Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial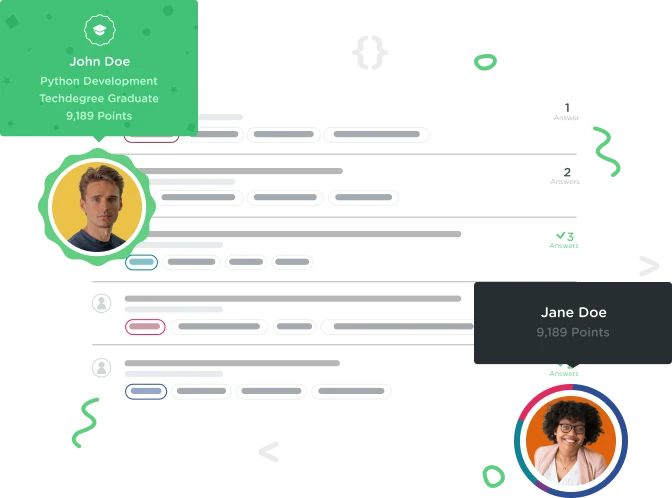
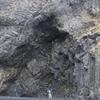
Jay Bhatt
6,502 PointsHow do I use the IllegalArgumentMethod to eliminate someone inputting laps that make the battery less than 0?
I have the code right here and I believe the condition of the laps having to be greater than 0 eliminates that problem. However, it is not accepting it. please help.
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive() {
drive();
}
public void drive(int laps) {
int lapRemain = mBarsCount + laps;
int lapsRemain= Math.abs(lapRemain);
if (lapsRemain > MAX_BARS && laps > 0) {
throw new IllegalArgumentException("Not enough battery remain");
}
mBarsCount = lapsRemain;
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}
2 Answers

Kristian Gausel
14,661 Points public void drive(int laps) {
if (laps > mBarsCount || laps < 0) {
throw new IllegalArgumentException("Not enough battery remain");
}
mBarsCount -= laps;
}
No need to make it complicated

Kristian Gausel
14,661 PointsAlthough you don't really need the "or laps < 0" part
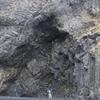
Jay Bhatt
6,502 PointsI went with just doing I have not tried your but I am sure it works public void drive(int laps) { int LapRemain = mBarsCount- laps; if (LapRemain < mBarsCount) { throw new IllegalArgumentException("Not enough battery"); } }

Kristian Gausel
14,661 PointsJust a tip: learn to not write complicated code for simple problems.
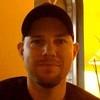
Jeremy Hill
29,567 PointsFor this challenge it is not really necessary to create another variable. All you really need to do is check whether the argument (laps) is more than the remaining battery (mBarsCount) if it is throw the exception if it is not then decrease the mBarsCount variable by the number that was passed in.
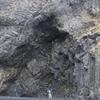
Jay Bhatt
6,502 PointsI saw your comment (the earlier one), it really helped, I was not thinking straight with that yesterday.
Here is what I did
public void drive(int laps) { int LapRemain = mBarsCount- laps; if (LapRemain < mBarsCount) { throw new IllegalArgumentException("Not enough battery"); } }
Jeremy Hill
29,567 PointsJeremy Hill
29,567 PointsThe variable that you created "lapRemain" you set it equal to the laps + mBarsCount. You need to remove the number passed in from the mBarsCount; like this: mBarsCount -= laps; This is a short cut to saying: mBarsCount = mBarsCount - laps; So each lap driven will be removed from the battery until the battery returns empty. If someone tries to drive further than the battery will allow an exception will be thrown alerting the user that they can't go that many laps.