Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial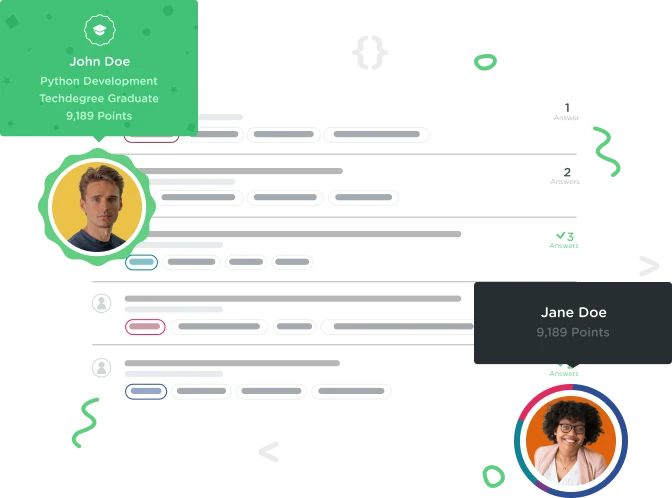

Giorgio Sky
Courses Plus Student 115 PointsHow does comprehension work with range?
{round(x/y) for y in range(1,11) for x in range(2,21)}
Given the above code, what is going on? I would expect that every number in:
[2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20]
gets divided by every number in:
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
But the result is:
{0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20}
How is this obtained? What does python do under the hoods? Thanks for any answer. And thanks for all these python courses, they are so useful. Really.
1 Answer

Iain Simmons
Treehouse Moderator 32,305 PointsBecause you're using the curly braces in this comprehension, you're doing a set comprehension, not a list comprehension. So you're getting the unique values, not all of them in order.
Change your curly braces to square brackets and you're all good to go:
>>> [round(x/y) for y in range(1,11) for x in range(2,21)]
[2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 1, 2, 2, 2, 3, 4, 4, 4, 5, 6, 6, 6, 7, 8, 8, 8, 9, 10, 10, 1, 1, 1, 2, 2, 2, 3, 3, 3, 4, 4, 4, 5, 5, 5, 6, 6, 6, 7, 0, 1, 1, 1, 2, 2, 2, 2, 2, 3, 3, 3, 4, 4, 4, 4, 4, 5, 5, 0, 1, 1, 1, 1, 1, 2, 2, 2, 2, 2, 3, 3, 3, 3, 3, 4, 4, 4, 0, 0, 1, 1, 1, 1, 1, 2, 2, 2, 2, 2, 2, 2, 3, 3, 3, 3, 3, 0, 0, 1, 1, 1, 1, 1, 1, 1, 2, 2, 2, 2, 2, 2, 2, 3, 3, 3, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 2, 2, 2, 2, 2, 2, 2, 2, 2, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 2, 2, 2, 2, 2, 2, 2, 0, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 2, 2, 2, 2, 2, 2]
Giorgio Sky
Courses Plus Student 115 PointsGiorgio Sky
Courses Plus Student 115 PointsYep, true, I missed that part of the explanation. Though they should be unordered, according to set documentation... am I wrong?
Thank you so much for the answer anyway ;)
Iain Simmons
Treehouse Moderator 32,305 PointsIain Simmons
Treehouse Moderator 32,305 PointsYou're not wrong: technically if it recorded the order it was inserted, 2 would be first. There may be some Python thing that just displays it in ascending order when every value is an integer.