Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial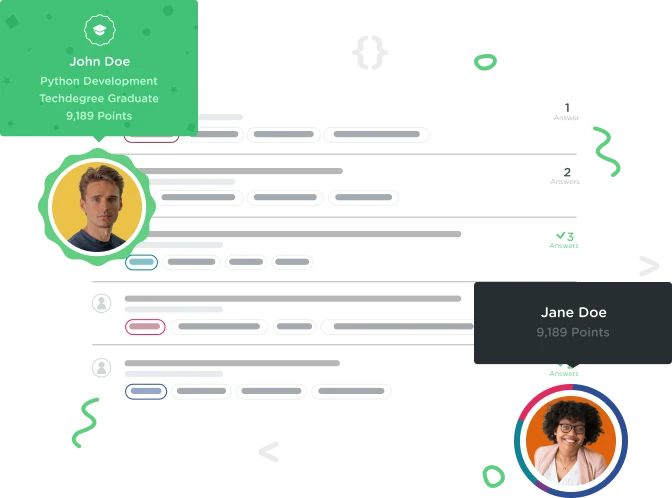
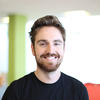
Kristian Woods
23,414 PointsHow to create a login/register form?
Hey, I've been piecing together a database that receives data through an HTML form. At the moment, I'm trying to create the login part. However, I'm having trouble comparing the password the user enters against the stored password.
I'm trying to compare the username AND password
<?php
include("inc/login_query.php");
?>
<!DOCTYPE html>
<html>
<head>
<title>LogIn</title>
<link rel="stylesheet" type="text/css" href="main.css">
</head>
<body>
<div class="inner-form-bg">
<form action="welcome.php" method="post">
<h1 class="form-h1">Member Login</h1>
<input type="text" placeholder="Username:" name="username" class="field-input req" required><br>
<input type="password" placeholder="Password:" name="password" class="field-input req" required><br>
<button type="submit" class="submit field-input">Submit</button><br>
<button type="button" class="field-input new-acc-btn"><a href="signUp.php" class="create-acc">Create Account</a></button>
</form>
</div>
</body>
<footer>
</footer>
</html>
<?php
include("inc/dbconnection.php");
//check for form submission
if($_SERVER['REQUEST_METHOD'] == 'POST') {
$errors = array();
//check for username
if(empty($_POST['username'])) {
$errors = "You forgot to enter your Username";
} else {
$username = mysqli_real_escape_string($connection, trim($_POST['username']));
}
//check for password
if(empty($_POST['password'])) {
$errors[] = "You forgot to enter your password";
} else {
$password = mysqli_real_escape_string($connection, trim($_POST['password']));
}
if(empty($errors)) {
$sql_login = "SELECT * FROM login WHERE username LIKE '$username' AND passcode LIKE SHA1('$password')";
$result = mysqli_query($connection, $sql_login);
if(mysqli_num_rows($result) == 1) {
echo "welcome " . $username . "<br>";
} else {
echo "Account not found" . "<br>";
}
}
}
?>
I continue to get "Account not found".
I tried testing JUST the username, and it worked - I received the welcome message
Any help would be great
Thanks
1 Answer
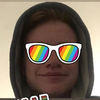
Nils Garland
18,416 PointsHi Kristian! I think I have what you are looking for. In your SQL statement you are telling it to look for the username and password that are 'LIKE' you one they entered. This means that if I entered "BLA" and the password is "BLABLA" I would still be authenticated.
$pwd = SHA1('$password');
$sql_login = "SELECT * FROM login WHERE username='$username' AND passcode='$pwd'";
Then after the statement
if (!$row=mysqli_fetch_assoc($result)) {
header("Location: index.php?error=invalid_u_or_p");
exit();
} else {
$_SESSION['id'] = $row["id"];
header("Location: home.php?success=session_start");
}
That may not be exactly what it is in your case, but it should give you an idea. Just out of curiosity why SHA1?
I hope this helps in any way, if not maybe I just understood the question wrong :P