Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial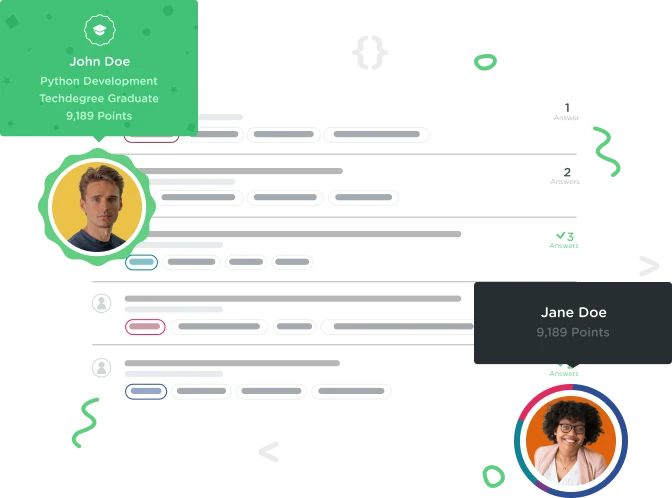

Ashish Mehra
Courses Plus Student 73 PointsHow to access database connection in another class
Hey I am working on php project for oop practice. In my project folder I include all classes under classes directory and my database connectivity is store in BASE class.
class BASE {
//creating variables for storing db operations
private static $connection = null; //variable for checking db connectivity
private $_pdo,$_query;
//creating a function which process db connecting when class instantiate
public function __construct()
{
try{
$this->_pdo = new PDO("mysql:host=".GetConfig::get_config("mysql/host").
";port=".GetConfig::get_config("mysql/port").
";dbname=".GetConfig::get_config("mysql/database")."","".GetConfig::get_config("mysql/username")."");
$this->_pdo->setAttribute(PDO::ATTR_ERRMODE,PDO::ERRMODE_WARNING);
}catch(Exception $e){
echo $e->getMessage();
}
}
//creating a method for checking database connectivity is exist or not
public static function CheckConnection()
{
if(!isset(self::$connection)){
self::$connection = new BASE();
}
return self::$connection;
}
public function usernameExist($username){
try{
$this->_query = $this->_pdo->prepare("select count(user_id) from user_info where userName = ?");
$this->_query->bindParam(1,$username,PDO::PARAM_STR);
$this->_query->execute();
if($this->_query->fetchColumn(0) == 1){
return true;
}
return false;
}catch(Exception $e){
$e->getMessage();
}
}
public function emailExist($email){
try{
$this->_query = $this->_pdo->prepare("select count(user_id) from user_info where email = ?");
$this->_query->bindParam(1,$email,PDO::PARAM_STR);
$this->_query->execute();
if($this->_query->fetchColumn(0) == 1){
return true;
}
return false;
}catch(Exception $e){
$e->getMessage();
}
}
//user authentication
public function user_id($userName){
try{
$this->_query = $this->_pdo->prepare("select user_id from user_info where userName = ?");
$this->_query->bindParam(1,$userName,PDO::PARAM_STR);
$this->_query->execute();
$result = $this->_query->fetchColumn(0);
return $result;
}catch(Exception $e){
$e->getMessage();
}
}
public function getPass($userName){
try{
$this->_query = $this->_pdo->prepare("select password from user_info where userName = ?");
$this->_query->bindParam(1,$userName,PDO::PARAM_STR);
$this->_query->execute();
$result = $this->_query->fetchColumn(0);
if(!empty($result)){
return $result;
}else{
echo "Username and password combination not match";
}
}catch(Exception $e){
$e->getMessage();
}
}
public function record($data){
array_pop($data);
$field = implode(",", array_keys($data));
$value = "'".implode("','", $data)."'";
$this->_query = $this->_pdo->prepare("insert into user_info ($field) values($value)");
if($this->_query->execute()){
return true;
}else{
return false;
}
}
}
How i can execute function like public function getPass($userName),public function record($data) from seperate class using same connectivity.
1 Answer

Vicente Armenta
11,037 PointsHello,
I don't know why you are using "BASE" for a class name, but it's ok. What I usually do to use functions from another class is the following.
//file_dir app\Base.php
<?php
class BASE {
public function getPass( $userName ) {
......
}
}
The on the code that you want to use it you need to require the file and instantiate the object
//file_dir app\main.php
<?php
require_once( 'Base.php' );
$objConnection = new BASE();
$objConnection->getPass( 'mighty_duck' );
if your function is static then you don't need to instantiate the object
<?php
require_once( 'Base.php' );
BASE::getPass( 'mighty_duck' );
Hope it helps.
Regards.