Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial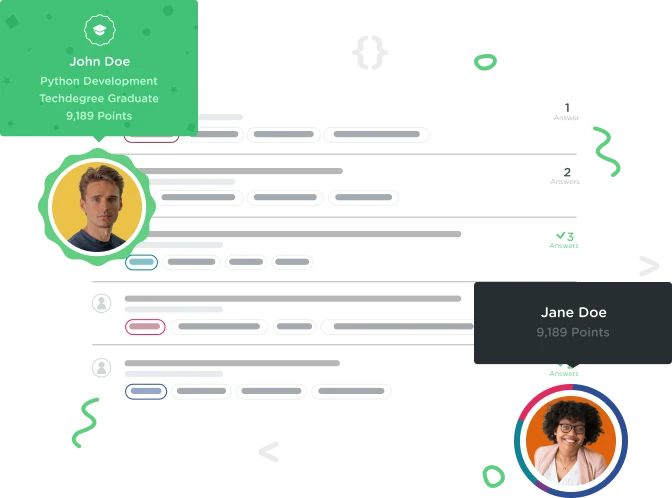

Kenneth Phillips
Courses Plus Student 10,188 PointsHow to execute two activities
I have an android app where I want to get feedback from the user. I made it so they can fill out a form and send it via email. However in the process this starts a new activity. After the person sends the email I want the app to go back to the Main Activity and restart. However if I try to make it go to another activity afterwards than it skips sending the email and defeats the purpose of the page. So how do I have the app send the email and then go back to the MainActivity?
8 Answers

Mark Filter
32,718 PointsThe key here is to launch your Email Intent using startActivityForResult()
rather than startActivity()
. Once the Intent finishes sending the email, the Activity will fire finish()
and return you to your requesting application. You can test the returned result for specific conditions such as Cancelled, Success, or Error if you want advanced customized handling as well.

Mark Filter
32,718 PointsonActivityResult()
is specifically for handling your return back to your original application. If the result was okay, then you could Toast a message saying "Email was sent successfully", or you could notify the user of the error (best practice for giving the user feedback).
// Constant
private static int SEND_EMAIL_REQUEST = 1;
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (resultCode == RESULT_OK){
if (requestCode == SEND_EMAIL_REQUEST) {
// do what you'd like here upon successfully sent email on return
}
}
}

Kenneth Phillips
Courses Plus Student 10,188 PointsSo would this look correct?
package com.teamtreehouse.testapplication;
import android.content.Intent; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.EditText;
public class ContactFormActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_contact_form);
((Button) findViewById(R.id.btnOK)).setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
sendEmail();
}
});
}
private int Send_Email_Request = 1;
private void sendEmail() {
String to = ((EditText) findViewById(R.id.txtTo)).getText().toString();
String number = ((EditText) findViewById(R.id.phoneNumber)).getText().toString();
String mess = ((EditText) findViewById(R.id.txtMessage)).getText().toString();
Intent mail = new Intent(Intent.ACTION_SEND);
mail.putExtra(Intent.EXTRA_EMAIL, new String[]{to});
mail.putExtra(Intent.EXTRA_SUBJECT, number);
mail.putExtra(Intent.EXTRA_TEXT, mess);
mail.setType("message/rfc822");
startActivityForResult(mail, Send_Email_Request);
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (resultCode == RESULT_OK){
if (requestCode == Send_Email_Request) {
// do what you'd like here upon successfully sent email on return
Intent mainAct = new Intent(this, MainActivity.class);
startActivity(mainAct);
}
}
}
}

Mark Filter
32,718 PointsEverything looks great up until you get to your onActivityResult()
. This should be in your MainActivity already. If you executed this, it would duplicate your MainActivity or crash your app (one of the two). When you send your email, it should automatically return you back to your MainActivity in the originating application.

Kenneth Phillips
Courses Plus Student 10,188 PointsOk so once I put it back into main activity what should I put for the if statement in the onActivityResult() method so that it doesn't create two activities but takes it back to mainActivity?

Mark Filter
32,718 PointsYou really use the onActivityResult()
to either pass data back from another activity or to give feedback to the user of success or errors. In your specific implementation, it doesn't seem to make sense to launch another Activity, most especially the MainActivity. If you need to get back to the Main Activity and you are not returning there after you submit an email, then consider the finish() method, or if you have been nested into other activities beyond just one, then you can clear the Activity stack by following these instructions: https://stackoverflow.com/questions/11460896/button-to-go-back-to-mainactivity

Kenneth Phillips
Courses Plus Student 10,188 PointsSo here is the code I put:
private void sendEmail() {
String to = ((EditText) findViewById(R.id.txtTo)).getText().toString();
String number = ((EditText) findViewById(R.id.phoneNumber)).getText().toString();
String mess = ((EditText) findViewById(R.id.txtMessage)).getText().toString();
Intent mail = new Intent(Intent.ACTION_SEND);
mail.putExtra(Intent.EXTRA_EMAIL, new String[]{to});
mail.putExtra(Intent.EXTRA_SUBJECT, number);
mail.putExtra(Intent.EXTRA_TEXT, mess);
mail.setType("message/rfc822");
startActivityForResult(mail, Pick_Email_Request);
finish();
}
The app is still crashing when I test it. So what do I do after the startActivityForResult() to make the application return to MainActivity and not crash?

Mark Filter
32,718 PointsRemove finish(); That method closes your Main Activity...which is what you need to remain active while you send your email.

Kenneth Phillips
Courses Plus Student 10,188 PointsIt works but doesn't return me to the MainActivity and just stays in the current activity which was my original problem. That's why I was wondering if you use the onActivityResult() method.

Mark Filter
32,718 PointsI would have to see your code and a screen cast of what's going on.

Kenneth Phillips
Courses Plus Student 10,188 PointsI just want to say thanks for taking the time to help out. I hope you understand this has just really confused me. Here is my current code:
package com.teamtreehouse.testapplication;
import android.content.Intent; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.EditText;
public class ContactFormActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_contact_form);
((Button) findViewById(R.id.btnOK)).setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
sendEmail();
}
});
}
private int Send_Email_Request = 1;
private void sendEmail() {
String to = ((EditText) findViewById(R.id.txtTo)).getText().toString();
String number = ((EditText) findViewById(R.id.phoneNumber)).getText().toString();
String mess = ((EditText) findViewById(R.id.txtMessage)).getText().toString();
Intent mail = new Intent(Intent.ACTION_SEND);
mail.putExtra(Intent.EXTRA_EMAIL, new String[]{to});
mail.putExtra(Intent.EXTRA_SUBJECT, number);
mail.putExtra(Intent.EXTRA_TEXT, mess);
mail.setType("message/rfc822");
startActivityForResult(mail, Send_Email_Request);
}
}
As for a screencast just let me know what you want to see. Thanks

Mark Filter
32,718 PointsTry
startActivity(mail);
rather than
startActivityForResult(mail, Send_Email_Request);
I don't think you need to get anything from your implicit intent...just to send an email.

Kenneth Phillips
Courses Plus Student 10,188 PointsHere is what happens. I hit the send button and it asks through what platform I want to send it. Gmail, Yahoo, etc. After I send the email in this platform it exits that existing application and goes back to before the I hit send on the email form activity with everything filled out. It will do the email but it won't return to MainActivity.
Kenneth Phillips
Courses Plus Student 10,188 PointsKenneth Phillips
Courses Plus Student 10,188 PointsHey thanks for responding. However I looked at the documentation for startActivityForResult() and I'm confused on exactly how to set this up. Here is the code I currently have:
So it says I must put request code in the second parameter that will be put in onActivityResult(). That's where I'm confused because I'm not sure how to set that up exactly.