Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial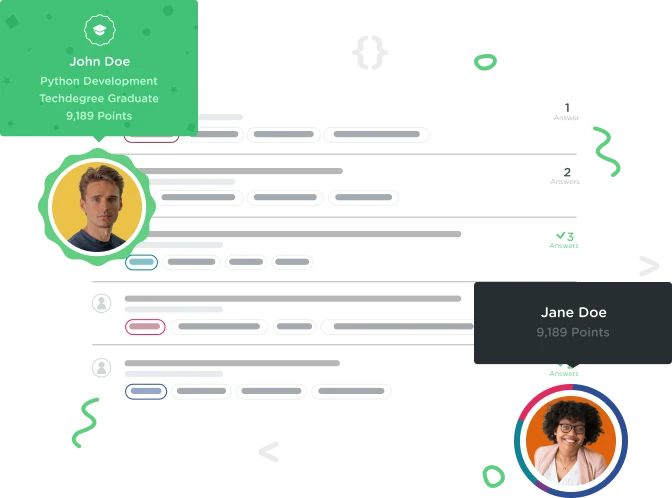

Stuart McPherson
15,939 PointsHow to put form dropdown and message together into email once form submitted
So I did the build a website with php course and got it all working. I want to add more fields (dropdowns and input fields) into my form but also have that data coming through on the email. What php code do I need to add to get this working?
{% extends 'main.twig' %}
{% block content %}
<div class=contact>
<h2>Getting In Touch</h2>
<p class="intro">If you would like further information about the club, or if you're looking for your son/daughter to join. Please submit via the enquiry form below:</p>
<form action="" method="post">
<fieldset>
<input name="name" type="text" placeholder="Full Name">
<input name="email" type="email" placeholder="Email Address">
<select class="dropdown" name="dropdown" size="1">
<option value="option">option</option>
<option value="option2">option2</option>
</select>
<textarea name="msg" placeholder="Your message..."></textarea>
</fieldset>
<input type="submit" class="button">
</form>
</div>
{% endblock content %}
<?php
require __DIR__ . '/vendor/autoload.php';
date_default_timezone_set ('Europe/London');
use Monolog\Logger;
use Monolog\Handler\StreamHandler;
//$log = new Monolog\Logger('name');
//$log->pushHandler(new Monolog\Handler\StreamHandler('app.txt', Monolog\Logger::WARNING));
//$log->addWarning('Warning');
$app = new \Slim\Slim(array(
'view' => new \Slim\Views\Twig()
));
$view = $app->view();
$view->parserOptions = array(
'debug' => true
);
$view->parserExtensions = array(
new \Slim\Views\TwigExtension(),
);
$app->get('/', function() use($app){
$app->render('index.twig');
})->name('home');
$app->get('/about', function() use($app){
$app->render('about.twig');
})->name('about');
$app->get('/contact', function() use($app){
$app->render('contact.twig');
})->name('contact');
$app->post('/contact', function() use($app){
$name = $app->request->post('name');
$email = $app->request->post('email');
$dropdown = $app->request->post('dropdown');
$msg = $app->request->post('msg');
if(!empty($name) && !empty($email) && !empty ($dropdown) && !empty($msg)) {
$cleanName = filter_var($name, FILTER_SANITIZE_STRING);
$cleanEmail = filter_var($email, FILTER_SANITIZE_EMAIL);
$cleanDropdown = filter_var($dropdown, FILTER_SANITIZE_STRING);
$cleanMsg = filter_var($msg, FILTER_SANITIZE_STRING);
} else {
//message the user that there was a problem
$app->redirect('/contact');
}
$transport = Swift_SendmailTransport::newInstance('/usr/sbin/sendmail -bs');
$mailer = \Swift_Mailer::newInstance($transport);
$message = \Swift_Message::newInstance();
$message->setSubject('Email from our website');
$message->setFrom(array($cleanEmail => $cleanName));
$message->setTo(array('treehouse@localhost'));
$message->setBody($cleanDropdown, $cleanMsg);
$result = $mailer->send($message);
if($result > 0) {
// send thank you message
$app->redirect('/thanks');
} else {
// send a message to the user that the message failed ot send
// log the error
$app->redirect('/contact');
}
});
$app->run();