Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial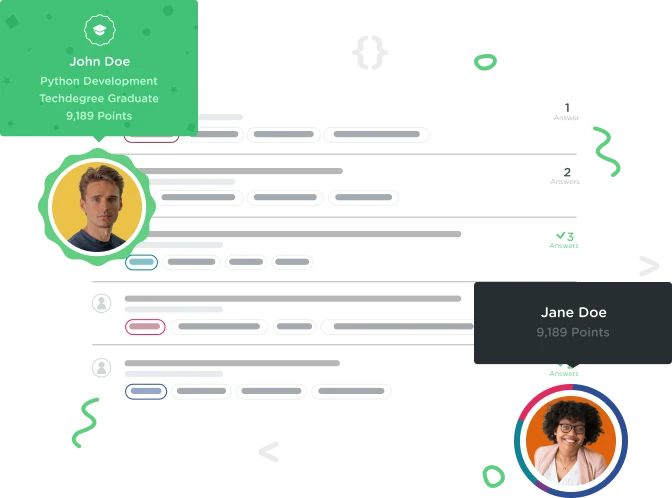
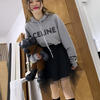
Kevin Narain
11,379 PointsHow to loop multiple elements in JavaScript?
Hi :)
I want to loop 50 fishes and 50 bubbles in the browser how do I do that? I've tried to create an array but it didn't work out. I've been struggling for 3 hours already. Your help would be apperciated. Thanks for reading 😀 I've put a comment in the JavaScript file to point out where I think the solution might possibly be.
let randomXValue = window.innerWidth - Math.floor(Math.random() * window.innerWidth) + 0 + 'px';
let randomYValue = window.innerHeight - Math.floor(Math.random() * window.innerHeight) + 0 + 'px';
let randomHueColor = Math.floor(Math.random() * 360) + 0 + 'deg'; // number between 0 en 360
// Checks if the element is in the viewport, if not then place fish or bubble back to coordinates (0, 0)
function isInViewport(element) {
const rect = element.getBoundingClientRect();
if (rect.top >= 0 &&
rect.left >= 0 &&
rect.bottom <= (window.innerHeight || document.documentElement.clientHeight) &&
rect.right <= (window.innerWidth || document.documentElement.clientWidth)) {
} else {
element.style.transform = `translate(0, 0)`;
element.style.filter = `hue-rotate(${randomHueColor})`;
}
}
// HERE THE FISHES GET CREATED BUT IF I PUT THIS INTO A FOR LOOP IT GETS OVERWRITTEN
// I MIGHT NEED AN ARRAY BUT I DON'T HOW TO GET MULTIPLE FISHES ON THE SCREEN.
const game = document.querySelector("game");
let fish = document.createElement("fish");
game.appendChild(fish);
fish.style.transform = `translate(${randomXValue}, ${randomYValue})`;
fish.style.filter = `hue-rotate(${randomHueColor})`;
isInViewport(fish);
// HERE THE BUBBLES GET CREATED BUT IF I PUT THIS INTO A FOR LOOP IT GETS OVERWRITTEN
// I MIGHT NEED AN ARRAY BUT I DON'T HOW TO GET MULTIPLE FISHES ON THE SCREEN.
let bubble = document.createElement("bubble");
game.appendChild(bubble);
bubble.style.transform = `translate(${randomXValue}, ${randomYValue})`;
isInViewport(bubble);
body {
background-color: #70CF51;
font-family: Arial, Helvetica, sans-serif;
overflow:hidden;
margin:0px; padding:0px;
}
fish, bubble, background {
position: absolute;
display: block;
box-sizing: border-box;
background-repeat: no-repeat;
}
fish {
background-image: url(../images/fish.png);
width:130px; height: 110px;
filter: hue-rotate(90deg);
cursor:pointer;
}
.dead {
background-image: url(../images/bones.png);
}
bubble {
background-image: url(../images/bubble.png);
width:55px; height: 55px;
cursor:pointer;
}
background {
background-image: url(../images/water.jpg);
width:100vw; height: 100vh;
background-size: cover;
}
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Pixel Aquarium</title>
<link rel="stylesheet" type="text/css" href="css/style.css">
<script defer src='js/main.js'></script>
</head>
<body>
<game>
<background></background>
</game>
</body>
</html>
1 Answer
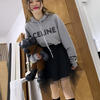
Kevin Narain
11,379 PointsHi guys I figured it out myself I didn't put the randomXValue and randomYValue in my function. Because functions are in the local scope I needed to reinitialize these variables.
function createFish() {
randomXValue = window.innerWidth - Math.floor(Math.random() * window.innerWidth) + 0 + 'px';
randomYValue = window.innerHeight - Math.floor(Math.random() * window.innerHeight) + 0 + 'px';
// vis element
let fish = document.createElement("fish");
fish = document.createElement("fish");
game.appendChild(fish);
// Vervang de x en y waarden van de vis en bubble door willekeurige waarden
fish.style.transform = `translate(${randomXValue}, ${randomYValue})`;
fish.style.filter = `hue-rotate(${randomHueColor})`;
isInViewport(fish);
}
function createBubble() {
let randomXValue = window.innerWidth - Math.floor(Math.random() * window.innerWidth) + 0 + 'px';
let randomYValue = window.innerHeight - Math.floor(Math.random() * window.innerHeight) + 0 + 'px';
// bubble element
let bubble = document.createElement("bubble");
game.appendChild(bubble);
bubble.style.transform = `translate(${randomXValue}, ${randomYValue})`;
isInViewport(bubble);
}