Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial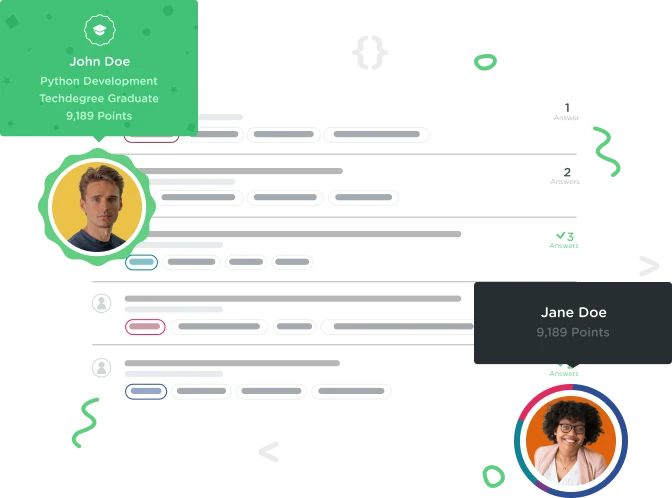
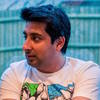
Punal Chotrani
8,817 PointsHow to remove field from an array if the column is == '\n' in the database.
I have a function to retrieve all the data using PHP PDO, but i'm need to modify with some conditions.
The trouble is that in the database i have value's of "\n", and would like to remove the field arrays with the value of '\n'. Any help regarding this would be very helpful.
Below is my code.
<?php
if (isset($_GET['id'])) {
$sql = "SELECT * FROM pod_repo WHERE pod_id =:id";
$statement = $pdo->prepare($sql);
$pod_id = filter_input(INPUT_GET, 'id');
$statement->bindValue(':id', $pod_id, PDO::PARAM_STR);
$statement->execute();
$formatString = '<div class="holds-columns-together">
<div class="breather">
<h1 class="inline">%s</h1>
<h3>Address: %s</h3>
<h3>Town: %s</h3>
<h3>County: %s</h3>
<h3>Country: %s</h3>
<h3>Poscode: %s</h3>
<hr>
<h4>Telephone: %s</h4>
<h4>Email: %s</h4>
<hr>
<h4>URL: %s</h4>
<h4>Catalogue URL: %s</h4>
</div></div>';
while (($result = $statement->fetch(PDO::FETCH_ASSOC)) !== false) {
printf($formatString,
$result['pod_name'],
$result['pod_address'],
$result['town'],
$result['county'],
$result['country'],
$result['postcode'],
$result['telephone'],
$result['email'],
$result['url'],
$result['catalogue_url']
);
}
} else {
printf('<li class="tna-result">%s</li>', 'Please select a region from the map.');
}
Thanks
3 Answers
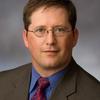
Ted Sumner
Courses Plus Student 17,967 PointsFirst, if you control the database you should remove them from the data. Then you don't have to worry. It doesn't look like they belong.
If you cannot remove them, I would try something like this (This is for the concept. The exact code below may or may not work):
<?php
foreach ($result as $data) {
if ($data ==='\n') {
$data = "";
}
}
This code will need some tweaking because you want to actually assign the array value to "" from "\n", but that is the idea of what I would do. You remove them from the result at the beginning and you no longer have to worry about it later in the code.
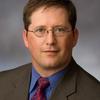
Ted Sumner
Courses Plus Student 17,967 PointsThinking a little more, you could have a counter and update the array based on the counter value. Then the array structure is altered directly.
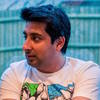
Punal Chotrani
8,817 PointsHi Ted, Thanks for the feedback. I was looking for a more simple method of using conditions in the the PDO. Something like this.
<?php if (in_array($data ==='\n')) { unset($result['']); }
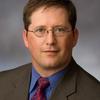
Ted Sumner
Courses Plus Student 17,967 PointsYes, that is probably a much better solution than what I thought of. There is so much about PHP I still have to learn. :)
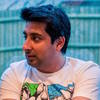
Punal Chotrani
8,817 PointsThis is probably the best way to learn. As soon as i find a solution i will post it up on this forum.
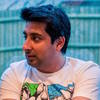
Punal Chotrani
8,817 PointsAt the end, this is the solution i went with
<?php if (isset($_GET['id'])) {
$sql = "SELECT * FROM pod_repo WHERE pod_id =:id";
$statement = $pdo->prepare($sql);
$pod_id = filter_input(INPUT_GET, 'id');
$statement->bindValue(':id', $pod_id, PDO::PARAM_STR);
$statement->execute();
$output = array();
while (($result = $statement->fetch(PDO::FETCH_ASSOC)) !== false) {
$output[] = '<span class="inline">Pod Name:</span> ';
$output[] = $result['pod_name'];
$output[] = '<br>';
$output[] = '<span class="inline">Pod Address:</span> ';
$output[] = $result['pod_address'];
$output[] = ', ';
$output[] = $result['town'];
$output[] = $result['county'];
$output[] = ', ';
$output[] = $result['country'];
$output[] = ', ';
$output[] = $result['postcode'];
$output[] = '<hr>';
$output[] = '<span class="inline">Telephone:</span> ';
$output[] = $result['telephone'];
$output[] = '<br>';
$output[] = '<span class="inline">Email:</span> ';
$output[] = '<a href="mainlto:"'.$result['email'].'>'.$result['email'].'</a>';
$output[] = '<br>';
$output[] = '<span class="inline">Url:</span> ';
$output[] = '<a href='.$result['url'].'>'.$result['url'].'</a>';
$output[] = '<br>';
$output[] = '<span class="inline">Catalogue Url:</span> ';
$output[] = '<a href='.$result['catalogue_url'].'>'.$result['catalogue_url'].'</a>';
}
$outputString = implode($output, ' ');
$properString = str_replace('\N', '', $outputString);
echo $properString;
}
} ?>
It's not perfect, but if anyone feels a better way of doing this, please let me know, and it has worked but not perfect.
Ted Sumner
Courses Plus Student 17,967 PointsTed Sumner
Courses Plus Student 17,967 Pointsedited to format code.