Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial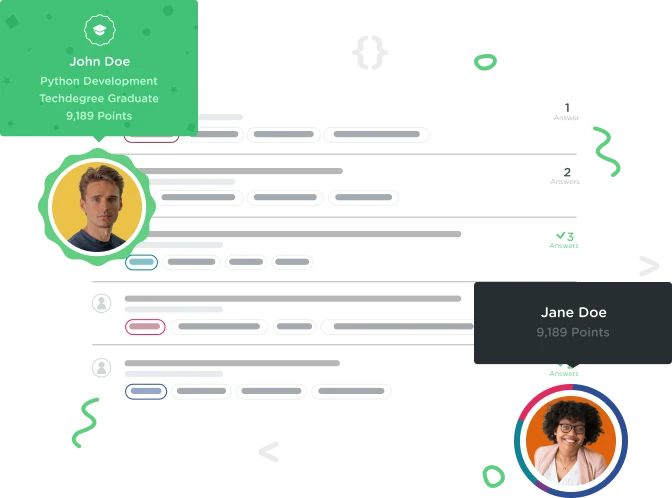
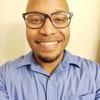
Anthony Smith
9,897 PointsHow to remove a div from the DOM when a user clicks the 'Delete' button
I'm trying to use Javascript to delete the .extra
div when a user clicks on the newly created button but when I click on the button I get the following error:
scripts.js:42 Uncaught TypeError: Cannot read property 'removeChild' of null at HTMLButtonElement.<anonymous> (scripts.js:42)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<link rel="stylesheet" href="css/main.css">
<title>Practice Manipulating the DOM</title>
</head>
<body>
<div class="container">
<h1></h1>
<p class="desc"></p>
<ul>
<li><input> Play music</li>
<li><input> Swim</li>
<li><input> Bike ride</li>
<li><input> Code</li>
</ul>
<div class="extra">
<p>This is extra content you need to delete.</p>
</div>
</div>
<script src="js/scripts.js"></script>
</body>
</html>
// 7: Create a <button> element, and set its text to 'Delete'
// Add the <button> inside the '.extra' <div>
const button = document.createElement('button');
button.innerHTML = "Delete";
const buttonPlace = document.getElementsByClassName('extra')[0];
buttonPlace.appendChild(button);
// 8: Remove the '.extra' <div> element from the DOM when a user clicks the 'Delete' button
const removeDiv = document.querySelector('extra');
button.addEventListener('click', () => {
removeDiv.removeChild('p');
});
2 Answers
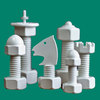
Steven Parker
241,970 PointsIt looks like there are two issues:
- the "removeChild" method should be called on the parent of the element being removed
- the argument to "removeChild" should be the element being removed
buttonPlace.parentNode.removeChild(buttonPlace);
The "removeDiv" is not needed here since "buttonPlace" already refers to the desired element. But FYI: when a class name is used as a selector, it should be preceded by a period (".extra
").
UPDATE: As suggested by ri, if browser compatibility is not an issue, you could use the newer "remove" method:
buttonPlace.remove();

ri wir
PHP Development Techdegree Student 32 Points<div class="extra">...</div>
const removeDiv = document.querySelector('extra');
Check your mistake of your selector name
extra
, should bediv.extra
or.extra
My Solution
<div id="extra">
<p>...</p>
</div>
//* prefer to use this method to avoid like this kind of mistake in future
const removeDiv = document.getElementById('extra'); // document.querySelector('#extra')
button.addEventListener('click', () => {
//* to avoid error, you can check with condition statement OR try..catch
if (removeDiv) {
removeDiv.remove();
}
});