Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial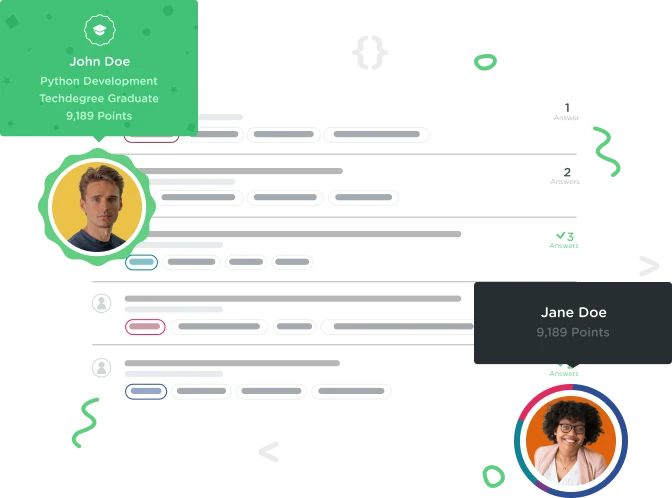

Caleb Cox
Full Stack JavaScript Techdegree Student 7,765 PointsHow do I set up setInterval(), clearInterval(), setTimeout(), and clearTimeout() properly? Also..do I need to use all 4?
Here is my code:
//creates an empty array for viewedQuotes var viewedQuotes= []; //sets interval variable var intervalID; //sets a timeout variable var timeoutID;
//selects random quote var getRandomQuote = function() { //holds random number from 0 to quotes.length and selects that obj in array var randomQuote = Math.floor(Math.random() * quotes.length); //takes randomQuote out of quotes array var splicedQuote = quotes.splice(randomQuote, 1)[0]; //pushes it into empty array viewedQuotes.push(splicedQuote); //if the quotes array runs dry if(quotes.length === 0){ //recycles viewedQuotes back into quotes array quotes = viewedQuotes; viewedQuotes= []; } //getRandomQuote returns splicedQuote to be printed in printQuote return splicedQuote; };
//prints quote var printQuote = function() { //calls on selected splicedQuote var getQuote = getRandomQuote(); //builds html text using the selected object's keys var html = "<p class='quote'>" + getQuote.quote + "</p>"; html +="<p class='source'>" + getQuote.source; html +="<span class='citation'>" +getQuote.citation + '</span>'; html +="<span class='tag'>" +getQuote.tag + '</span></p>'; //puts the constructed html into the 'quote-box' div document.getElementById('quote-box').innerHTML = html; //calls randomColor randomColor();
//Sets the "slideshow"?????? timeoutID= window.setTimeout(slowChange, 5000); };
//selects random background-color var randomColor = function(){ //sets random hue values var r = Math.floor(Math.random() * 256); var g = Math.floor(Math.random() * 256); var b = Math.floor(Math.random() * 256); //combines hues to make random color var color= "rgb(" + r + ", " +g+ ", " +b+ ")"; var difColor= "rgb(" + g + ", " +b+ ", " +r+ ")"; //selects the body and styles the background to make it randomColor document.body.style.background= color; //selects the button and makes its background a different color document.getElementById('loadQuote').style.background=difColor; };
//trying to set 5sec duration for each quote var slowChange = function(){ intervalID= window.setInterval(printQuote, 5000); };
//trying to stop the duration when next quote shows var stopChange = function() { window.clearInterval(intervalID); };
****I added an onclick="stopChange()" attribute to the button bc that's what examples were showing me
//click button to switch quotes document.getElementById('loadQuote').addEventListener("click", printQuote, false);
1 Answer

Erik Nuber
20,629 PointsThe difference between setTimeout and setInteral is that setTimeout fires only one after a specified amount of time and setInterval will fire over and over in set intervals (set times).
You use the clearInterval and clearTimeout to stop their respective set timers.
ex.
<input type="button" onclick="clearInterval(timer)" value="stop">
<script>
var i = 1
var timer = setInterval(function() { alert(i++) }, 2000)
</script>
The above will give an alert every two seconds that is just a number counting up until you hit a button that says stop. Just to note, it is not considered a good practice to use an inline script like onclick=... this should be handled in a script tag or better still an external js file.
ex
<input type="button" onclick="clearTImeout(timer)" value="stop">
<script>
var i = 1
function func() {
alert(i++)
timer = setTimeout(func, 2000)
}
var timer = setTimeout(func, 2000)
</script>
Here is the same but with setTimeout. As setTimeout only fires once, inside the function, it continues to call itself so that it fires repeatedly. Same thing happens, you would click a button called stop that would clear the timeout.
The other thing to notices is that when calling the clear methods, you pass in the parameter you are clearing. In both of these cases its "timer" which is the variable we assigned the interval and timeout too.