Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial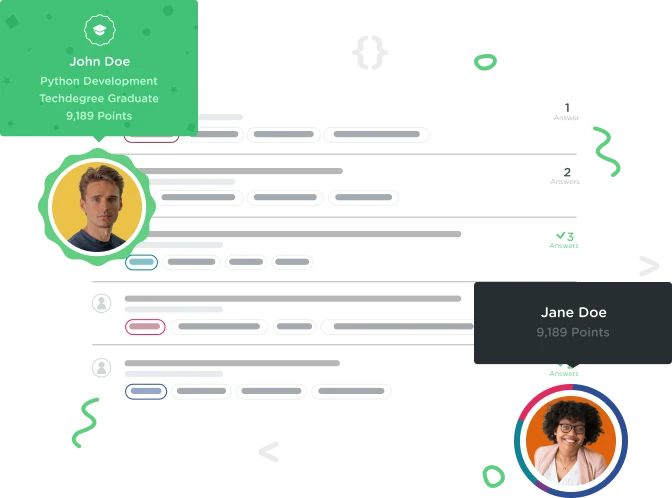
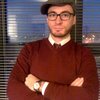
Frank D
14,042 PointsHow to handle multiple Checkboxes Values in a FORM using PHP!
How can I get the values from a multiple checkbox? Basically I am developing a website that has one checkbox section for the "Customers interests" and one for the "Privacy & Policy" and it goes this way:
1) I am intrested in:
[]Web Design
[]Web Development
[]Branding
[]Photography
2)
[] I agree to the the Terms & Conditions of this website.
I have already coded the html form properly with all the values and names, now I need to get the values from the php code.
7 Answers
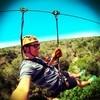
thomascawthorn
22,986 PointsHere's an example:
index.php
<form action="/checkbox.php" method="post">
<input type="checkbox" name="flavours[]" value="strawberry">
<input type="checkbox" name="flavours[]" value="vanilla">
<input type="checkbox" name="flavours[]" value="chocolate">
<input type="submit" value="Submit!">
</form>
checkbox.php
<?php
echo "<pre>";
var_dump($_POST);
exit;
submitting the form with a random selection of flavours will give:
<?php
array(2) {
["flavours"]=>
array(2) {
[0]=>
string(10) "strawberry"
[1]=>
string(7) "vanilla"
}
}
If you leave all checkboxes unselected, $_POST['flavours'] will not be set.
You could do:
<?php
if (array_key_exists('flavours', $_POST))
{
//
}
// or
if (isset($_POST['flavours']))
{
//
}
to check.
John Steer-Fowler
Courses Plus Student 11,734 PointsYou might be able to find the answer to your question here:
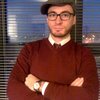
Frank D
14,042 PointsHi John, thanks for your reply. Hovewer I am struggling yet cause I don't understand where the values for the checkboxes must to be stored.
Is it ok to store them in the variables this way?
$name = trim($_POST["name"]);
$email = trim($_POST["email"]);
$message = trim($_POST["message"]);
$intrest = trim($_POST["intrest"]); // These are the checkboxes for intrests
$privacy = trim($_POST["privacy"]); // This is the checkbox for the privacy & policy
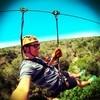
thomascawthorn
22,986 PointsIf you're doing this:
<label for="interests">
<input type="checkbox" name="interests[]" value="web-design">Web Design
</label>
<label for="interests">
<input type="checkbox" name="interests[]" value="web-development">Web Development
</label>
Then the data you're looking for will be stored as an array inside:
<?php
$_GET['interests'] // array('web-design', 'web-development')
foreach($_GET['interests'] as $interest) {
echo $interest;
}
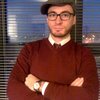
Frank D
14,042 PointsHI TOM and thanks for replying. I was getting an error with your code, but then it worked with this lil variation:
$_POST['interests'];
foreach($_POST['interests'] as $interest) {
echo $interest;
}
Basically instead of _GET i used _POST.
The only thing now is that I get only one checkbox value when i receive the email (the last one checked on the list) even if you choose two or more. Any though?
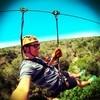
thomascawthorn
22,986 PointsOh crap sorry! Yes $_POST for in place of $_GET, good find.
if you do:
<?php
echo "<pre>";
var_dump($_POST['interests']);
exit;
what do you get back?
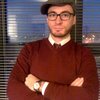
Frank D
14,042 PointsHi Tom, I get NULL
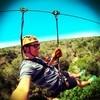
thomascawthorn
22,986 PointsDid you check any boxes before submitting the test form?
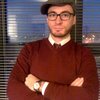
Frank D
14,042 PointsHi Tom, here is what i get without ticking any checkboxes:
Notice: Undefined index: privacy in /Applications/MAMP/htdocs/website.com/contact.php on line 9
Notice: Undefined index: interest in /Applications/MAMP/htdocs/website.com/contact.php on line 10
line 9 $privacy = trim($_POST["privacy"]);
line 10 $interest = trim($_POST["interests"]);
Notice: Undefined index: interest in /Applications/MAMP/htdocs/website.com/contact.php on line 80
echo "<pre>";
line 79 var_dump($_POST['interest']);
line 80 exit;
line 81 NULL
</pre>
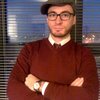
Frank D
14,042 PointsHere's what i get ticking one or more checkboxes:
string(8) "Branding"
So basically the privacy checkbox seems to work fine but the multiple option one ($interest checkbox) is echoing only the last choice in order of appearance even if i tick more than once
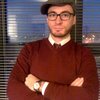
Frank D
14,042 PointsHi Tom, that's all correct, although when I use PHPMailer and try that online I get back: array in my email and not the values as supposed to be. I have used:
$email_body = $email_body . "<b>Flavors</b>: " . $flavors . "<br>";
I think I will shortly post the whole code for a better understanding.
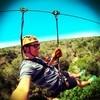
thomascawthorn
22,986 PointsYep, you can't echo out an array - php will print 'array' instead.
You could do:
<?php
$flavours = implode(',', $flavours);
see implode
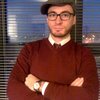
Frank D
14,042 PointsWowzy, it works perfectly now! Thank you Tom, you resolved my 1 week of hard work trying to understand checkboxes.
The only thing is, when I use the spam code (below), as Randy Hoyt showed us in one video, I get the error message and it doesn't send the email anymore. This happens only with the checkboxes, if I delete them the email works just fine, same way, if I delete the spam code the email works fine and I finally get all the values from the checkboxes.
What the problem with that spam code, any idea?
There was a problem with the information you entered.
// ANTI-SPAMMER ATTACK CODE, CHECKS THAT NONE OF THE FIELDS HAS MALICIOUS CODE
// This code loops through the post array using a for each loop, loading each element one at a time into a working variable called value. It then uses a function and a conditional to check if each post variable contains a malicious value. If it finds one, it stops the PHP file from executing.
if (!isset($error_message)) {
foreach( $_POST as $value ){
if( stripos($value,'Content-Type:') !== FALSE ){
$error_message = "There was a problem with the information you entered.";
}
}
}
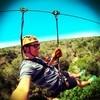
thomascawthorn
22,986 PointsUsing checkboxes in this way submits an array through the post request - as we've already seen.
Sending an array will affect the content type, which is why that particular check is failing for you.
If it's valid to send an array of checkbox values through your form, that's fine! You just need to add a conditional/check to not validate your checkboxes in that particular bit of the validation.