Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial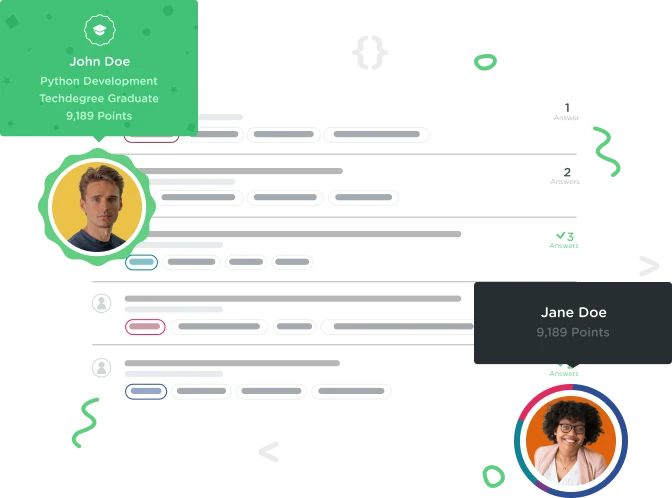

Jackie Jen
2,723 PointsHow to define a specific key and get the value in associative array foreach loop
Hi,
my code is below
<?php
$age = array("Peter"=>"35", "Ben"=>"37", "Joe"=>"43");
foreach($age as $x => $x_value) {
}
?>
how can i get Ben value in foreach loop?
11 Answers
Tiffany McAllister
25,806 PointsForeach loops iterate over every item in an array. To echo the value of one item only you can do:
echo $age["Ben"];
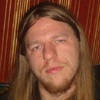
Vedran Brnjetić
6,004 PointsHi, the $x variable will be the key and $x_value will be the value.
In your foreach loop "Ben" will show up in the $x variable.
If you only want to echo "Ben" , you need to either check if ($x_value==37) or if ($x=="Ben")
Like this:
<?php
$age = array("Peter"=>"35", "Ben"=>"37", "Joe"=>"43");
foreach($age as $x => $x_value) {
if($x_value==37) echo $x;
}
?>
or like this:
<?php
$age = array("Peter"=>"35", "Ben"=>"37", "Joe"=>"43");
foreach($age as $x => $x_value) {
if($x=="Ben") echo $x;
}
?>
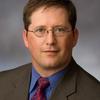
Ted Sumner
Courses Plus Student 17,967 PointsEdited to add syntax highlighting. If you put the language immediately after the first three ` it will give you syntax highlighting.
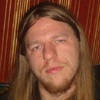
Vedran Brnjetić
6,004 PointsThank you, sir, I'm still getting used to this markdown.
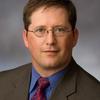
Ted Sumner
Courses Plus Student 17,967 PointsMy pleasure. It is how we learn. I have found your comments helpful. Thank you for your contributions to the forum.
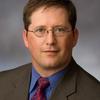
Ted Sumner
Courses Plus Student 17,967 PointsEDIT: this will not work
I think you want the key()
function, although it has been a long time since I have seen or used this. I would try the code below first, then try your code with the this inside the foreach loop: echo key($x_value);
<?php
foreach ($age as $x) {
echo key($x);
}
Let me know how this works because I have a project this would work sell for.
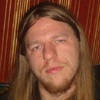
Vedran Brnjetić
6,004 PointsThe key function works a bit fuzzy with foreach. If you want to use the key function, use while loop like this:
<?php
while ($x_value = current($age)) {
$x=key($age);
if ($x_value== '37') {
echo $x;
}
next($age);
}
?>
this will print out Ben
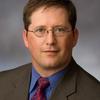
Ted Sumner
Courses Plus Student 17,967 PointsIf I understand the question, he wants all of the keys, not just one. I admit I have spent a bit of time on this and cannot get it to work. My solution above does not. I will try to get it and post a snapshot to the workspace I am using when It does work.
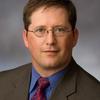
Ted Sumner
Courses Plus Student 17,967 PointsThe course on PHP functions has a link to the built in PHP function array_keys()
. That is what you want to use.
<?php
$age = array(
"Peter"=>35,
"Ben"=>37,
"Joe"=>43,
);
$keys = array_keys($age);
print_r($keys);
// prints (not in this format) [0] => Peter, [1] => Ben, [2] => Joe
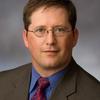
Ted Sumner
Courses Plus Student 17,967 PointsI just reread the question asked and it was specifically how to get the value for ben using a foreach loop. If you only want one value from an array, the foreach loop is not the right tool. That specifically goes through every element of the array and does the code inside the loop.
I may not understand what you are trying to accomplish. Can you give us a little more as to what you are trying to accomplish?

Jackie Jen
2,723 PointsHi Guys
Thanks alot all of your reply, Let me put an scenario. Let said i have doing sql query select from database. Let said User 1 have below key and values in associative array
$results= array("id"=>"35", "name"=>"Jen", "country"=>"CA");
foreach($results as $x => $x_value) {
}
?>
Since i have sql query select all the database field. Then i know there is a field call "country". So i want to echo out the country value therefore i know which country of user1 is living.
I'm not sure is this the right way to use foreach loop or while loop. Please advice. Appreciate all yours reply
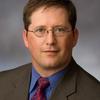
Ted Sumner
Courses Plus Student 17,967 PointsThis is what I understand. You have a database with a bunch of users. In your example above, the row with the id of 35 is Jen from CA. You query your database for all the info on id 35 and get an array back. Since you know the structure and the column you want, you just need to echo $results['country'];
.
If you have a list of many users and you want to know how many of each country you have, you would actually use a query for that result. I don't know how to do that off the top of my head, but I know there are SQL elements that do that for you. You can avoid multidimensional arrays that way.
If you want the result for multiple users at the same time, I would probably have a class for the database call and send it the users for individual database queries. Then process the results one at a time. Again, this would avoid a multidimensional array.
If you want something to happen to everything in the array, use a foreach loop. Use a while loop if you want to do something a set number of times or if there is some condition that halts the loop.
I hope this helps.

Jackie Jen
2,723 PointsHi Ted,
Yes this is what i mean below.
<p>This is what I understand.You have a database with a bunch of users. In your example above, the row with the id of 35 is Jen from CA. You query your database for all the info on id 35 and get an array back. Since you know the structure and the column you want, you just need to echo $results['country'];.</p>
Does it mean that after query from database and output out in multidimensional arrays format is not the friendly way for extracting the value?
What is the best way for those who deals with the query from database and output out?
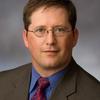
Ted Sumner
Courses Plus Student 17,967 PointsMultidimensional arrays are more complicated to work with, but definitely have their place. I like to think about how much work is involved when figuring out how to accomplish something. Lets say I want a specific piece of data from the database. I can write my query to get that piece directly. Then I use it. If I write my query to get everything, then I have have to process everything to get what I want, the computer is having to do much more work. It is much more processing to get everything and sort it again. Both the result and the processing take time.
But if I need to use everything, then maybe I want one query and process the results of everything. I think the best practice is to try to keep your code short and understandable. Something that you can come back to in a year and understand what you did quickly is always better, I think.

Jackie Jen
2,723 PointsHi Guys
$results[]= array("id"=>"35", "name"=>"Jen", "country"=>"CA");
foreach($results as $x ) {
echo $x['name'];
}
Above php code, i able to output the value of specific key. But i did not understand why i need to put
$results[]= array("id"=>"35", "name"=>"Jen", "country"=>"CA");
instead of
$results= array("id"=>"35", "name"=>"Jen", "country"=>"CA");
Please Help
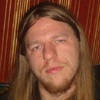
Vedran Brnjetić
6,004 PointsFirst option will make $results to be like a container which can hold information about all people from database.
Second option will make $results basically a single result. A single person.
Vedran Brnjetić
6,004 PointsVedran Brnjetić
6,004 PointsBut that will not echo "Ben". It will echo 37.
Tiffany McAllister
25,806 PointsTiffany McAllister
25,806 PointsMaybe I mis-read the question. She said she wanted to get "Ben value" so I just assumed she meant she wanted to echo out the value of Ben.