Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial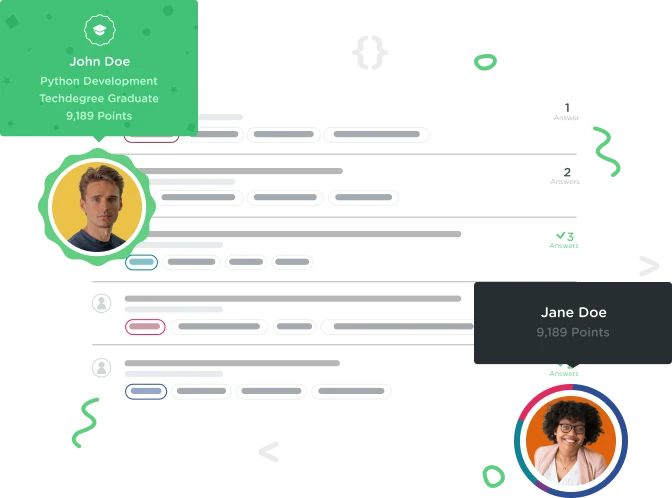
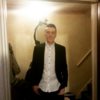
James Barrett
13,253 PointsHow to differentiate between two forms using $_SERVER["REQUEST_METHOD"] == "POST"
Hi, I am currently working on a project that implements this technique. However, how do you go about differentiating two forms on the same page? For example:
if($_SERVER["REQUEST_METHOD"] == "POST") {
$search = trim(filter_input(INPUT_POST,"user_search",FILTER_SANITIZE_SPECIAL_CHARS));
$search = preg_replace("#[^0-9a-z]#i", "", $search);
if($search == "") {
$error_message = "Please enter something before submitting";
}
}
Later on in this code segment, an SQL query would be performed if the $error_message was not set.
I want another form on the same page that applies the same principles as this... It stores the user's input into a variable which is sanitized and trimmed and uses the same error checking above (checking if the user's input was empty, etc). However I feel this code would look quite messy and disorganised.
What are some ways of differentiating between two forms on the same page?
1 Answer
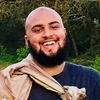
Lee Ravenberg
10,018 PointsI think the easiest way to distinguish the difference between two form submissions, is by adding a hidden
input type in your form markup. For example:
<form action="someprocessing.php" method="post">
<input type="text" name="user_search">
<input type="hidden" name="number1" value="number1">
<input type="submit">
</form>
<form action="someprocessing.php" method="post">
<input type="text" name="user_search">
<input type="hidden" name="number2" value="number2">
<input type="submit">
</form>
Your global $_POST
variable will hold the value
of either the number1
or number2
input field, depending on which form has been submitted. I would check on that like this:
// Returns number1 or number2 string value depending on which form is submitted. If none, return null.
function whatFormHasBeenSubmitted()
{
if ( !empty( $_POST["number1"] ) ) {
return "number1";
} elseif ( !empty( $_POST["number2"] ) ) {
return "number2";
} else {
return null;
}
}