Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial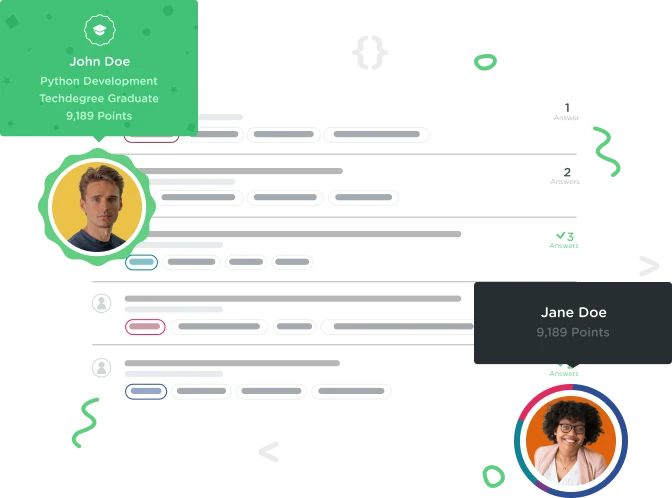

Colton Wollin
738 PointsHow to create a form that will ask for a record you want to update in php.
So I want a form that will search for the record to update and then display the record after updating. I'm really at a loss on how to do this! I know how to make a contact form that links to mysql database but I can't figure out how to do what I just said above that links to mysql database.
1 Answer
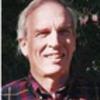
jcorum
71,830 PointsHere are some general guidelines. My apologies if I go over stuff you already know.
The first question is whether you want the user of the page to select the record to edit. If so, then you need to provide some way for users to do that.
The next step is to query the database and bring back the record you want to update (or the record the user selected to update).
For this you would write a query, and you would submit it to the web server to pass on to the database server using mysqli or PDO:
<?php
$sql = "SELECT * FROM table_name WHERE recordID = nnn";
---mysqli or PDO code here---
$results = . . .
?>
This PHP can be at the top of the html page with the form, or it can be a separate page. If it's at the top of the same page, make sure you have some code to keep it from executing until the record has been selected. This is usually done in a PHP if statement.
The third step, after you get the record from the database, is to display it in the form fields.
The results of the query will come back as a recordset, which will be stored in a variable, say $results. Then, in each of the form's elements you would echo the appropriate returned value. E.g., if you have a textbox for firstName, you would do this:
<input type="text" name="firstName" id="firstName" value="<?php echo $results['firstName']; ?> />
It's a little bit trickier to echo for check boxes, radio buttons and selects, but there are plenty of instructions on the web on how to do that. For each type you would use $results[. . .] with the appropriate field name inside the brackets in one way or other.
If you know Javascript and AJAX, you can do all of this without a full page refresh. Which provides a nicer user experience. They select a record to update, and the form fields get filled!
Let me know if you need more detail on any of the steps.