Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial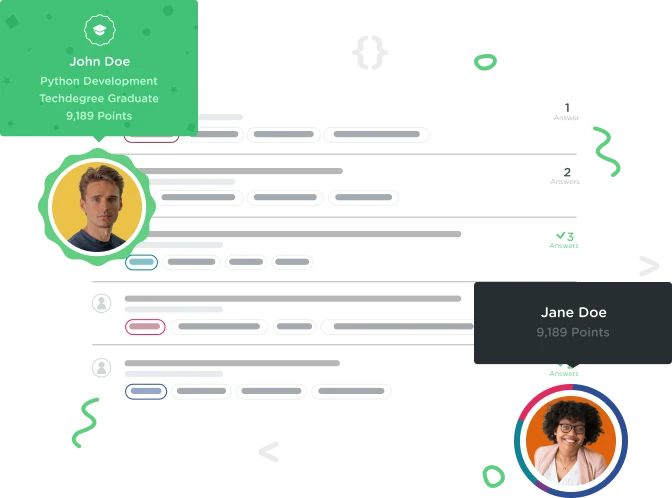

Montalvo Miguelo
24,789 PointsHow to implement OOP with my Models
I'm playing with OOP to create a kind of mini-framework to apply what I learnt in OOP php, enhancing simple web app, PDO courses...
At the moment all the queries are done by helper functions in helpers.php.... and the instance of PDO is in /model folder... How can I enhance the queries with OOP and the business logic of my example?
1 Answer
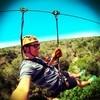
thomascawthorn
22,986 PointsThey key is to think first about how you want to call the data. E.g. you're probably going to want to say "Show me all the movies". How would that be most readable in your code?
Probably something like:
<?php
$movie = new Movie;
$movies = $movie->all();
foreach($movie->all() as $movie) {
echo $movie->name;
echo $movie->description;
echo $mobie->rentalPrice;
}
// or
$movie = $movie->findByID($movieID);
echo $movie->name;
echo $movie->description;
echo $mobie->rentalPrice;
When you know how you want to use it, you can start coding! :-)
I would therefore create a model called 'Movie' and have 'Movie' extend Database. Inside your movie model is where you start to translate ->all() into MySQL. all->() would need to return an array of Movie objects - which is quite fun to work out ;)
I think something like
<?php
/**
* Get all the movies
*
* @return array
*/
public function all()
{
$movieResults = $this->execute('SELECT * FROM `movies`');
foreach($movieResults as $movieData)
{
// turn array into objects and fill each object with information from array
// something like
// $movie = new self;
// $movie->fill($movie);
// $movieObjects[$modie['id']] = $movie;
}
return $movieObjects;
}
You can write an execute method in the Database object - which you can call because you're extending Database.php.
The execute method could contain all of your try catch logic etc.. and will centralise everything.
I've never set something up like this from scratch before, but this is how I would get started! Hope if at least points you in some kind of direction.
Tom