Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial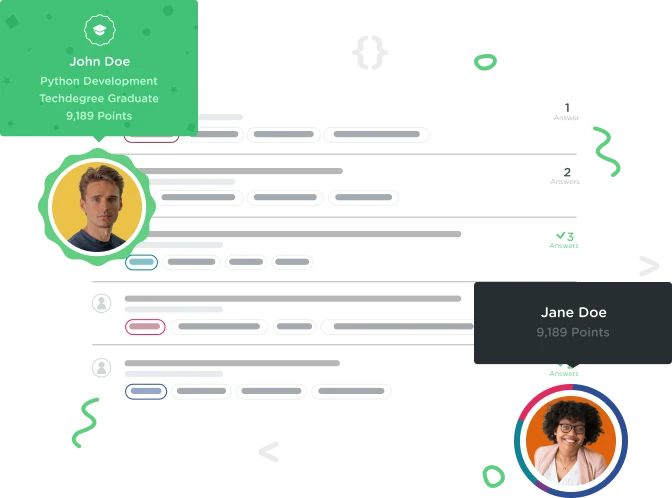

Aryaman Dhingra
3,536 PointsHow to change background color with JS
I have some test code here, in which I am trying to change the background color of a page with the click of a button. The code seems correct to me but I don't know where I am going wrong, as the color is not changing. Here is the code.
<body>
<div>
<input type='submit' id='red' class='buttons' value='Red'>
<input type='submit' id='blue' class='buttons' value='Blue'>
<input type='submit' id='green' class='buttons' value='Green'>
<input type='submit' id='purple' class='buttons' value='Purple'>
</div>
<script>
var blue = document.querySelector('#blue');
var red = document.querySelector('#red');
var green = document.querySelector('#green');
var purple = document.querySelector('#purple');
blue.addEventListener('click', => {
document.body.style.backgroundColor = 'blue';
});
red.addEventListener('click', => {
document.body.style.backgroundColor = 'red';
});
green.addEventListener('click', => {
document.body.style.backgroundColor = 'green';
});
purple.addEventListener('click', => {
document.body.style.backgroundColor = 'green';
});
</script>
</body>
1 Answer

andren
28,558 PointsThe issue is your arrow functions. When you create an arrow function that does not take any parameters you need to have empty parenthesis before the fat arrow. You can't just leave them off like you have done in your code.
Like this:
<body>
<div>
<input type='submit' id='red' class='buttons' value='Red'>
<input type='submit' id='blue' class='buttons' value='Blue'>
<input type='submit' id='green' class='buttons' value='Green'>
<input type='submit' id='purple' class='buttons' value='Purple'>
</div>
<script>
var blue = document.querySelector('#blue');
var red = document.querySelector('#red');
var green = document.querySelector('#green');
var purple = document.querySelector('#purple');
blue.addEventListener('click', () => {
document.body.style.backgroundColor = 'blue';
});
red.addEventListener('click', () => {
document.body.style.backgroundColor = 'red';
});
green.addEventListener('click', () => {
document.body.style.backgroundColor = 'green';
});
purple.addEventListener('click', () => {
document.body.style.backgroundColor = 'purple';
});
</script>
</body>
I also changed the color assignment value for the purple button, which you had forgotten to do in your code.
Aryaman Dhingra
3,536 PointsAryaman Dhingra
3,536 PointsThank you!! It works like a charm now!!