Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial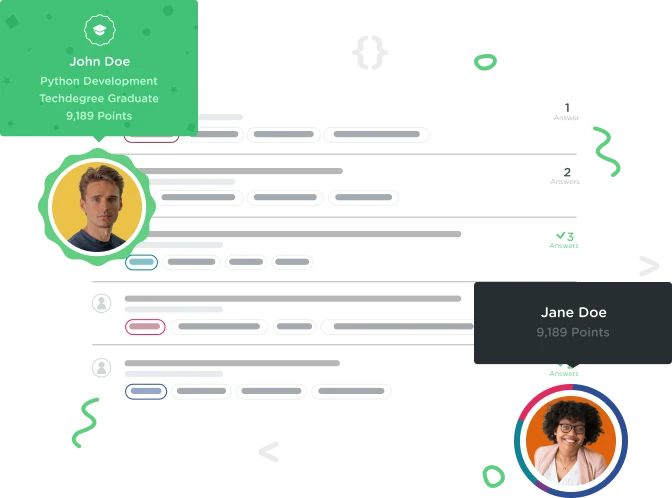
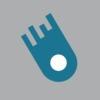
Gabriel E
8,916 PointsHow do I throw an Exception
Hi there,
I am on this Teacher Assistant challenge, and I have to throw an Illegal Argument Exception if the field name doesn't meet the requirements. Here is my code. I'm not sure what I'm doing wrong. Thanks!
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
if (! Character.isFieldName(fieldName)) {
throw new IllegalArgumentException("Doesn't meet requirements");
}
// These things should be verified:
// 1. Member fields must start with an 'm'
// 2. The second letter in the field name must be uppercased to ensure camel-casing
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
return fieldName;
}
}
1 Answer
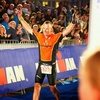
Steve Hunter
57,712 PointsHi Gabriel,
For this challenge you need to write the tests to enable the parameter passed to the method to pass the two tests defined in the comments.
If either test fails, then you need to throw the exception.
The first test is that the first letter of the parameter, fieldName
must be an 'm', as is the convention for class member variables. FOr that, you need to take the first character of fieldName
and see if it is 'm'. You can do that with:
fieldName.charAt(0) == 'm'
Next, you need to see if the second character is upper case, as is the convention with camel case variable names. That's a little trickier but the test takes the second charactoer of fieldName
using charAt(1)
and uses a class method of the Character
class called isUpperCase()
. Chaining that together gives you your second test:
Character.isUpperCase(fieldName.charAt(1))
If both of those things are true
then your parameter passed the test. If either is not true, then you throw the exception. Let's deal with that combination first. You need both outcomes to be true
- that's an AND, right? For that we use the &&
binary operator. All this is part of an if
statement, so we're saying IF these two things are true, then we pass, else, throw an exception. OK? Let's write that out:
if(fieldName.charAt(0) == 'm' && Character.isUpperCase(fieldName.charAt(1))){
/// both things are true - do whatever success looks like
} else {
//throw that exception
}
What does success look like? The question says the method will return the validated field name, fieldName
so we can add return fieldName;
to the first part of the if
statement. Next up we need to throw an exception on failure. THat's dead easy and needs no explanation so I'll just add this to the final code which looks like:
public static String validatedFieldName(String fieldName) {
if(fieldName.charAt(0) == 'm' && Character.isUpperCase(fieldName.charAt(1))){
return fieldName; // success!!
} else {
throw new IllegalArgumentException("ERROR!"); // exception thrown!
}
}
I hope that all makes sense!
Steve.