade2 AND subject3 = :subject3
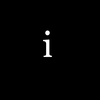
Furquan Ahmad
5,148 Pointsis this code any better
$query = $db->prepare( " SELECT * FROM paststudent ". " WHERE subject1 = :subject1 AND grade1 = :grade1");
$query->execute(array(':subject1' => $subject1, ':grade1' => $grade1)) ;
$rows = $query->fetchAll(PDO::FETCH_ASSOC);
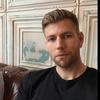
Paul Yabsley
46,713 PointsIt depends on what you want to do with the results from the query. If you have a query that returns multiple rows but you don't know how many you could loop over the query results and print the name and grade from each returned row. For example:
foreach($rows as $row) {
echo "<p>The predicted grade for " . $row['name'] . " is " . $row['final_maths'] . "</p>";
}
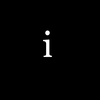
Furquan Ahmad
5,148 PointsWhen the predicted grade is an A it works, however with other conditions it doesn't work.
<?php print_r($result); ?>
<h1> <?php echo "Your predicted grade is ". $result[0]['final_maths']?> </h1>
<h3> <a href="memberpage.php">Home</h3>
</body>
</html>
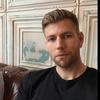
Paul Yabsley
46,713 PointsIt shouldn't matter what the actual grade is. $result is a multi-dimensional array i.e. an array which contains two additional arrays. I think it is this that is tripping you up.
Your SQL query is returning multiple rows but you are attempting to output the value from just one of the rows. Did you see my comment above about using a loop to output the grade from each returned row?
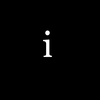
Furquan Ahmad
5,148 PointsYes i tried that, i'm trying to do the query with a for loop so it only outputs the first output instead of all the conditions .
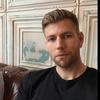
Paul Yabsley
46,713 PointsHmmm, if you just want to use the first result from the query I think you could just use fetch() instead of fetchAll(). That will give you a $result of:
Array (
[id] => 1
[name] => Matt G
[subject1] => Maths
[grade1] => A
[subject2] => Further Maths
[grade2] => A
[subject3] => Computing
[grade3] => A
[subject4] => Physics
[grade4] => B
[final_maths] => A
[attendance] => 99.1
[gender] => Male
)
So going back to your original post you'd change your code to be:
$query = $db->prepare("SELECT * FROM paststudent WHERE subject1 = :subject1 AND grade1 = :grade1");
$query->bindValue(':subject1', $subject1, PDO::PARAM_STR);
$query->bindValue(':grade1', $grade1, PDO::PARAM_STR);
$query->execute();
$result = $query->fetch(PDO::FETCH_ASSOC);
// fetch() instead of fetchAll just gets the first result
// Output the predicted grade (or any item from the array) like this
echo '<p>Your predicted grade is a' . $result['final_maths'];
Furquan Ahmad
5,148 PointsFurquan Ahmad
5,148 PointsYou have been amazing, thank you for your help so far !!!!! :)